jdgamble555
Explore posts from serversCDCloudflare Developers
•Created by jdgamble555 on 4/20/2025 in #pages-help
Running SvelteKit in Cloudflare
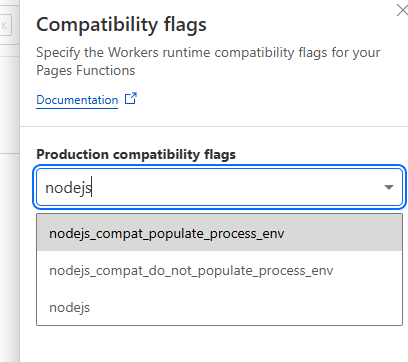
3 replies
When to use createEffect
I have a signal that depends on another signal. My
todos
signal is depended on my user
signal. When there is a user, I want to subscribe to the todos collection, and when there isn't I want to unsubscribe. When there is again (login), I want to resubscribe (user could change too). Should I use createEffect
for this? I thought you shouldn't set a signal inside a signal though? Here is my code:
Thanks,
J6 replies
Preloading Data with 'use server'
I'm following the limited documentation, and I want to preload data from the server for a route:
I am preloading some data from a database. I'm not sure why the
createAsync
has an option for undefined, but I do about()
as the docs suggest. However, the data does not display properly UNLESS I do full referesh. It does not stay when navigating back to the page. I only want to fetch it once, I just don't every want a blank page.
You can see the example (without logging in) :
https://solid-start-firebase.vercel.app/
How do I fix this?
J15 replies
Client inside Server Component
Is it possible to put a client component inside a server component? I can't get this to work. I also tried with
experimental.componentIslands.selectiveClient
and using nuxt-client
. Any examples of this, or does it not work.
Thanks
J4 replies
CDCloudflare Developers
•Created by jdgamble555 on 3/14/2023 in #workers-help
Connect to Redis on Cloudflare Workers
I'm getting this error when trying to connect to a simple redis instance:
How can I simply connect to Redis on my Cloudflare instance? My code is simple:
https://github.com/jdgamble555/redisgraph-graphql-sveltekit/blob/master/src/lib/server/redis.ts
How can I get this to work?
J
5 replies
CDCloudflare Developers
•Created by jdgamble555 on 1/23/2023 in #workers-help
SvelteKit Deployment Not Working
I was able to deploy my SvelteKit Test App fine when I used the Wrangler CLI.
https://cloudflare-svelte.jdgamble555.workers.dev/
https://github.com/jdgamble555/cloudflare-svelte
However, I want to tie my deployments to GitHub Actions instead of using Wrangler. I deployed the exact same app in the dashboard under Pages --> Create Project --> Connect to Git (selecting my project with the SvelteKit Template) and I get the following error:
How can I get this to work through GitHub actions?
J
11 replies