MaveriX89
Explore posts from serversBABetter Auth
•Created by MaveriX89 on 4/7/2025 in #help
New to SaaS Application Building
Hey all, I'm curious what people do surrounding authentication/authorization for SaaS apps. I'm new to the arena and wondering what the different solutions are. So if I intend to deploy an application across multiple tenants who have their own IdP (e.g. Azure, AWS, GCP, Logto, etc.) that I want my app to interface with, what Better Auth plugin should I be using? Organization? OIDC? SSO? A hybrid mix?
If I hook up to their IdP, then I need a way to map their IdP defined roles to the app roles that my application will define. How do people typically manage that IdP configuration for the app and IdP role to app role mapping? At deploy time? Runtime check with some administrative UI pages built into the application?
Sorry if these are dumb questions, but curious to get insight as I'm trying to build a scalable SaaS app.
14 replies
BABetter Auth
•Created by MaveriX89 on 4/4/2025 in #help
Organization: Determine what team a user belongs to
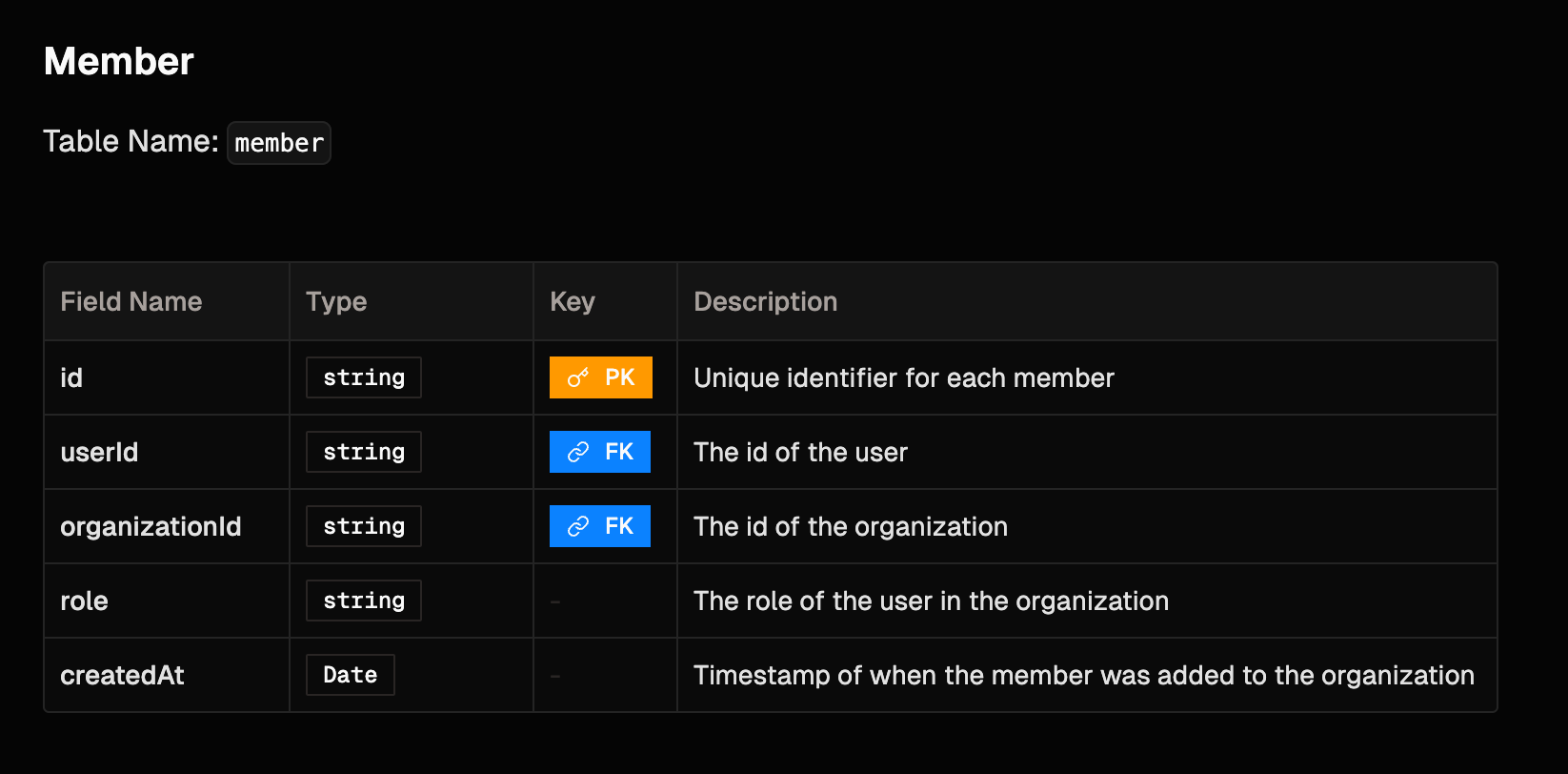
11 replies
How to Abstract Routers using Classes
I'm trying to create a library that leverages a subset of routing functions under the hood. With the release of TanStack Router for SolidJS, I do not want to couple the library specifically to Solid Router and want to provide a
Router
abstraction that consumers of the library can configure with their router of choice. The way I'm going about that is providing abstract Router classes. Below is one example:
The same pattern above is applied for TanStackRouter
as I dynamically load the library in the constructor and implement the same interfaces with all the things that TanStack Router expects. I have two primary questions:
1. Is the above paradigm a good way of going about providing the abstraction over routing? If not, what's another way that allows users to configure routing.
2. Every time I use the class, I am getting an exception at the point of the navigate
interface being called despite it being invoked while being a descendant of a <Router />
component in the JSX tree:
Not sure how to debug the error. I suppose it has something to do with invoking const _navigate = this.router.useNavigate();
in the context of a class and not a component? 🤔1 replies
BABetter Auth
•Created by MaveriX89 on 3/24/2025 in #help
How to Ensure Organization List API is authorized
I'm currently trying to use Better Auth and pair it with TanStack Query to optimize request caching as I want to dedupe multiple of the same requests that I see firing in my application.
