Muhct
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Muhct on 11/10/2024 in #questions
Recommended code analysis tool for React?
I'm interested in adding a code analysis tool for my React-Typescript project to check what can I improve. I already have ESLint and Prettier.
Perhaps I could use SonarCloud or Snyk, but my issue is that:
1) The things SonarCloud detects when it does code analysis are not advanced enough for me to think that it's worth it. It detects code duplication, possible undefineds, etc. That's fine, but I'm looking for a tool that is more advanced and detects less obvious stuff.
2) My app has no interaction at all with any backend. There isn't any HTTP requests, which makes it less vulnerable from the get-go. And I have good results running npm run audit for the packages. So a security-focused tool like Snyk is also not worth it for me.
Is there something else you would recommend?
2 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 9/14/2024 in #questions
Is there a Vitest UI equivalent for Jest test suites?
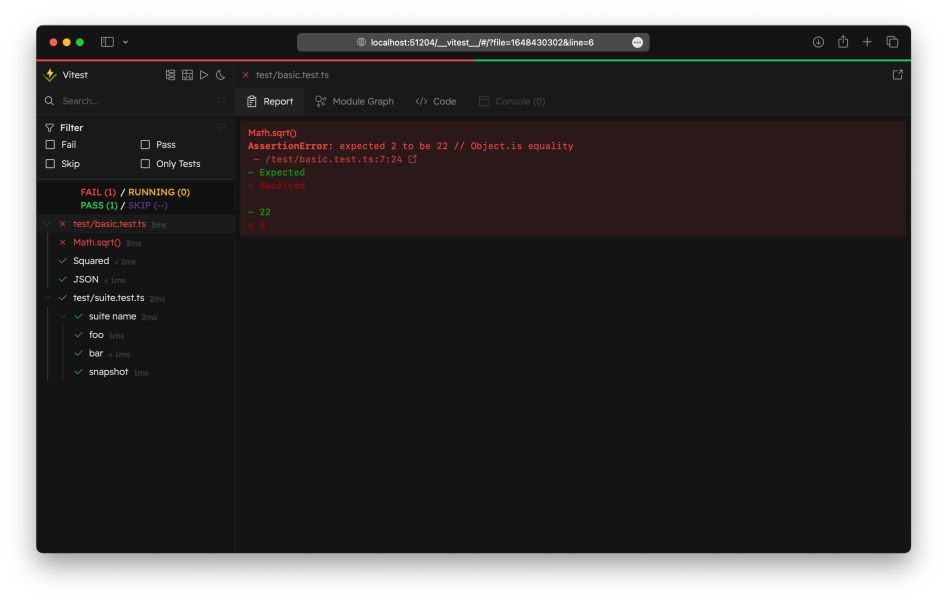
2 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 8/17/2024 in #questions
Is there a built-in way to prevent ID duplicates using Zod?
Let's say that your data is an array of objects with "id" and "name" fields:
as you can see, the id is duplicated but it should be unique. Does Zod have a built-in method to validate against this kind of issue? I wasn't able to find any. Or am I forced to use .refine and create a new Set with all the ids in my data?
5 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 7/14/2024 in #questions
Using hardcoded data for comparison in unit tests with toEqual?
Hi there, I have a React app and I want to create a unit test for a function that removes scattered zeros inside of an array. Basically my idea is to have a src\mocks\data\IndexadorDataMock.ts file with hardcoded dirty data, and then the (also hardcoded) clean data - aka the expected output. For example:
And then my test file Helpers.test.ts:
My question is: is this a common practice for React projects? By "this" I mean having a separate file with very long, hardcoded data that you'll use for .toEqual comparisons in your tests
8 replies
KPCKevin Powell - Community
•Created by Muhct on 7/11/2024 in #front-end
Px, em, or rem?
Hi there - I haven't decided which unit I should use... Do you recommend px, em, or rem? I've read several articles about this topic but they just go over my head... Basically my question is: why shouldn't I use em for absolutely everything?
15 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 6/22/2024 in #questions
Best practices for rendering the version of your app
I have a React-TypeScript web app in which I'd like to render the current version at the top of the home page. What I'm thinking is that I could have a version.ts file with this: and then when I make a commit, I increase that number. But I've never seen this being done in real world open source github projects. What's the best approach here?
14 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 6/20/2024 in #questions
Best practices for typing a reusable button?
I have a React-TypeScript-Material UI app with a Button component but I'm not sure if what I'm doing is a good practice. Basically in the ButtonProps 4 out of 5 props are optional. Is this ok or should I structure the code in a different way? Perhaps not turning the button into a reusable component at all?
