Keeping A Channel Polls Only
I'm trying to make a specific channel polls only. Since Discord doesn't have a permission set for this I've setup my bot to detect the difference in a poll message and a regular message.
Regular messages get deleted from the channel and the user a DM stating why.
Polls get ignored.
This has been successful.
What isn't successful though is when the poll ends and Discord sends out the notification message stating the poll has ended it gets deleted as well.
Is there a way to detect poll end messages too? I tried having the bot see if the messages contained specific text, but no luck.
10 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!-_- and my question/code gets removed. le sigh
not sure what you mean by that, it's in the post starter where it should be?
Check also for the message type
Ignore if it is of that type ^
Discord must be messing up on my end then :/
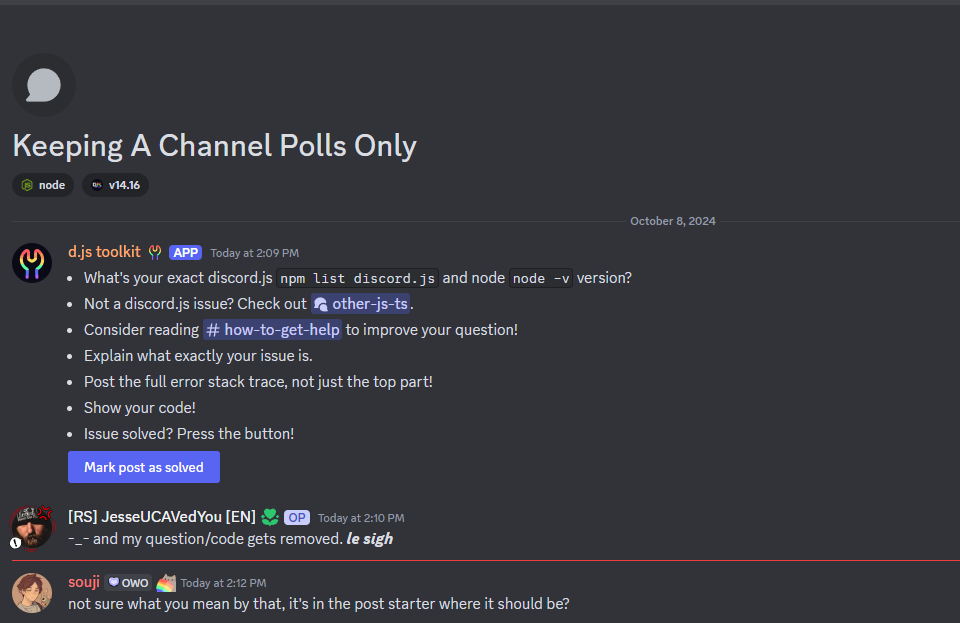
So here I am looking like a fool lol
interesting :kek:
it's def there
:PES_Happy:
Will try. Thanks!
Works wonderfully thanks to you. Will post my code here for future reference of others.