The question I have is, instead of using the
client.useListOrganizations
hook API that the docs recommend, I am using the raw client.organization.list
API and wrapping that within the TanStack Query function like so:
The issue is, after signing in via email using the Better Auth client, invoking that list API call results in me receiving at 401 Not Authorized response.
Can someone point to me what I'm missing to get that API to work properly after having the Better Auth cookies set properly post sign in?2 replies
BABetter Auth
•Created by MaveriX89 on 3/16/2025 in #help
Better Auth ABAC (not RBAC)
Anyone have an example of doing ABAC using Better Auth? Just curious.
1 replies
BABetter Auth
•Created by MaveriX89 on 3/12/2025 in #help
Adding Members to Organization with Teams Enabled
I am using the
organization
plugin with teams
enabled and am trying to add members to the organization/team via the addMember
API call:
I am trying this but when I inspect my database table, I do not see the teamId
being recorded into the database column. What am I missing?18 replies
BABetter Auth
•Created by MaveriX89 on 3/11/2025 in #help
Admin + Organization Plugin: Role Columns
I have a question about using the
admin
and organization
plugins together. When using the admin
plugin, it adds a role
column to the user
table and when using the organization
plugin, it creates a member
table that itself contains a role
column along with a userId
column that references the earlier user
table.
The question I have is, how should the relationship between those two distinct role
columns be managed? What are the expectations?54 replies
BABetter Auth
•Created by MaveriX89 on 3/7/2025 in #help
Database Seed Script Using Organization Plugin
Anyone here using the
organization
plugin and made a local database seed script that populates your database with a fake organization and users in said organization? If so, can you share what that looks like? Step-wise sequence is:
Create organization
Invite users to organization
Accept invitations
But how does one do step 2 of the process in a seed script? Is it possible to skip it all together and directly add users to the organization using the server API?3 replies
DTDrizzle Team
•Created by MaveriX89 on 2/16/2025 in #help
Recommended Docker Compose Workflow
Hey all, I'm new to making full stack applications and am working with Drizzle to make mine. I have a question on what the best practice is when using databases that are spun up using Docker Compose and how to initialize them with Drizzle tables.
