piscopancer
Explore posts from serversPPrisma
•Created by piscopancer on 11/17/2024 in #help-and-questions
Infer type from create query?
here's the function that creates a new
Schedule
. Attention to the shape, not data
I want to get the type of the Lesson
of the shape in this function. so it has to look like this
so that I can use somewhere else
Is there a utility type in prisma to infer this shape?7 replies
PPrisma
•Created by piscopancer on 11/15/2024 in #help-and-questions
narrow down String type using prisma client's extension?
can I explain to prisma client and typescript that this particular field needs to be a string union?
4 replies
PPrisma
•Created by piscopancer on 11/15/2024 in #help-and-questions
`Prisma.skip` seen as `undefined`?
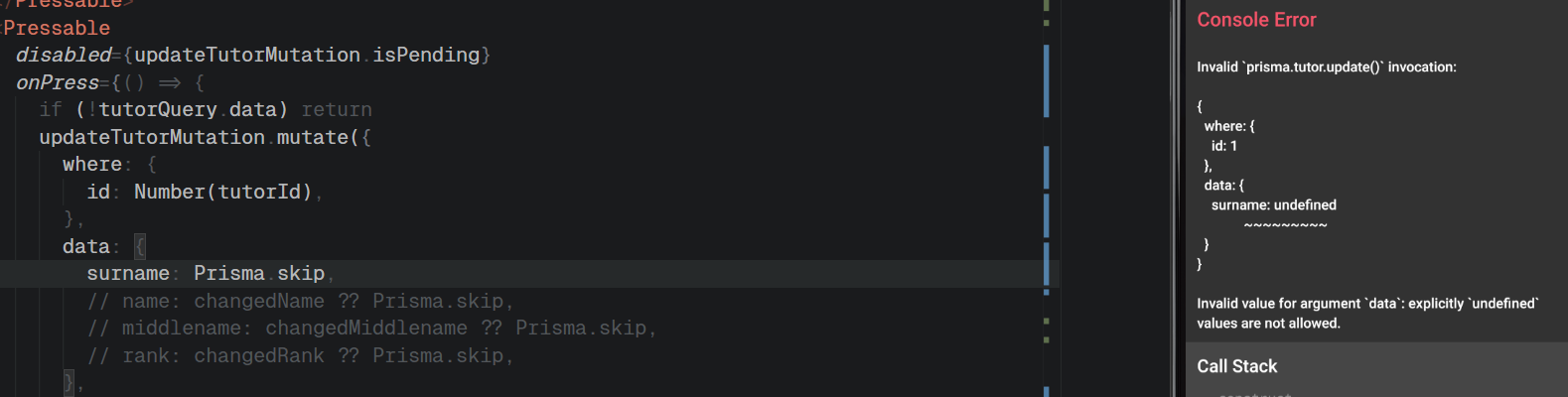
5 replies
PPrisma
•Created by piscopancer on 11/12/2024 in #help-and-questions
[React Native] DB duplicated to the emulator. Intentional?
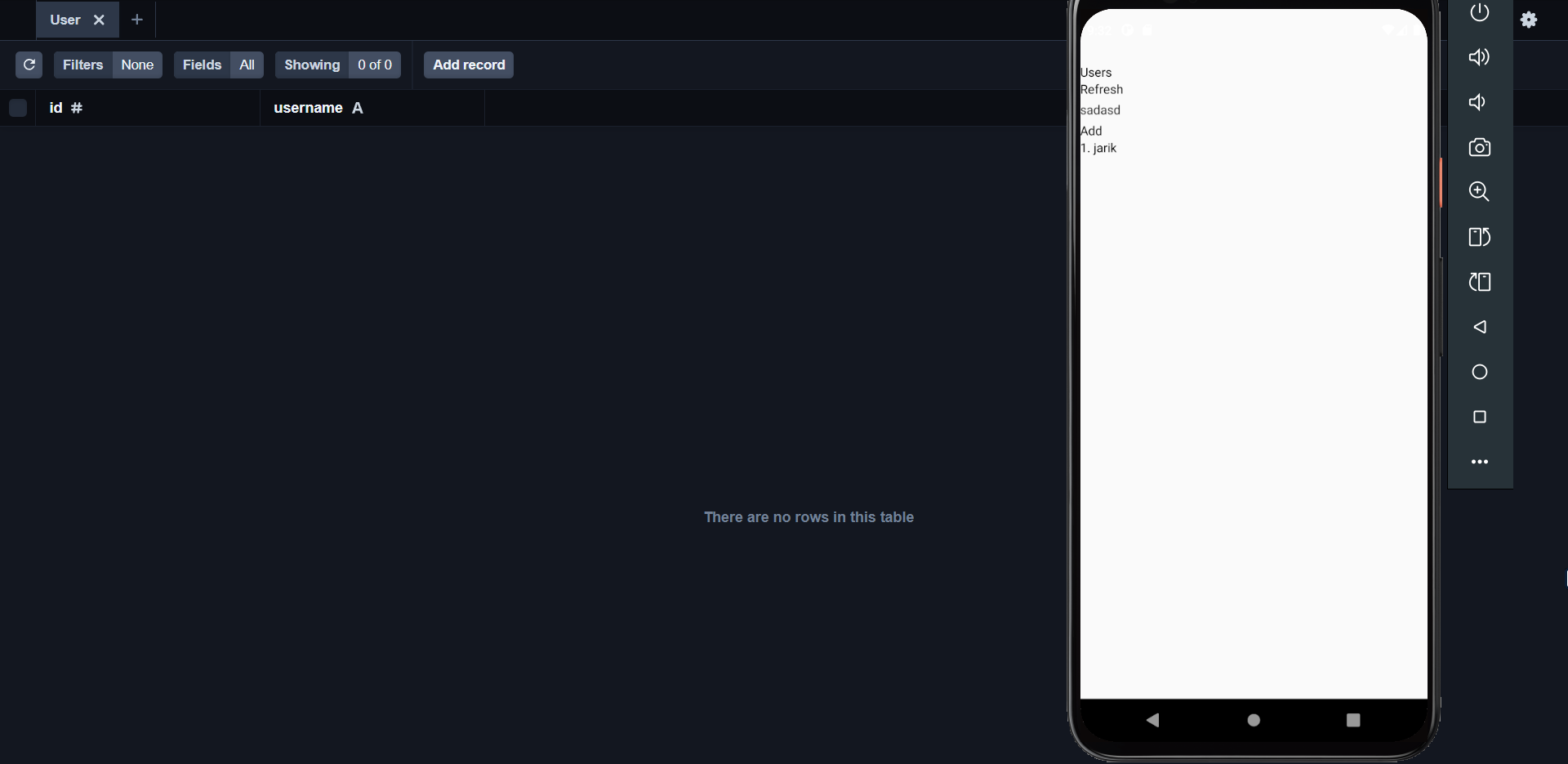
4 replies
PPrisma
•Created by piscopancer on 11/12/2024 in #help-and-questions
[React Native] Creating client with Supabase crashes
with db.ts like so (pretty basic)
the app crashes after calling this function
with the following schema
11 replies
PPrisma
•Created by piscopancer on 11/12/2024 in #help-and-questions
Prisma extension in vscode too slow
vscode: 1.95.2
prisma ext: v5.22.0
10 replies
PPrisma
•Created by piscopancer on 6/14/2024 in #help-and-questions
Get type of `create` with `connect`?
I would like to get the type for object I pass to
create
function. How can I do that? I assume I should use internal Prisma utility types, right?
The trick here is that tutor
is a required property so it must be either created or connected (in my case, connected)2 replies
DTDrizzle Team
•Created by piscopancer on 6/4/2024 in #help
need postgres explanation
do I have to start my own server for my postgres database with user and password and run in alongside my next app to be able to connect to it with
?
3 replies
DTDrizzle Team
•Created by piscopancer on 3/26/2024 in #help
how to replicate **joins** in **db.query**?
this is a
db.select
query with a join to filter out only answers that are accepted
let's say, there may be 2 accepted answers, thus this will be the response
How do I use joins in db.query
api that will result in the same response or will filter out users accepted answers in a similar way? is it even possible with db.query
? do i have to manage my relations in the schema?2 replies
DTDrizzle Team
•Created by piscopancer on 3/25/2024 in #help
count() for join tables in query API
Usage
I have a user page where you can see how many of their answers were considered correct (a person who asked a question accepted them, like on github).
