Best practices when interfacing with vanilla JS libraries.
I'm trying to integrate lexical editor with my Solid app. Can you tell me if I'm thinking these integraions correctly?
```
export const UserInput: Component<ColumnProps> = (props) => {
let editorInstance: LexicalEditor | undefined...
How to force refetch on createResource trigger with same fetcher id
createResource(() => props.id, fetch_calculation)
when props.id triggers twice with the same value like 'abc' and 'abc', createResource doesn't refetch.
I want it to refetch to get a fresh clone of the fetch_calculation result.
...Best LTS / Stable use of Solid for projects & business?
If I have a project I want to be viable and actually ship a product, would something like SolidStart actually be good enough?
Obviously it won't be as feature rich as NextJS and be more volatile, but will it suffice?
Are there other options?
I love Solid way more than React...
How to make controlled <textarea>
I'm trying to make the simplest possible controlled textarea. What I want is to show only and exactly what I set, not what the user types.
My problem is that this only works for one single character, after which the user can just type freely.
Minimal repro case:...
SolidStart Websocket Proxy
Hello,
I've tried to create a proxy to standalone websocket server in SolidStart, for purpose of this showcase I created a simple demo showing the issue I ran into.
index.js aka the websocket server...
"use server" file vs "use server" function
Hi all,
I just have a question about the behaviour of "use server" for a single function vs "use server" for a whole file..
I have a sign-up component that imports an action from my api:
```ts...
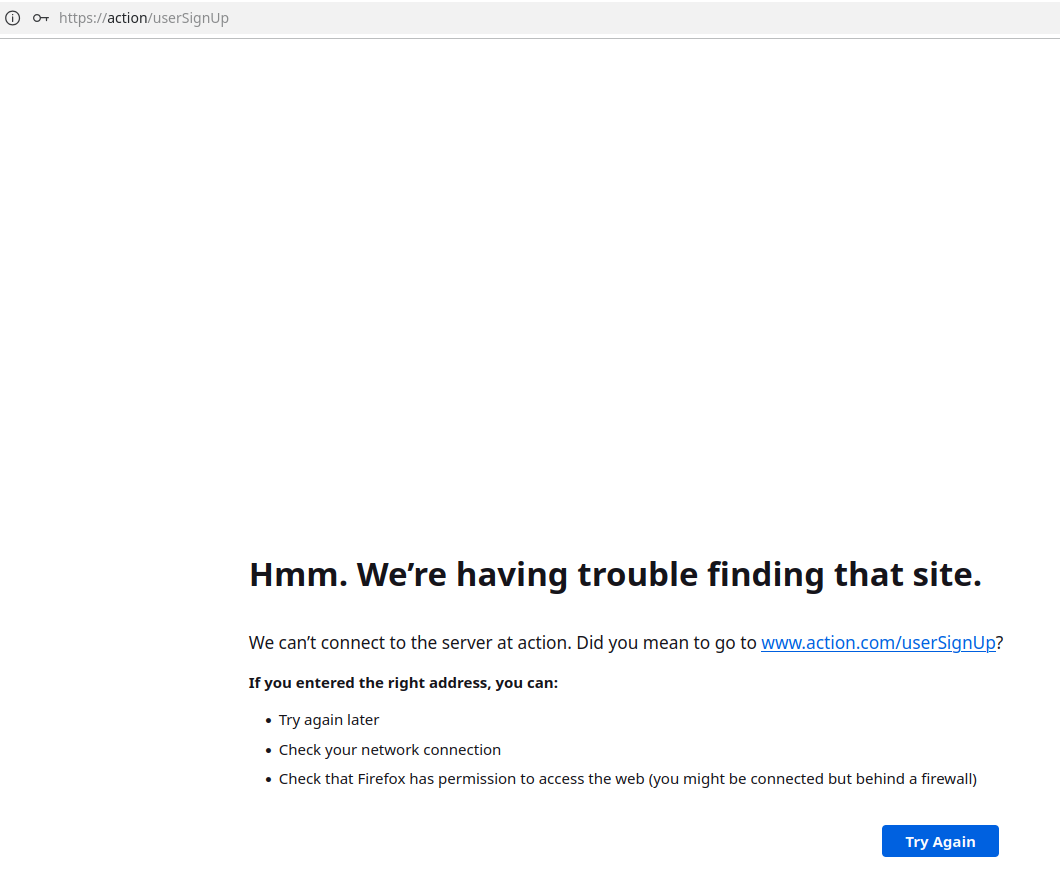
Auth guard
Hey there. I was trying to find a good solution to handle authentication and set up a guard that will redirect users to the authentication page if they are not authenticated.
