The Typhothanian
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 1/27/2025 in #java-help
Need ideas for separating my client, server, and common code for my game
Title isn't good, but it's the best I can think of. I've been working on a game for almost 17 months now, and when I just tried to add multiplayer, I came across an issue. I have my world separated into modifiable chunks. These chunks have code for rendering and storing the world data inside. Both client and server need the storing part, but only the client needs rendering part. I can't think of a good way to separate them so that both client and server get their versions of common, but client having the rendering stuff. I also want my games to be able to have mods that run on client and server. The rendering code is far too much to feasibly use but code manipulation to inject at compile (and I also wouldn't have complete source code). This is very frustrating, as I thought I would need only a few more weeks to be able to release my game. Now I have to refactor the entire thing. The point of this post is to ask for ideas to fix this. Please help, any suggestions will be appreciated.
13 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 1/5/2025 in #java-help
Ensure method is called after all subclass constructors have finished but before object used
I am making a game and have a class that could be extended a lot, with multiple levels of classes. The subclasses may implement a wide arrange of interfaces. I have ran into an issue where calling instanceof or Class.isAssignableFrom causes significant overhead. I decided to cache possibly null instances of each interface in the object (this is a "type" object so there won't be many of them), and the fields are initialized at construction, like this:
That works fine, but then I run into the issue where the interface is just a getter, and I need the object:
But when the returned value is a variable in a subclass that is passed into constructor, it isn't initialized at that point, so it always returns null. Simple solution, right? Store the getter rather than the value. BUT the returned value has some interfaces it could implement that could also be implemented by the subclass because IValue implements IGetter and returns itself (for as many options as possible), so we do this:
and that's where the problem is. I need the value to be initialized when the value2 init code is called. However, the code should only be called once per object for efficiency, and after the last subclass constructor has finished but before any modders have the opportunity to call something on the object and mess it up. Even if I moved the container class from being a superclass to being a field in the subclass, the same issue would show up. Factory method(s) won't be feasible here.
5 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 11/18/2024 in #java-help
LWJGL missing EGL .dll
I hope someone here can help me with this. I just tried to add EGL to my game (for shared contexts), and it can't find the egl .dll file. Build.gradle:
If I try adding gradle can't find it? Please help, been struggling with this for an hour.
40 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 10/26/2024 in #java-help
Override Java awt repaint system (or at least make a paint listener)
I'm trying to hook up Java awt to a glfw window. I have a container (no parent) with some children, like a button. I want to know when the container is trying to repaint an area, then make my own repainting system that sends the painted content to a texture to be rendered. How can I do this? I've tried overriding repaint and paint in the container, and they don't get called. I don't want to have to insert code for all components that I add to the container. Is there some paint listener thingy I can use to do this?
Also, kinda related question. If the container is trying to paint a specific section, I create a BufferedImage, create a graphics for it, then tell the container to paint to it, right? But it would just paint the whole window to that image, but I only want that specific section. And is there a better way than the BufferedImage? I need the output to eventually go to a ByteBuffer.
Hope this is clear enough
107 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 4/20/2024 in #java-help
Load image file into int[]
Hi. I want to load an image. I would like support for png, jpeg, webp, bmp, and (if possible) gif. I found a good source for png (http://www.libpng.org/pub/png/spec/1.2/PNG-Structure.html), but nothing for the others. I don't want to use ImageIO then read from the buffered image, as that is slow. What I am asking for is how the bits of each type are laid out, so I can construct my own image loader. Also, the int[] is a flattened image, with the index of x and y being x + y * width. I am making my own window and game library, and don't want to use the stupidly slow java awt or java swing utilities. Please help.
10 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 4/4/2024 in #java-help
JNI C++ OpenGL Window Crash
Hi. I'm new to C++ and JNI, and just started yesterday. I am trying to make my own OpenGL window system. (yes, I know that is a big task, and I don't care)
I got a window a while ago, but now there is a weird issue. I get this console output java-side:
1 Invalid window handle.
2 Invalid window handle.
3 Invalid window handle.
4 Invalid window handle.
...
4 The system cannot find the file specified.
...
1 Invalid window handle.
2 Invalid window handle.
3 Invalid window handle.
4 Invalid window handle.
...
The numbers at the start of each line indicate where in my C++ program the issue is coming from. I don't know why the middle one is there, because I'm not loading any files. The "invalid window handle" comes from resizing one dimension of the window, and the crash comes from resizing two at the same time. My code is in comments. Please help.
12 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 3/31/2024 in #java-help
Java Socket NIO Reading Issue
(code in comments)
I have a server and a client. I am trying to transmit a long from the server to the client (or reverse, neither works). I can tell it is transmitting, but the reciever gets hung up on the socket.read(buf) line in readLong. Please help. I just started NIO this morning and have no clue if I'm doing it right.
7 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 3/14/2024 in #java-help
Java Swing MouseListener issue
I'll have to segment the code in comments because it's too large for Discord. I have a frame, a container, and two components in the container. I made the components draggable with
It was working a few minutes ago, but now the mouse listeners aren't being triggered. Full code in comments
13 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 3/9/2024 in #java-help
Collision Between Non Axis Aligned Quadrilateral Hitboxes
I have two List<double[][]>s representing several 3d quadrilaterals. They are not axis aligned. I would like to perform collision between them, and push the second one away appropriately. How can I do this? It needs to be efficient enough to work once or twice a frame in a game. Please help
4 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 3/7/2024 in #java-help
JOGL glUniform4fv issue
Here's my set uniform code:
And here's the error:
com.jogamp.opengl.GLException: Thread[#39,AWT-EventQueue-0,6,main] glGetError() returned the following error codes after a call to glUniform4fv(<int> 0x0, <int> 0x1, <[F>, <int> 0x0): GL_INVALID_OPERATION ( 1282 0x502)
Why is there this issue? Shader init code in comment.
