[RS] JesseUCAVedYou [EN]
Explore posts from serversDIdiscord.js - Imagine ❄
•Created by [RS] JesseUCAVedYou [EN] on 10/8/2024 in #djs-questions
Keeping A Channel Polls Only
I'm trying to make a specific channel polls only. Since Discord doesn't have a permission set for this I've setup my bot to detect the difference in a poll message and a regular message.
Regular messages get deleted from the channel and the user a DM stating why.
Polls get ignored.
This has been successful.
What isn't successful though is when the poll ends and Discord sends out the notification message stating the poll has ended it gets deleted as well.
Is there a way to detect poll end messages too? I tried having the bot see if the messages contained specific text, but no luck.
14 replies
DIdiscord.js - Imagine ❄
•Created by [RS] JesseUCAVedYou [EN] on 10/6/2024 in #djs-questions
Bot Not Updating Voice Permissions
I'm trying to have an event voice channel that is locked(
everyone
cannot connect or speak), but when an event is live have the perms update so everyone
can connect and speak to that channel.
There are no console errors I am seeing, and I know it's detecting an active event since the channels status gets updated to reflect the event name as it's supposed to.
Can someone give this a look over and see what I'm doing wrong in the permissions area?
3 replies
BBattleMetrics
•Created by [RS] JesseUCAVedYou [EN] on 8/10/2024 in #support-forum
7 Days to Die - Player Leaves
It seems that with this last 7 Days to Die update triggers no longer fire when users leave the server.
Guessing a variable or something got changed on their end. 🙂
Just figured I'd let you know.
2 replies
BBattleMetrics
•Created by [RS] JesseUCAVedYou [EN] on 8/7/2024 in #support-forum
7 Days to Die - Joining Trigger - Triggers Twice
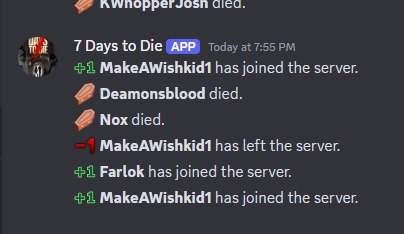
10 replies
BBattleMetrics
•Created by [RS] JesseUCAVedYou [EN] on 7/25/2024 in #support-forum
7 Days to Die Chats
Chat messages from 7 Days to Die don't seem to work anymore.
On the website console you can't see what players say anymore and when using this code in a webhook no chats get sent to Discord.
This same exact code I use for sending Battlebit Remastered chats to a webhook. Both games support the same variables so I don't believe it's the code.
7 replies
BBattleMetrics
•Created by [RS] JesseUCAVedYou [EN] on 5/16/2024 in #support-forum
Few variables not working - Battlebit
Tried to create a message for a command in game. Variables weren't working so I added all the
squad
ones to a console log and got this as the result: (Activity Log) JesseUCAVedYou used the !lead command and became squad leader of {{player.squadName}}. User ID {{user.id}} User Nickname {{user.nickname}} Team Index {{player.teamIndex}} BattleBit Remastered Squad Index {{player.squadIndex}} BattleBit Remastered Squad Name {{player.squadName}} BattleBit Remastered Is Squad Leader true (5) (Trigger)
.12 replies