6 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 11/3/2023 in #questions
Improving accessibility of a Card component
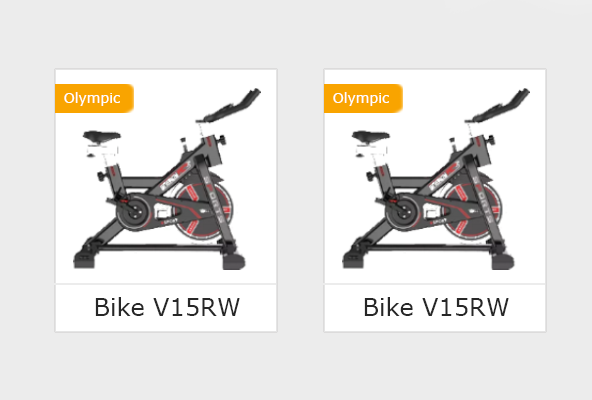
2 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 6/29/2023 in #questions
Sizes prop in the <Image/> component
I've always been using the <Image> component with the fill prop, but I've never used the sizes one: https://nextjs.org/docs/pages/api-reference/components/image#sizes I'm reading the docs and I don't understand what it does. I thought NextJS Images were automatically served with the proper size according to the device of the user, was I wrong? Is the sizes prop the thing that takes care of that? As an example, I have a hero image that always takes up the whole screen width, I've added the sizes prop like this: and I don't see any difference when it comes to the srcset of images when I inspect the element - or any difference in lighthouse (with or without the sizes prop, the amount of KB spent on images is the same) so I'm not sure what it's actually doing
5 replies
KPCKevin Powell - Community
•Created by Muhct on 5/11/2023 in #front-end
Customizing Dev tools inspect element popup with element data?
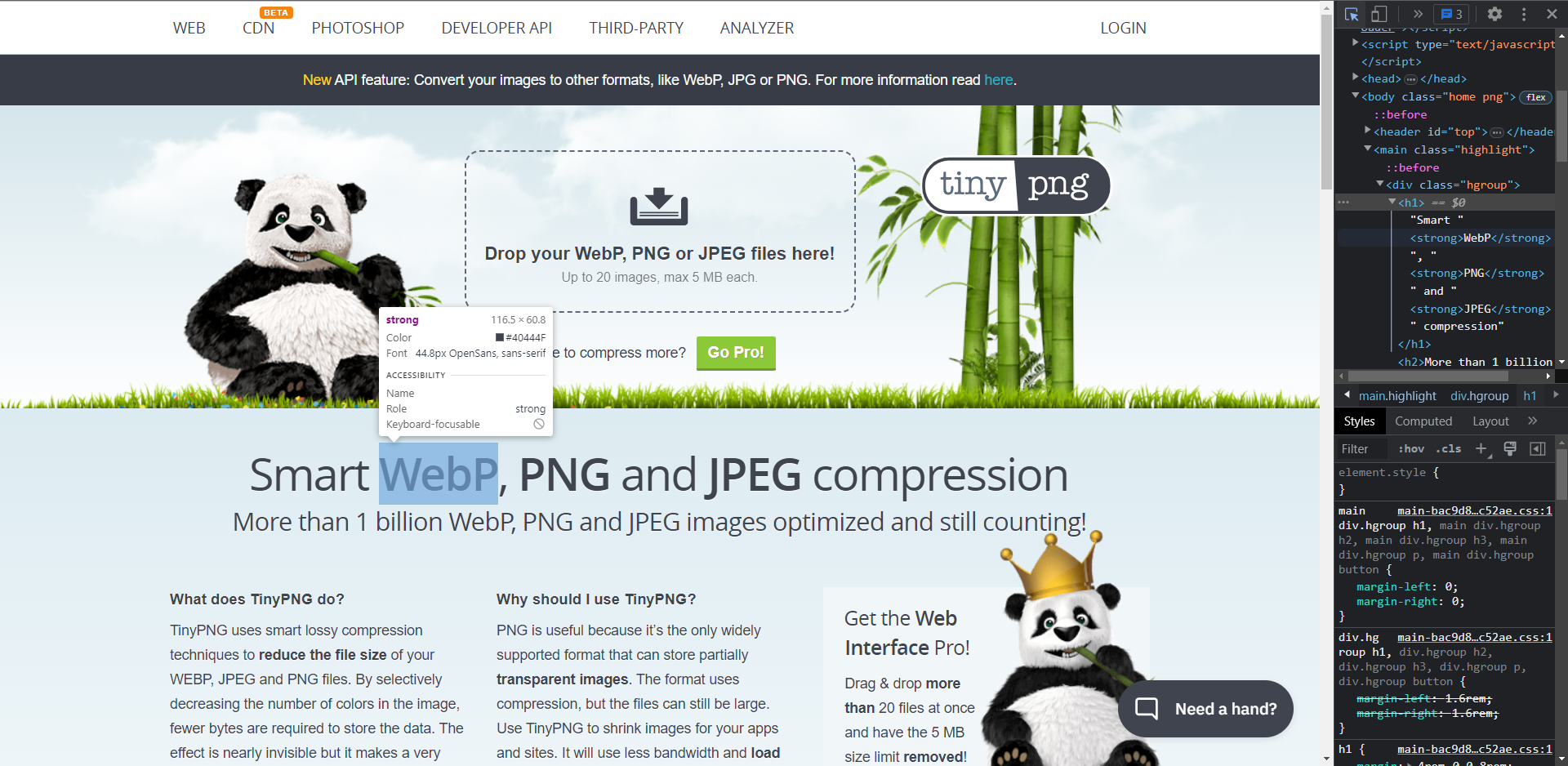
3 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 3/3/2023 in #questions
Serverless and price of DDoS attacks
There's one thing in serverless that I'm not able to figure out: as far as I know if you suffer a DDoS attack in AWS then you have to pay for all the usage that the DDoS attack has caused, which can get very pricey. Other non-serverless hosting companies dont have this issue: if you suffer a DDoS attack you dont get charged an extra amount.
Does that simply not exist in serverless? How do you tackle DDoS attacks for your apps in serverless? A rate limiter wouldnt be enough, right?