3 tables are used:
*
users
* questions
* answers
each answer
has a column authorId
which refers user.id
, each question has acceptedAnswerId
which refers answer.id
Draw your attention to the extras
, what should be written there? I am not quite following21 replies
DTDrizzle Team
•Created by piscopancer on 3/22/2024 in #help
"You're about to add not-null useless column without default value, which contains 1 items"
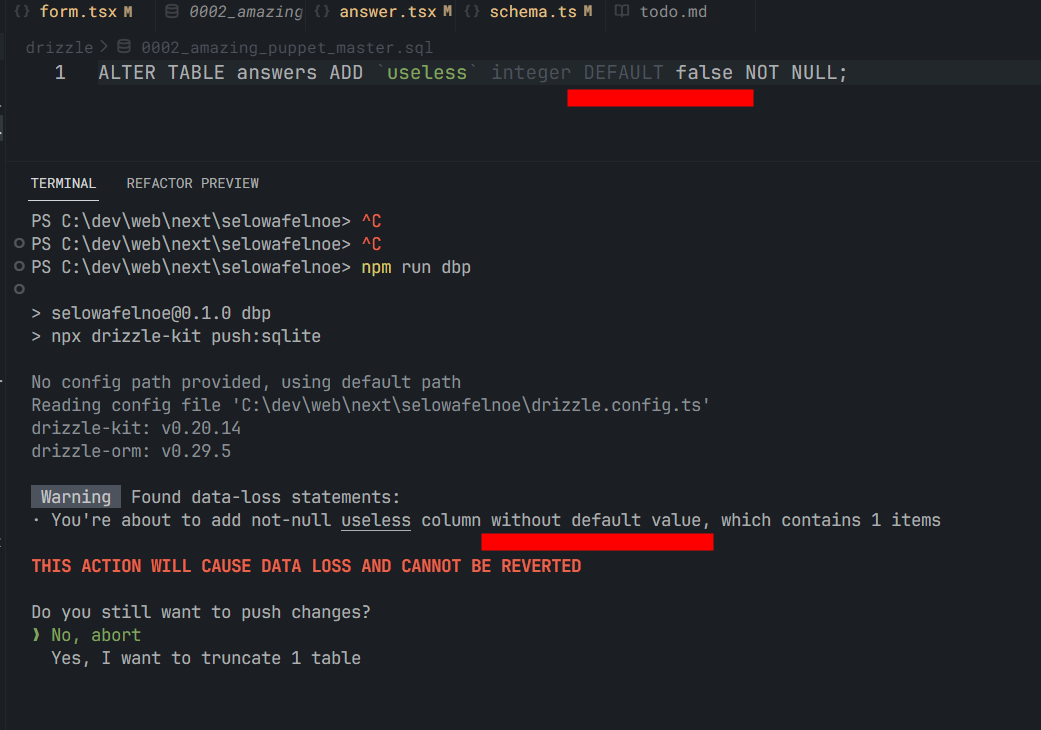
6 replies
DTDrizzle Team
•Created by piscopancer on 3/18/2024 in #help
many-to-many foreign key constraint 787 issue
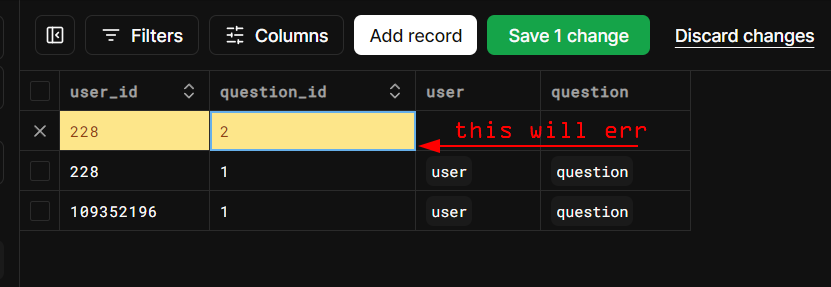
4 replies
DTDrizzle Team
•Created by piscopancer on 3/16/2024 in #help
use `count` in db.query?
I am not fully understanding the API, require support
4 replies
DTDrizzle Team
•Created by piscopancer on 3/16/2024 in #help
2 tables cannot reference each others columns?
I have the following tables declaration:
As you can see, for some reason I CANNOT reference one table's column from the other table, while this table's column is already referenced by the other table. Can you explain why so and what should I do?
12 replies
DTDrizzle Team
•Created by piscopancer on 3/11/2024 in #help
I do not understand relations 😟 (many to many, users can follow and have followers)
this is the code I tried but I messed up the relations and the error says the following:
2 replies