bot joins to voice channel, doesn't play any sound, times out and disconnects
hi there! im following the basic example repo, although is a common error, i wonder, since i basically have the same code as the repo
6 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.
yes, all this is the
index.ts
and i just followed the repo which abstracts the VoiceAdapter
to the function in adapter.ts
in the repo, let me try it out!GitHub
voice-examples/basic/src/main.ts at main · discordjs/voice-examples
A collection of examples of how to use @discordjs/voice in your projects - voice-examples/basic/src/main.ts at main · discordjs/voice-examples
i plan to refactor it but when i've used my own
ready.ts
to instatiate the client and parse my commands but when i call the playSong()
, player
instance and adapter
that the repo calls on index.ts
the functions never get triggered because they dont log anything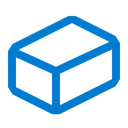
let me try it brb
weird right? i saw the discordjs.guide and all, and copied the code by hand, reading the types and interfaces but couldnt get why it did it like that instead of using the normal creator, its a shame that actual
.ts
implementations are so scarce for the discord api
huh, it worked with the normal adapter
10 months is old? haha wow, i thought it was kinda recent, this api and its ecosystem go fast af gotta admit