3 replies
KPCKevin Powell - Community
•Created by Muhct on 2/8/2023 in #front-end
Automated styling testing?
Hi there, I was wondering what's the common practice when it comes to testing the style of your whole website. What I mean is, there are libraries like react-library-testing/cypress that can carry out multiple tests in order to check if something on a different part of the website was broken in the process, checks if a button no longer exists or doesn't open a popup, etc. Is it possible to do that with styles? I'm thinking maybe a Puppeteer script that takes screenshots of all the pages in your website and tells you what parts started having a different size/font-weight/visual position, etc.
4 replies
KPCKevin Powell - Community
•Created by Muhct on 1/19/2023 in #front-end
Is there a way to properly check how your website looks on different devices?
I've seen that some components in my website look differently when using a Samsung A11 VS Xiami Mi Note Pro 10 for example. But I had to physically check this to know it, since on Firefox/Chrome responsive view it looks fine, so I was wondering if there's a (ideally free) way to properly check how your website looks on different devices. Like, truly simulation software?
54 replies
KPCKevin Powell - Community
•Created by Muhct on 1/12/2023 in #front-end
Is chrome dev tools responsive view working correctly?
Hi there, since 14 days ago I think, when I use the chrome dev tools to see if my website is responsive and move around the responsive bar to increase/decrease the width of the viewport, I can see that my website goes out of the viewport and I can scroll horizontally. Now, if I close the responsive view and then close the dev tools, and then open it up again, it looks ok. Am I the only one?