7 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 3/3/2024 in #java-help
Get list of all loaded classes
Title says it. Need list of all loaded classes at one moment. Planning on using URLClassLoader for mods, so I can't just make a list of every class in my program.
5 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/26/2024 in #java-help
HashSet.contains not working as expected?
I have a HashSet<Face>. Here's face:
If I create "idential" faces (the texCoords and vertex order aren't same, but have same vertices), Face.equals returns expected value. However, if I do that for a HashSet, it doesn't work. An ArrayList works fine, but I need a Set. What can I do to fix?
10 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/24/2024 in #java-help
OpenGL Occlusion Culling?
I'm making a 3D game with JOGL. I expect to have a world layout like Minecraft, with a lot of cube-like models. That means I can skip faces that touch each other (occlusion culling) to speed up drawing. I want to be able to remove the indices for specific models to make this work. But, I'm using VAOs, which are not meant to be modified or read from the CPU once written. Is there a way to do this? Using JOGL GLJPanel with VAOs and Shaders. Code in comments bc I am running out of space.
6 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/23/2024 in #java-help
JOGL Model Issues
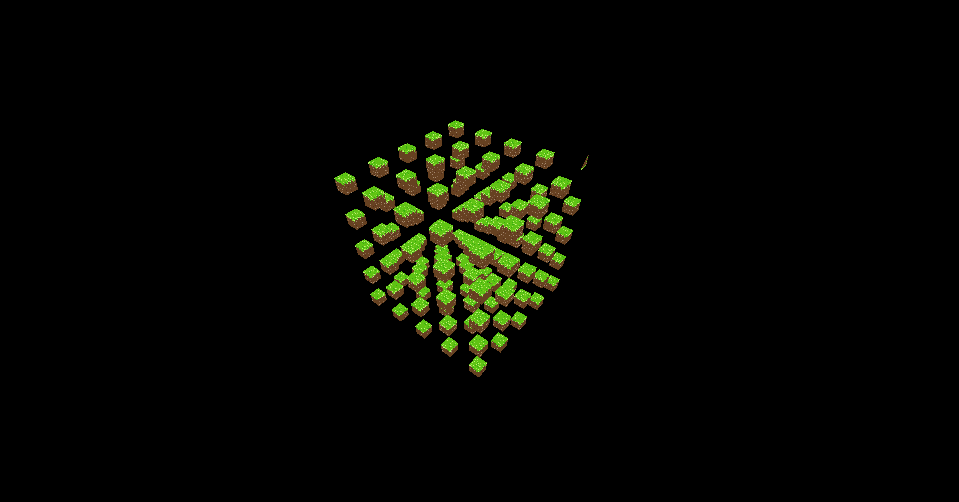
9 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/21/2024 in #java-help
Looking for JOGL VAO/VBO Tutorial
Title says it all. I am making 3D game, it was fine with GL2, want to upgrade to GL3. ChatGPT/Microsoft Copilot not helping. Using JOGL 2.5.0
6 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/19/2024 in #java-help
Java AWT Move mouse automatically without triggering MouseMotionListener
I'm making a game with JOGL. I made a GLJPanel, and got everything set up. However, I have trouble with the camera rotation. Here's my mouse listener:
As you can see, it should work fine. However, the Robot class re-calls this MML with inverted mouse X and Y, so the camera just snaps back with the mouse. Is there a way to fix this?
12 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/16/2024 in #java-help
Connect LWJGL to JComponent?
The title pretty much says it all. I want LWJGL to draw to a component in my JFrame, instead of a GLFW window. What's the most efficient way I can make this work, if it is possible? Thank you in advance.
6 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/14/2024 in #java-help
Help with "Spreadsheet"
I am making a 3D game. For this game, I need an efficient way to store and access block data. I want to be able to
- find any block at specified z coord,
- get all blocks of same type,
- get average coord for block in chunk,
stuff like that. I have an idea for this, but I'm not sure if it would work for primitive types. As the title suggests, I thought of a spreadsheet. Separate columns (or lists) of each variable, and each row makes up an Object. A cell should automatically update when the row's value is changed, and the row's value should be changed when the cell is changed. I know this is easy for non-primitive types, but I am getting confused on primitives. And, if the variable is set in one of them instead of updated, it wouldn't propogate to the other and the link would be broken. (sorry if I am not using right terms, new to java). I think reflection can do this, but I have no clue how to do it. Please help?
60 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/6/2024 in #java-help
javax.sound.sampled cannot change volume of clip (part 2, closed before properly testing)
oops. go here for what I have so far: https://discordapp.com/channels/648956210850299986/1204195625168805898
The summary is that I can control one or two things in my audio clip, but they don't seem to change anything. Also, volume, the one I want, is still unsupported. Here's what the code looks like currently:
Stuff like master gain works, but again, doesn't change anything. Putting before starting makes them all unsupported. Help?
31 replies
JCHJava Community | Help. Code. Learn.
•Created by The Typhothanian on 2/5/2024 in #java-help
javax.sound.sampled cannot change volume of clip
The method above should play a .wav file at the specified volume (file is a byte[] loaded from the .wav).
However, Java tells me that it's an unsupported control type. I've tried everything and none of it works. Am I just missing something obvious? This is what the oracle site told me to do. I have a windows 11 surface pro 7, and Minecraft can change audio volume, so it's not my device. Help?
32 replies