hapless.dev
Explore posts from servers❔ error: Unable to create a 'DbContext' of type '' ". When adding migration to MySQL database
hi there!
im just starting a webapi project and i wanted to use a mysql docker container and go code-first using the entity framework, however, im having troubles trying to add migrations, im getting the following error
in theory, i have my dbcontext declared
and my program builds with it
could someone give me a hand? what am i missing?
2 replies
DIAdiscord.js - Imagine an app
•Created by hapless.dev on 6/21/2023 in #djs-voice
how to set and track audio player states?
hi there, im learning data structures and algorithms and i thought it would be a nice exercise using them in a discord bot that takes a local folder and creates a track instance and plays it according to an initialized DSA... the thing is, i need to keep track of the player status, but it seems that
if (player.state === AudioPlayerStatus.__)
have no overlap and it complains, ive seen some player.on(___, async () =>)
implementations but looks confusing and verbose, is there any way to properly track and set player states to ensure the behaviour of a queue, stack or whatever?
21 replies
DIAdiscord.js - Imagine an app
•Created by hapless.dev on 6/18/2023 in #djs-voice
bot joins to voice channel, doesn't play any sound, times out and disconnects
hi there! im following the basic example repo, although is a common error, i wonder, since i basically have the same code as the repo
11 replies
DIAdiscord.js - Imagine an app
•Created by hapless.dev on 6/16/2023 in #djs-voice
another bot not joining the voice channel
Hi there, im learning my way through the docs and making my first bot, i was following line by line the basic example repo from
discord/voice
(https://github.com/discordjs/voice-examples/blob/main/basic/src/main.ts) but its not working, its basically the same code minus the formatting and the difference in current Status
comparison since it seems the deesctructuring of Constants
for Events, Status
is not longer used... the code logs is ready to play but when i type -join
on the chat it doesnt respond at all, is it because of the intents?
these are my dependencies
8 replies
DIAdiscord.js - Imagine an app
•Created by hapless.dev on 6/16/2023 in #djs-questions
good practices for project structure for a discord bot?
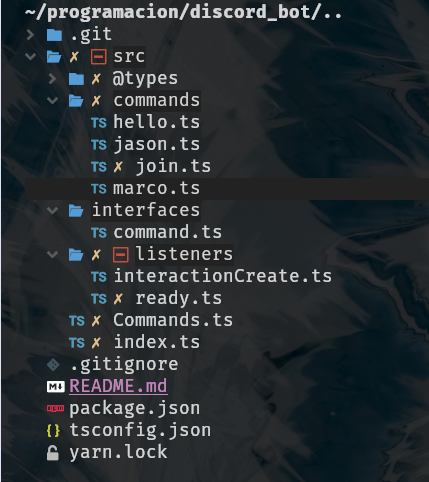
6 replies