6 replies
KPCKevin Powell - Community
•Created by Muhct on 1/10/2023 in #front-end
Removing the built-in padding-margin of SVGs
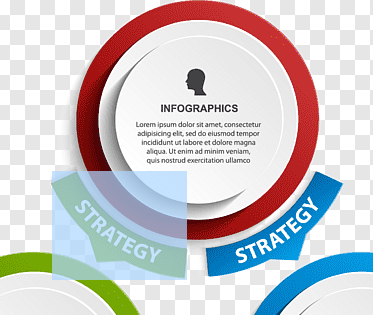
12 replies
KPCKevin Powell - Community
•Created by Muhct on 1/9/2023 in #front-end
Transform translate(X, Y) differs in Mozilla & Chrome
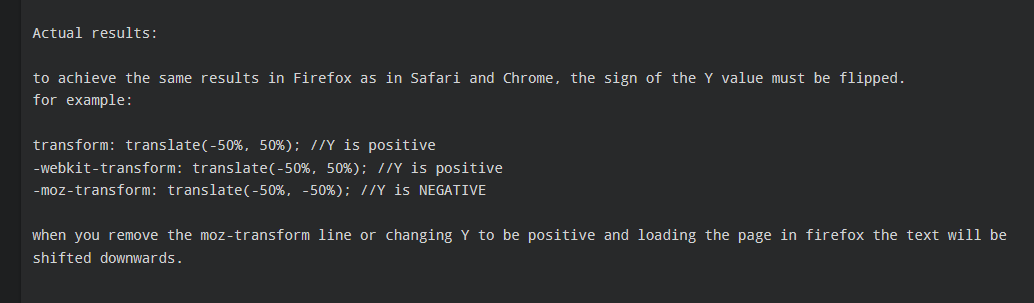
9 replies
TTCTheo's Typesafe Cult
•Created by Muhct on 12/26/2022 in #questions
Prevent DesktopHeader from loading on network tab
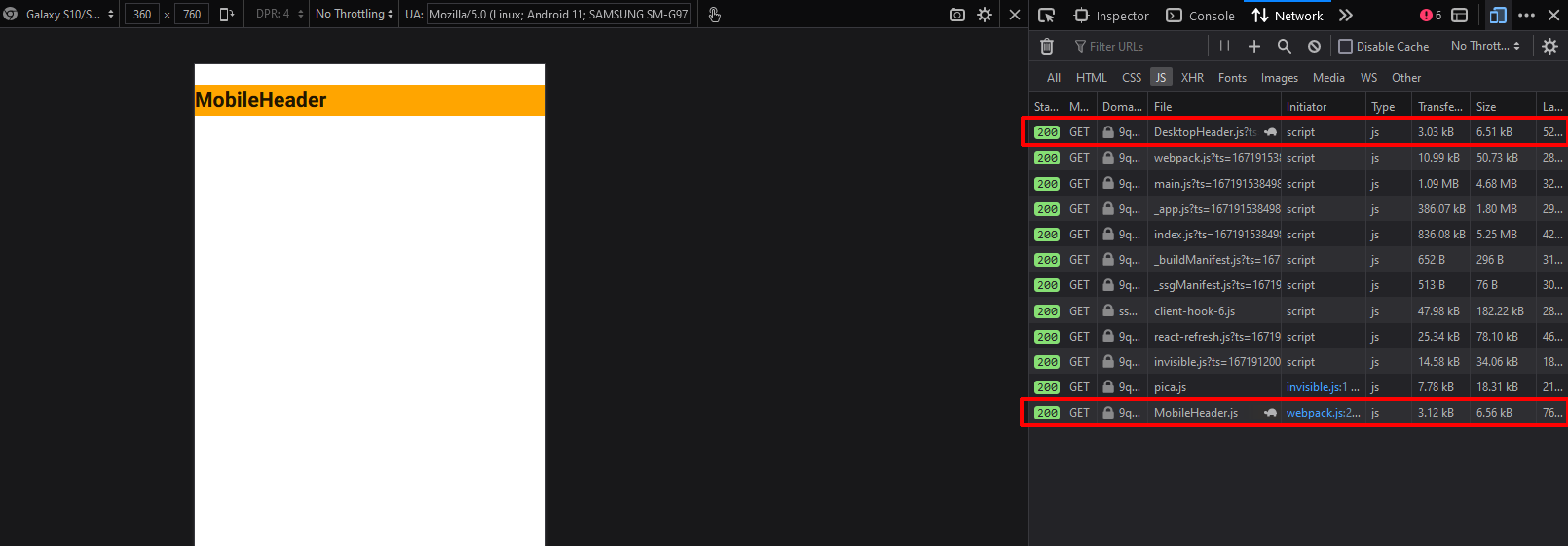
1 replies
KPCKevin Powell - Community
•Created by Muhct on 12/25/2022 in #front-end