While reading this discussion (https://github.com/solidjs/solid-router/discussions/364), I couldn't help but notice this comment
"This has the 2nd most upvotes of all time with no response"
...
Navigation error
I get a weird
cleanNode()
exception when navigating to another page.
The stack trace is useless (see in separate comment).
The navigation is performed by a <button>, it is trivial, nothing extra happens there: <button onClick={() => navigate(...)} ...
Do you have any recommendation how to find the root of the error?
Thanks....dynamic routes 404?
how can I intentionally throw 404 on a dynamic route (no server action or anything)?
Infinite scroll
I want to make infinite scrolling on notifications page since this is notifications page I don't use SSR, What I have problems with is how observer sees the last element of array. When I have elements enough to show scrollbar it doesn't have problems since it doesn't intersect with last element so my fetch request doesn't run twice but if I have small amounts of elements it sees the last element so it makes another request
here is the code:
`
createEffect(() => {...
Ignore specific error on the client during development (DevOverlay)
I'm getting an error about ResizeObserver that seems unavoidable.
https://github.com/inokawa/virtua?tab=readme-ov-file#what-is-resizeobserver-loop-completed-with-undelivered-notifications-error
I had the
SSR
enabled and I could avoid it by the following code:
...
Error when evaluating SSR module $vinxi/handler/ssr
Hi everyone
After update my dependencies, I'm facing this error
Error when evaluating SSR module $vinxi/handler/ssr:
These are my dependencies:
```json
"dependencies": {...
cloudflare sending 200 status for *404
pnpm create solid
choose solidstart
set cloudflare-pages preset
wrangler pages deploy dist (don't do wrangler pages dev, it works fine on local)
go to any page that doesn't exist on your production url, you'll see 200 status...
Updating a signal vs Updating the data in the database
I have a model created by various signals that is local to the browser. I also use indexedDB solid-dexie to persist some data. Now when I make changes to my model I want parts of changes to be persisted to the database as well. I said I am using solid-dexie, but what are some libraries or best practices to handle persistence of the application?
My use case is local only preferrably IndexedDB on the browser as the main database....
I want to debounce a signal for some effects, but not others
In short, I'm using a slider to allow the user to quickly adjust font scale linearly. I need the font to respond to the 1000s of adjustments, but I do not want to update the history buffer with every minor font change that the slider will trigger.
I tried to get the primitives to work, but failed to find a way to have both instant and bounced effects without having to write a lot of code.
https://primitives.solidjs.community/package/scheduled#debounce
...
When, and what types of errors <ErrorBoundary> catch?
The docs are not clear about exactly what types of errors are caught by
<ErrorBoundary>
. My understanding is that:
<ErrorBoundary>
catches any error that occurs during rendering or updating its children. That includes:
- Errors that occur while rendering the JSX....Google Analytics
Hi everyone
I'm trying to add Google Analytics to my Solid Start project, but no data appears on the analytics page.
Already try two ways:
- Using script loader...
props and createMemo
I'm confused. I thought I understood perfectly Solid, and today I stumbled upon a performance issue that I don't understand.
I've created a very small and silly example to reproduce it.
https://playground.solidjs.com/anonymous/21929a27-2909-461b-87fe-141c7401f23c
...