piscopancer
Explore posts from serversDTDrizzle Team
•Created by piscopancer on 6/4/2024 in #help
need postgres explanation
do I have to start my own server for my postgres database with user and password and run in alongside my next app to be able to connect to it with
?
3 replies
DTDrizzle Team
•Created by piscopancer on 3/26/2024 in #help
how to replicate **joins** in **db.query**?
this is a
db.select
query with a join to filter out only answers that are accepted
let's say, there may be 2 accepted answers, thus this will be the response
How do I use joins in db.query
api that will result in the same response or will filter out users accepted answers in a similar way? is it even possible with db.query
? do i have to manage my relations in the schema?2 replies
DTDrizzle Team
•Created by piscopancer on 3/25/2024 in #help
count() for join tables in query API
Usage
I have a user page where you can see how many of their answers were considered correct (a person who asked a question accepted them, like on github).
3 tables are used:
*
users
* questions
* answers
each answer
has a column authorId
which refers user.id
, each question has acceptedAnswerId
which refers answer.id
Draw your attention to the extras
, what should be written there? I am not quite following21 replies
DTDrizzle Team
•Created by piscopancer on 3/22/2024 in #help
"You're about to add not-null useless column without default value, which contains 1 items"
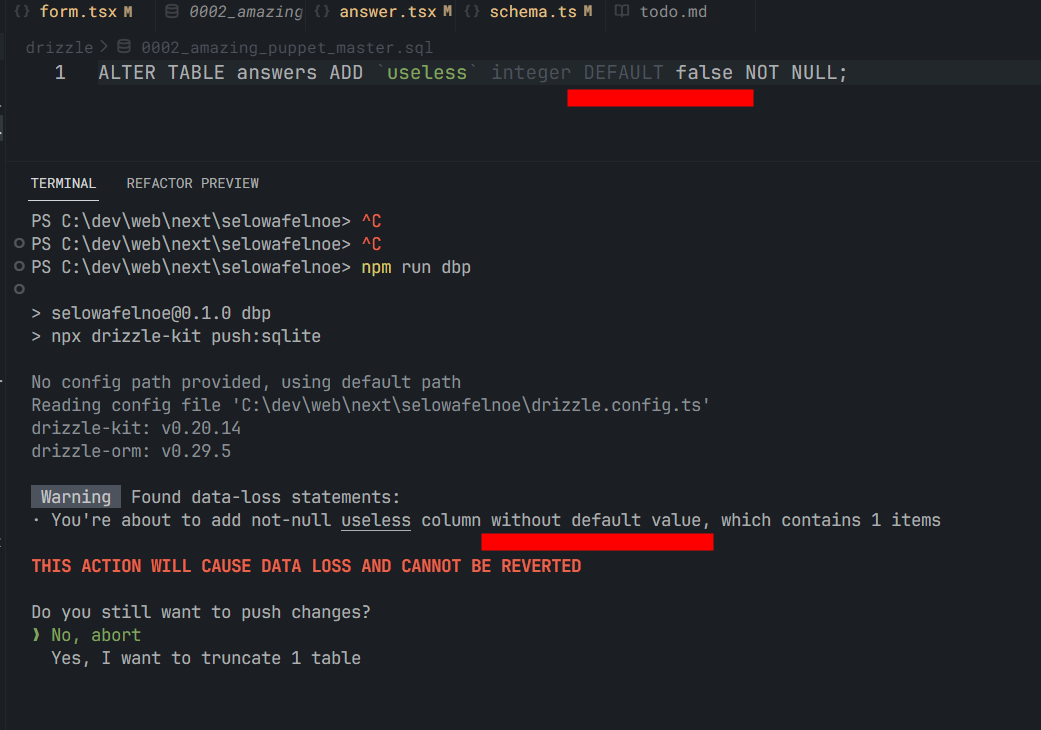
6 replies
DTDrizzle Team
•Created by piscopancer on 3/18/2024 in #help
many-to-many foreign key constraint 787 issue
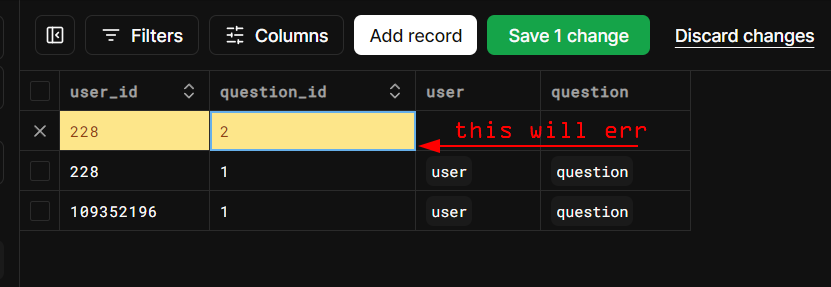
4 replies
DTDrizzle Team
•Created by piscopancer on 3/16/2024 in #help
use `count` in db.query?
I am not fully understanding the API, require support
4 replies
DTDrizzle Team
•Created by piscopancer on 3/16/2024 in #help
2 tables cannot reference each others columns?
I have the following tables declaration:
As you can see, for some reason I CANNOT reference one table's column from the other table, while this table's column is already referenced by the other table. Can you explain why so and what should I do?
12 replies
DTDrizzle Team
•Created by piscopancer on 3/11/2024 in #help
I do not understand relations 😟 (many to many, users can follow and have followers)
this is the code I tried but I messed up the relations and the error says the following:
2 replies