Luc Ledo
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Luc Ledo on 10/29/2024 in #questions
Weird tech stack from coding assignment
I just got a coding assignment that ask me to use Next.js with Fastify (backend) and tRPC. As I understand it, tRPC uses typescript to connect the frontend and the backend. I've used tRPC in Next.js as the frontend and backend. But how exactly does tRPC work when the frontend and backend are separated? Is my understanding of tRPC wrong? Wouldn't fontend and backend need to be in the repository for tRPC & typescript to work?
14 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 10/21/2024 in #questions
Need a free cursor pagination API for testing
Need something like https://jsonplaceholder.typicode.com/ but supports cursor pagination. Anything like that exist?
3 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 1/30/2024 in #questions
Best way to get a react component's raw code?
Is it possible to get a component's raw code? For example.
then something like this
Basically, I want something like this: https://ui.shadcn.com/docs/components/accordion, where I can see the preview of the component and the raw code. Technically, I can just copy/paste the component's code into the <code> element but I want to have a single source of truth. And I did try looking at shadcn/ui repo but I did not understand how it worked at all.
13 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 11/8/2023 in #questions
Passing in Form as prop in Shadcn/ui?
Basically I move FormField to another component and I need to pass in Form a props, the way I'm currently doing doesn't give auto-complete because of UseFormReturn<any>.
1 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 10/13/2023 in #questions
Is my backend team right?🤔
So I'm working on an AI image gen app and I proposed a full text search feature that would allow users to search all their generated images based on the prompt. The problem is our image collection is over 1 billion rows (mongodb btw). They pushed back and said they tried this in the past and it was not performant because the collection/table is too big and it would sometimes take up to 8 seconds. Now I believe it's probably because they were doing full text search on the full 1 billion+ table/collection, but if we query by first filtering it down based on userID first then perform the full text search on that much smaller set it would be performant right? I only know SQL database so I'm not sure but it wouldn't be similar for mongodb too? And if you know more performant way to do this, please let me know🙏
11 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 10/6/2023 in #questions
Uploadthing: Is there way to view all uploaded items?
Haven't started using it yet just to be clear but don't see any info about how to view all the uploaded items. If I lose the item url how will I interact with it from then on? Can I view all my uploaded item on amazon s3 or something like a prisma studio?
7 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/24/2023 in #questions
Can't get OG to show
https://github.com/Apestein/nextflix/blob/main/src/app/layout.tsx
site: https://nextflix-blush.vercel.app/
I can't get og to show on Discord and Twitter.
I'm following this repo example exactly, I don't understand why it won't show.
https://github.com/Nutlope/roomGPT
11 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/16/2023 in #questions
SQL Querying Best Practices?
Just want to discuss query best practices. I using drizzle here but I'm just talking about SQL in general.
And since I only need the nested relations. Relevant Drizzle docs: https://orm.drizzle.team/docs/rqb#partial-fields-select
Also, this is a query to check if a show is saved to a profile. It just returns true or false. I feel there is probably a better way to query this but I'm not sure how. Maybe a way using count?
1 replies
DTDrizzle Team
•Created by Luc Ledo on 8/16/2023 in #help
Querying Best Practices?
Just want to discuss query best practices.
And since I only need the nested relations
8 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/15/2023 in #questions
Suspense triggers weird bug
I have a component like this:
If I remove the suspense it works as normal.
1 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/12/2023 in #questions
Take over login flow from Clerk?
Is there a way to take over login flow? I'm using webhook and problem is Clerk login users before they can be created in database through webhooks. I need a way to take over login flow, await for user to be created in the database, then redirect to main page.
1 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/10/2023 in #questions
How to extract tuple from array of objects for Zod enums?
I need this for zod validation. Currently, I'm doing this way.
3 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/8/2023 in #questions
revalidatePath invalidates all route cache
Is this a known bug? I see no one mention it yet but calling revalidatePath in a server actions invalidates all route cache not just the one specified.
1 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 8/6/2023 in #questions
How to wait for state to update without useEffect?
Basically I have custom hook that looks like this:
Then I call it like this:
Of course I can use useEffect but I prefer not to.
2 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 7/29/2023 in #questions
Why Use Server Actions?
I can see the benefit of the server component version. Colocated, no need to make an api endpoint in another file.
But isn't the client component version just another way of writing API endpoints?
Guess you can use it in form without javascript:
But using it this way feels sucky as there is no way (that I know of) to make it typesafe.
4 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 7/28/2023 in #questions
Does server actions input have to be validated server side?
Are they like exposed api endpoints or are they private?
Would validating client side using input props like require/max-length work?
2 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 7/24/2023 in #questions
This Shouldn't Work But It Does🤔
https://github.com/Apestein/async-client-demo/tree/main
This is an async client component, this should not work and yet it does. The console.log will show up on client. It will not let you use useState but onClick will work.
If you do
export default async function
instead then it will give you an error as expected but export async function
will work.7 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 7/23/2023 in #questions
Clerk Forces All Routes To Be Dynamic
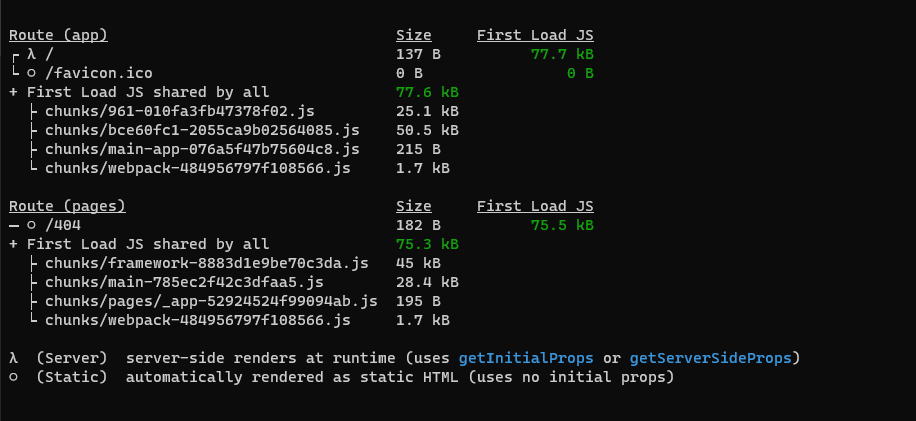
7 replies
TTCTheo's Typesafe Cult
•Created by Luc Ledo on 7/17/2023 in #questions
Intercepting Modal Cause Jump To Bottom Of Page
Live: https://nextflix-blush.vercel.app/
Repo: https://github.com/Apestein/nextflix/tree/main/src/app/(main)
Any ideas why this might be happening?
4 replies