Zamiel
Explore posts from serversDIAdiscord.js - Imagine an app
•Created by Zamiel on 10/20/2024 in #djs-questions
How do add a user to a thread silently?

23 replies
DTDrizzle Team
•Created by Zamiel on 1/1/2024 in #help
How to perform multiple queries?
I have multiple queries that I would like to do at the same time in order to save network traffic back and forth N times.
I see that the "Batch" section of the docs applies to LibSQL and to D1. But I am using Postgres.
Do I have any options in Postgres?
(Besides just writing the SQL query raw I guess, which I want to hopefully avoid.)
19 replies
DTDrizzle Team
•Created by Zamiel on 12/18/2023 in #help
How do I use NOW() in Drizzle?
Can someone explain how to write the following query in Drizzle?
6 replies
DTDrizzle Team
•Created by Zamiel on 11/27/2023 in #help
How to check if a row exists in Drizzle?
According to this StackOverflow post:
https://stackoverflow.com/questions/7471625/fastest-check-if-row-exists-in-postgresql
The most performant way to check if a row exists is as follows:
How do you accomplish this in Drizzle?
6 replies
DTDrizzle Team
•Created by Zamiel on 11/27/2023 in #help
What are the Drizzle conventions for giving names to Drizzle return types?
Hello all, I have a question about Drizzle conventions. Do people give names to the return types of their Drizzle calls?
For example, consider the following code:
- Here, we use the
ReturnType
utility type from TypeScript to satisfy DRY. (If we wanted to violate DRY, we could repeat all the user fields inside of a dedicated User
TypeScript interface
.)
- However, we now have a problem where Intellisense does not work properly. In other words, if we mouse over user
in the following code:
We get the full enumerated object inside of a union with undeifned
, instead of the much easier to read and understand User | undefined
.
Does anyone know if there are Drizzle conventions to get smarter Intellisense here?40 replies
DTDrizzle Team
•Created by Zamiel on 8/14/2023 in #help
What is the Drizzle convention for storing the `db` object?
In the "Quick Start" guide, it showcases connecting to the database at the root of a module.
However, in real code, this would likely be in a file called "db.ts", and it would not be at the root of the module, since the connection string would not yet be available.
This is my naïve attempt to show what a more real-life code would look like:
And then, elsewhere in the code:
But here, I export a "let" variable, which flags ESLint and suggests that I am architecting my code incorrectly.
Is there a more conventional way to handle the "db" object?
57 replies
DTDrizzle Team
•Created by Zamiel on 8/12/2023 in #help
Does Drizzle ORM support CommonJS (CJS)?
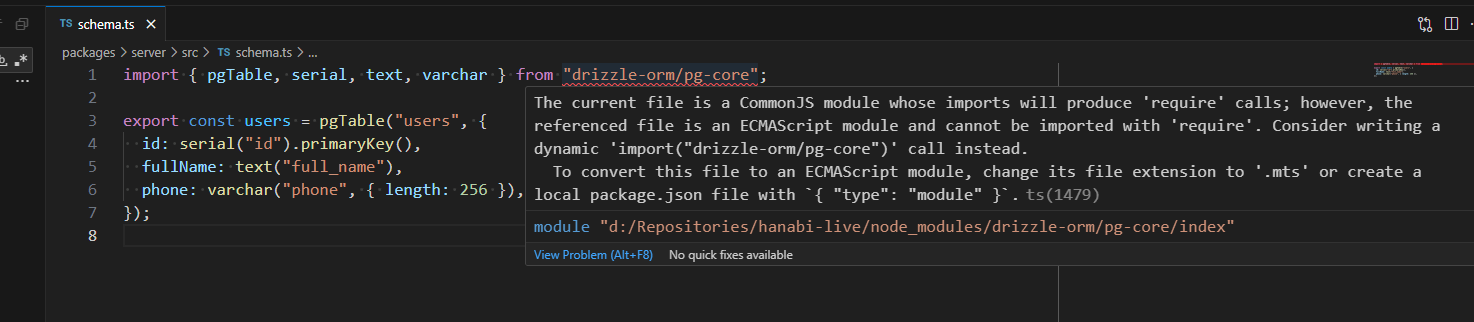
67 replies
TTCTheo's Typesafe Cult
•Created by Zamiel on 8/7/2023 in #questions
How to use readonly arrays with immer?
Consider the following short code snippet:
https://www.typescriptlang.org/play?#code/JYWwDg9gTgLgBAbzgESgQwGbwL5w1CEOAIlBAFMpiBuAKFuADsZKM0BjcuAWQE8BlGGhaJacOFHJoAJhEYAbXnBACArgCNBw8gC4eazUJZ1s9JiyhtO+-hq0iEYiVNkKlAZ0LlBUJgHM9dxhfRj86cUkZOUU4Twoff3c9SNcYoJC-AG0AXRN6DFVGdhhgOTg-chhkcjZVeRg+W0NtAAoASj1GuyMuRwjK1ShGUXFxOO9g-z1iDAgIYgAaJzGvBNCkuEyZucWSdTQqbKXxU1NaAqKSspUAJXJpVU4oADFC4tLGFqDtPVRMGAAPI0egA+NojODsORBZQGexcAC85Uq1Vq9S6zRY7XCsR6ADoVE14XAkYTuto8kA
Immer seems to have a problem with readonly arrays, which doesn't make sense to me, because your state should always be immutable, which is the whole point of using immer in the first place.
Before Immer version 7, this pattern worked fine, but in Immer 8+, it throws an error.
How do I work around this problem or structure my code in the correct way?
2 replies
DIAdiscord.js - Imagine an app
•Created by Zamiel on 10/31/2022 in #djs-questions
How do I write a `isVoiceChannelEmpty` function?
In previous versions, the code I wrote above would be how to find out if a voice channel was empty.
However, this code no longer works in the latest version.
How can I write this function?
8 replies
TTCTheo's Typesafe Cult
•Created by Zamiel on 10/5/2022 in #questions
Alternative to TypeScript Classes
Currently, I'm refactoring TypeScript code into classes because I want to use extends for N features to inherit some base functionality.
Previously, I had pockets of functions organized in separate files based on the type of functionality that they were providing. (foo.ts, bar.ts, baz.ts)
I figured classes would be better to stay DRY, as it removes a lot of copy-paste boilerplate between every feature. (common variables + common functions)
But I'm finding it really difficult to transition this structure into classes, because there are shared variables in a feature directory (
variables.ts
), and there is no partial
keyword in TypeScript like there is in C#, so I have to stick thousands of lines of code in the same file (to avoid circular imports).
As a result, I'm brainstorming ideas about how I could use normal objects to emulate classes, but it seems very messy.
Are there any blogs that describe some patterns that might be useful here?2 replies