Add a circle to a CircleProgress component
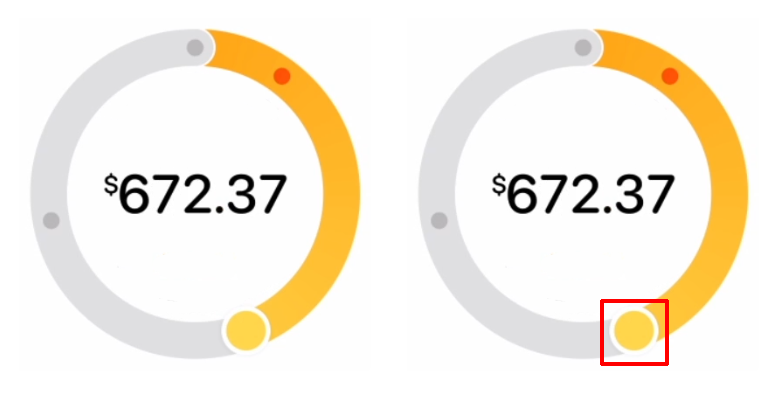
5 replies
KPCKevin Powell - Community
•Created by Muhct on 12/14/2022 in #front-end
CSS Color filter calculator
Hi there, does someone know of a CSS color filter calculator that lets you select the starting color and the objective color? I've found 4 of these calculators but the issue is that you can't set the starting color, only the final color
2 replies
KPCKevin Powell - Community
•Created by Muhct on 12/12/2022 in #front-end
Creating a 2-column 2-row flexbox structure
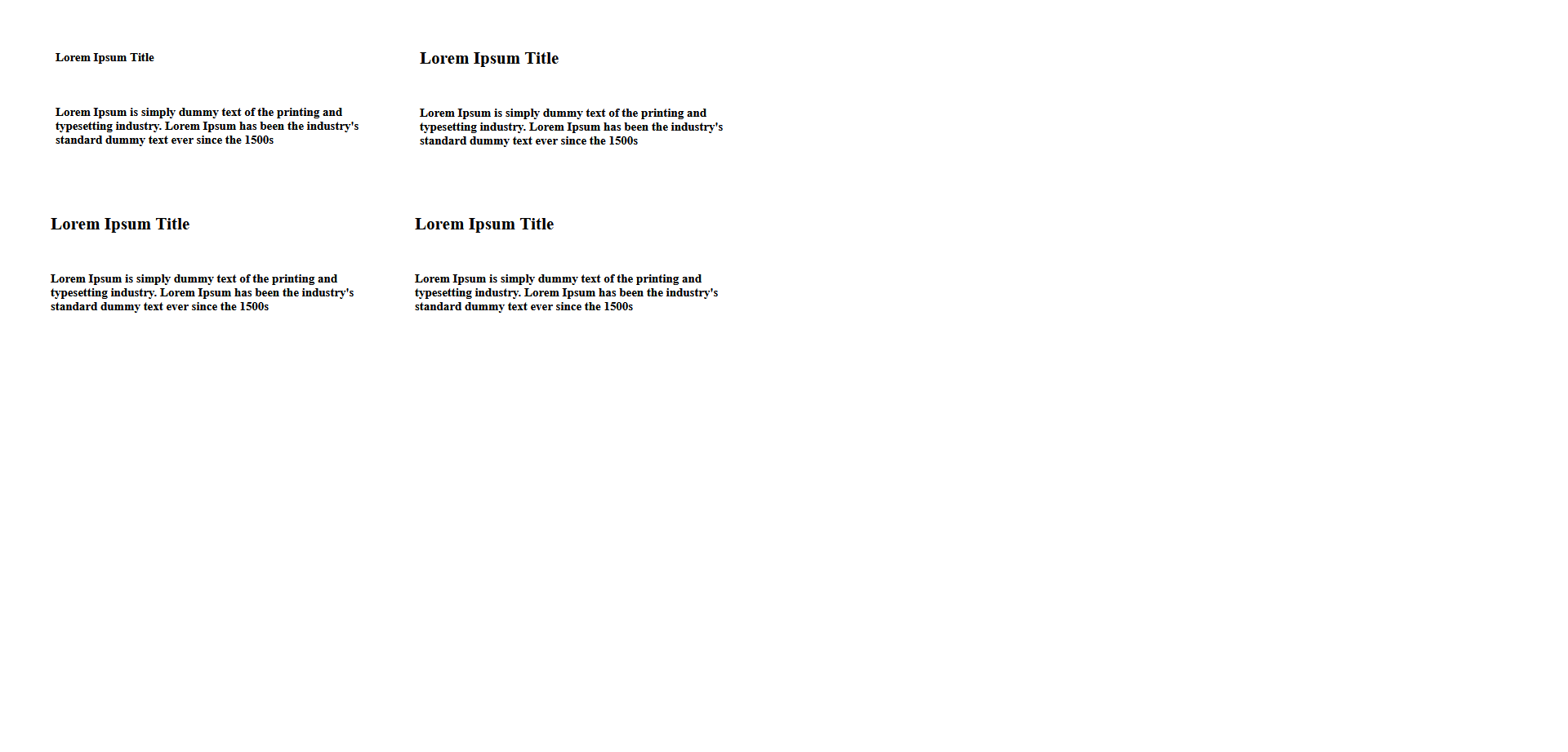
6 replies