Preloading Data with 'use server'
I'm following the limited documentation, and I want to preload data from the server for a route:
I am preloading some data from a database. I'm not sure why the
createAsync
has an option for undefined, but I do about()
as the docs suggest. However, the data does not display properly UNLESS I do full referesh. It does not stay when navigating back to the page. I only want to fetch it once, I just don't every want a blank page.
You can see the example (without logging in) :
https://solid-start-firebase.vercel.app/
How do I fix this?
J11 Replies
I'm not sure why the createAsync has an option for undefined
Unless you supply it with an initialValue
it's undefined
before the async op settles. I view createAsync
as the boundary between async values/events and the synchronous reactivity of Solid.
Reactive values are continuous so they will start out as undefined
unless they are given a specific value to start with.
optionsGitHub
solid-router/src/data/createAsync.ts at 1d0d4ffc5115962e41fce730f0c...
A universal router for Solid inspired by Ember and React Router - solidjs/solid-router
This fixes the problem
The TSX area is also an effect.
So
about() && tsxEffect
never let the TSX effect register during client side component creation when about()
was still undefined
.
When refreshing, SSR + Suspense saved the day because it caught the promise and only (re?)created the component once the promise resolved.
You have to let go of these "React-isms" which include using <For>
instead of .map()
otherwise things just won't work or de-optimize.first of all, the whole
about() && (...)
wouldn't work since it's a static expression, and as you know Solid components only evaluate once.
You need to use Show
for guaranteeing the value and Suspense
to allow the SSR to wait for the dataSo this solves the problem where it doesn't load at all, but it still clearly jumps when you first load the page: - https://solid-start-firebase.vercel.app/about
I just want it to load from the server after the data has been fetched. It shoud NEVER have the option to display a blank page. This is what it SHOULD look like - https://nextjs-firebase-todo.vercel.app/about
Create Next App
Generated by create next app
I just want it to load from the server after the data has been fetched.When does Next.js fetch that data? Before or after the navigation?
I want it to load only on the server before the page is displayed... it would have to be after the navigation (as it wouldn't know what to fetch otherwise), but before the page load
If you click on the link - https://solid-start-firebase.vercel.app/about - and click refresh, you see it jump... is this just how Solid works?
If you are talking about the SSR behaviour try:
Yup that fixed it. What does that do exactly?
This is what it SHOULD look likeBy default it starts the response ASAP to show something immediately and it then streams the resolved promise to update the page (progressive rendering).
deferStream
indicates to not release the response (suspense boundary?) until that async op has settled.https://dev.to/ryansolid/server-rendering-in-javascript-optimizing-performance-1jnk
https://dev.to/this-is-learning/marko-for-sites-solid-for-apps-2c7d
DEV Community
Server Rendering in JavaScript: Optimizing Performance
If you read Server Rendering in JavaScript: Optimizing for Size you might be wondering what else is...
DEV Community
Marko for Sites, Solid for Apps
I've been sitting on writing this article for 2 years. In my heart this was the article I was going...
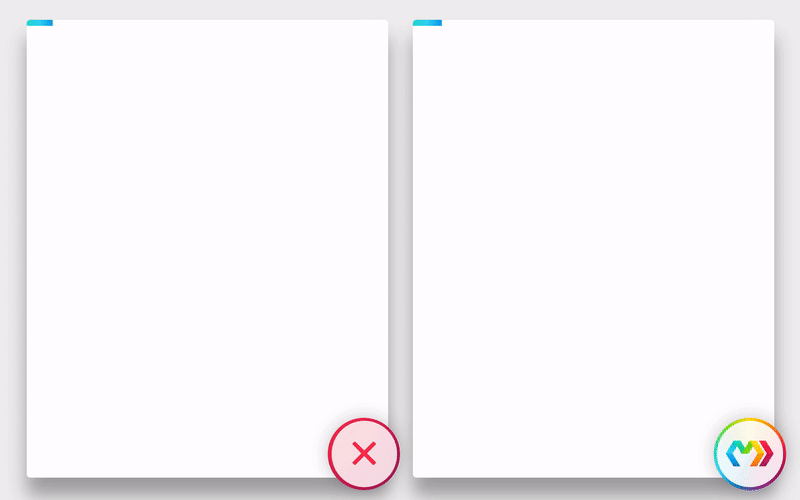
ok got it thanks! so it does work without it, just with streaming... makes sense