props and createMemo
Solid Start Cloudflare-pages deploy returns 404
How can I animate an svg element, that already has it's custom transform
Parent Get/Set Child Property
createEffect not re-runing when value in accessors are changed

Debugging "Error: Can't render headers after they are sent to the client."
setCookie
in my Supabase server client initialization function.
I'm not sure where to start on this one. I've been able to confirm that the problematic cookie is the Supabase Auth Token by logging out the name and the value when it errors: { name: 'sb-<project>-auth-token', value: '' }
...How does Solid attach event handlers to DOM elements?
Routes and fetch requests
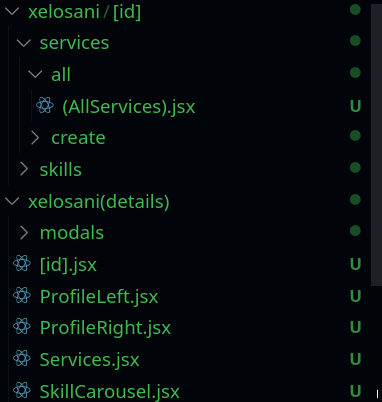
<Suspense> not fallbacking correctly
@solid-primitives/spring: obtain velocity?
router: "background" page
Having The Same Context Transfer Different Properties
Handling loading state
[valibot] Types generic
get
(then post
later).
I think you can guess what is the endpoint.
...useDraggable how to make second argument reactive
How to modify array partially?
Why does my component only update in a dummy For loop?
navigate - Wait for navigation to finish
useUser
and useMaybeUser
with the only difference between them being that useUser
would enforce that user is present or navigate you to login page.
Here's what I came up with for now.
```ts
import { useNavigate } from "@solidjs/router";...Another `template2 is not a function` issue
<h1>Hej</h1>
there is no template2
error.
I was originally using @kobalte/core's Tabs
component, then decided to try and make my own rough tab switch component. Both have produced this error and I'm not really sure how best to proceed or troubleshoot. So any guidance would be helpful....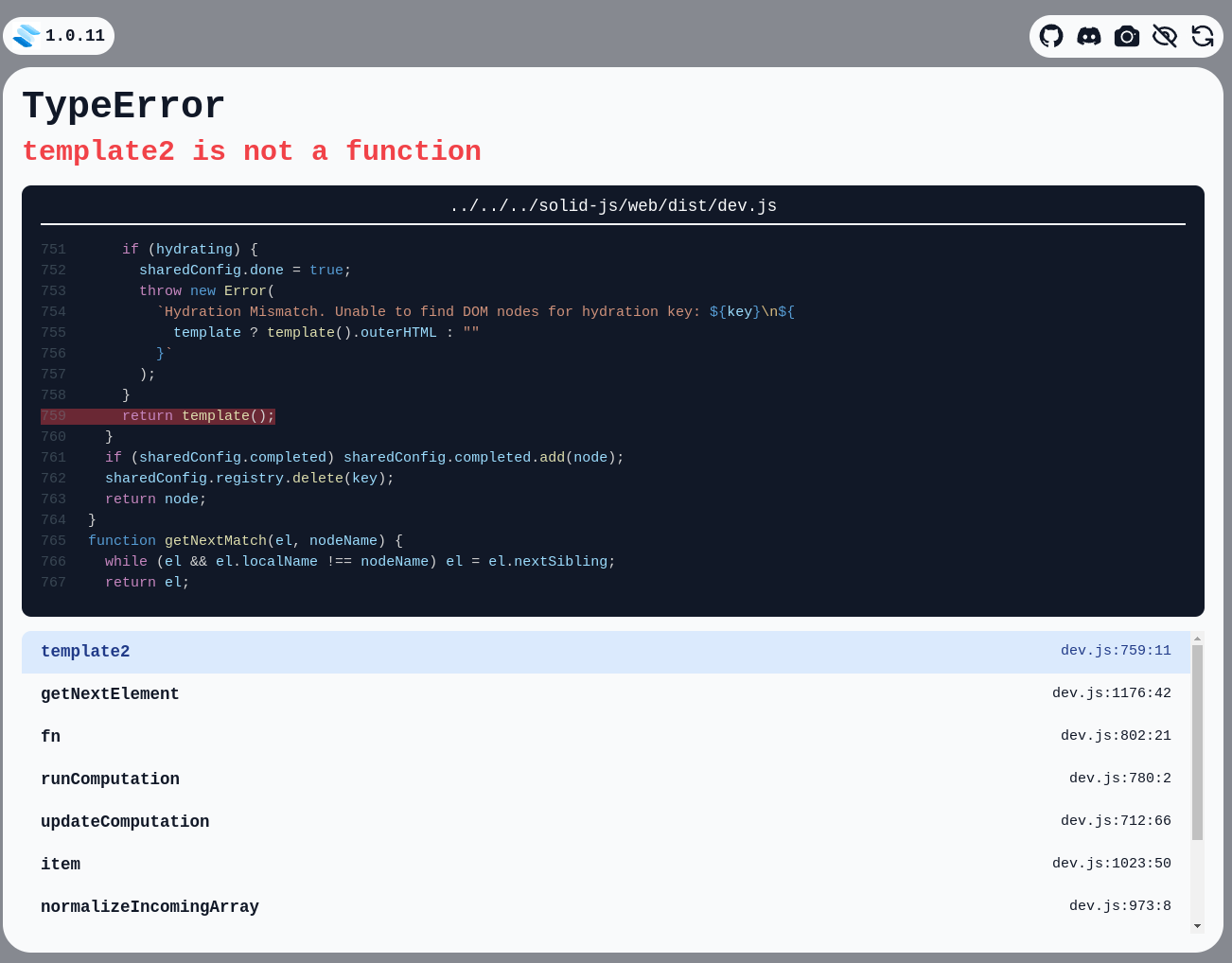
solid-router causes error: "cleanups created outside a `createRoot` or `render` will never be run"
createRoot
or render
will never be run
- computations created outside a createRoot
or render
will never be disposed
Since the router is actually rendered inside the render()
method of solid, I am not sure what I am doing wrong, any help how to fix the warnings would be appreciated. Below you can see my code (I also uploaded the full files because pasting entire code is higher than the discord message limit....