✅ controller endpoint gets 404 when hit
hi everyone, im trying to make an auth web api, i managed to create an AuthService and whatnot, and my controller has only two routes, im trying to implement the /register and swagger gets it fine, but as soon as i execute the request i get 404d, why is that? i tried curl and still, could someone give me a hand?
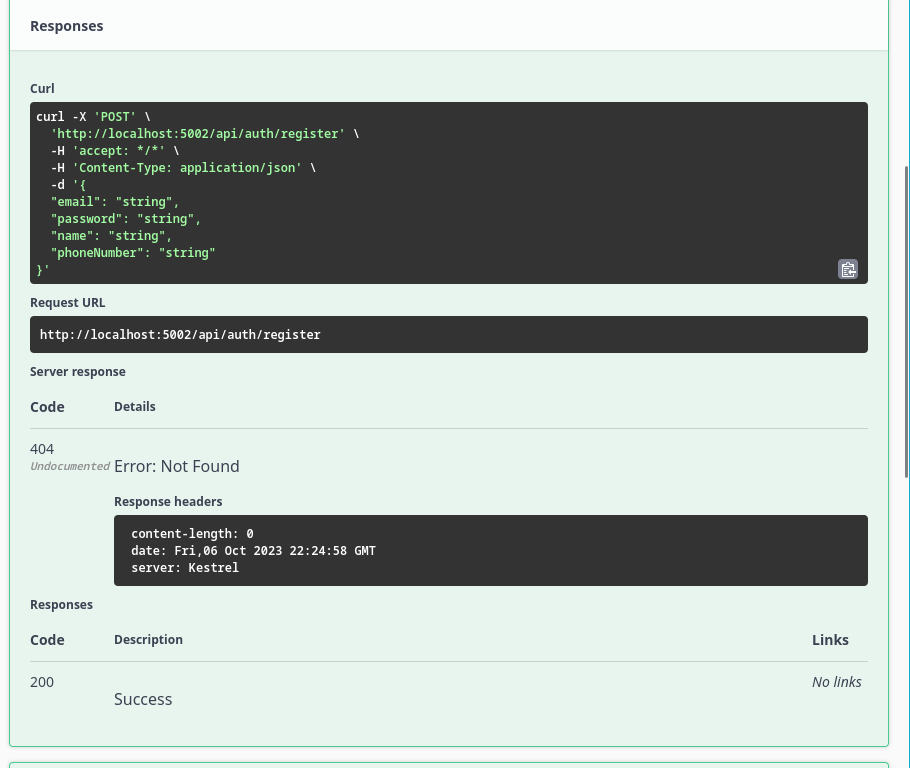
10 Replies
404 means the route you specified doesn't exist/isn't mapped
where is
AuthAPIController
registered?you mean like initialized? sorry im new to .net and c# in general, i think
builder.Services.AddControllers()
should parse any controller that inherits from BaseController
, or am i mistaken?
this is how my program.cs
looks like
i think builder.Services.AddControllers() should parse any controller that inherits from BaseControllerit should, yes you're missing routing and endpoints
could you provide the whole model of RegistrationRequestDTO
thank you so much!!! now it worked! however, i only added
when i tried to use
app.UseEndpoints()
the lsp would complain saying
There is no argument given that corresponds to the required parameter 'configure' of 'EndpointRoutingApplicationBuilderExtensions.UseEndpoints(IApplicationBuilder, Action<IEndpointRouteBuilder>)
im using .net 8.0, i was curious on the message and when would i need to use it or any sort of example where id encounter that, what does that error mean and why did it work without it?.MapControllers()
effectively has a call to .UseEnpoints()
inside of it, then
what .UseEndpoints()
is supposed to be is....
something to that effect
I.E. it's the method by which you register all of the endpoints you want your API to respond to
.MapControllers()
does that for you, but by reflecting upon all of your registered controllersi see, so and id have to map them to the specific routes on my controller i see right? like, for a more granular control over them?
yeah
I.E. if you're not using controllers
I'm a little rusty on the specifics
aha, i see
not at all, from this point i can take a look by myself, thank you so much! i was scratching my head so much around this
i really really appreciate it, thank you for your quick response, patience and guidance!
have a great day mate!