I have the following
docker-compose.yaml
I am using a code-first approach with Drizzle where I want my codebase to be the source of truth for the database since there is tight coupling between the DB and my API server + SPA. The question I have is, when my app is being spun up for a production deployment, how can I ensure that when my PostgresDB from docker spins up, it also prepped with my Drizzle tables via running drizzle-kit push
when it comes alive?
Essentially, I want to make sure that the definition of my database container being "ready" means, the Postgres container is up, and I pushed my Drizzle tables to it (alongside any optional seed script I decide to run -- I actually have a seed_db.ts
script that I typically invoke locally using bun ./scripts/seed_db.ts
which I would like to run for local dev workflows)2 replies
Component in test is not updating despite signal change
I am currently writing some tests for a library I'm building with Solid. I have the following test:
So the context summary is, I have two primary things under test:
SSOProvider
and the useSSO
hook. As you can see above, I have an internal test component called AuthStateComponent
that is using the useSSO
to either show an authenticated or unauthenticated view. The issue is, when I run my test and trigger a user update, the test component does not re-render to show the authenticated view. It always remains in the unauthenticated despite the fact the user has been updated internally within useSSO
(I verified this via console logging everything).
So that little createEffect log that I have within the test component only ever runs once, outputting a null
, and it does not re-run after the user signal updates internally.
I'm currently stuck in testing this particular area and can't proceed. Not sure exactly what I'm doing wrong here.2 replies
How to filter/remove elements from an array within a Store
If I have the following
createStore
invocation:
What is the proper way to filter/remove an element from that cards
list? I have tried the following and I get yelled at by TypeScript saying it's incorrect.
Since the cards
field is at the top level, is the only option to reference the previous value of the store?
6 replies
Exception thrown when invoking useNavigate within Router root layout
I am having a real hard time working through this problem. I feel like I've tried everything but nothing has been working and there's probably something I'm not seeing clearly anymore.
The short of it is, when I attempt to invoke
navigate("/login")
from my root layout component, I am greeted with the following exception:
Here is the entirety of my App.tsx
file:
The area to focus on is the onClick
for the Sign Out NavbarLink
. In the callback, I am invoking the Better Auth client to sign out and on success, I navigate to /login
. It's that invocation that causes the exception.
I'm not exactly sure the proper way to debug this and could use all the help I can get33 replies
BABetter Auth
•Created by MaveriX89 on 1/21/2025 in #help
Better Auth + Solid Start
When using Better Auth within Solid Start -- after doing all the required configuration, do we simply use the Better Auth server client (instead of the client one) to invoke all auth related matters? So given a Solid Start action like below:
Is the above the expected way we interface with Better Auth on the server? Or is there some other behind the scenes stuff happening due to the below configuration?
Or lastly, should we still be using the Better Auth client on the frontend and do:
3 replies
SolidJS SPA with Better Auth
I am trying to build a SolidJS SPA that uses the new Better Auth library to manage all things related to authentication/authorization for my app. Post all the needed configuration for it, on the SPA side, I have to use the Better Auth client to invoke all the auth related methods on my UI. I have one example here that I'm wrestling with in trying to get a redirect to happen after the user signs in but the redirect is not happening on the Solid SPA side:
My Better Auth Client configuration:
My
SignIn
component:
I'm suspecting that perhaps, the issue has to do with the fact that I am doing the throw redirect
inside of the onSuccess
handler of the Better Auth client as opposed to the scope of the action
itself? Wondering if others have attempted this yet.5 replies
TanStack Query vs Solid Router
I'm looking to use Solidjs for a production app and have a question about a library I typically reach for (TanStack Query) for my SPAs and some of the built-in APIs for Solid Router:
query
+ createAsync
.
Is there any reason to use TanStack when Solid Router comes with those built-in? Just wondering if there is something I'm missing because, the query
+ createAsync
combination if I'm not mistaken provides a lot of the same benefits that TanStack does with request de-deduping and caching by key. Wondering if there are other gaps present that I am not privy to (e.g. easy request refetching/polling on interval or conditional fetching like the enabled
field from TanStack Query).
For the visually inclined -- the question is what is the recommendation between these two options and the pros/cons of each:
Option 1:
VS
Option 2:
P.S. Just throwing it out there but, man, Option 2 look really good haha17 replies
BABetter Auth
•Created by MaveriX89 on 1/21/2025 in #help
Getting UNPROCESSABLE_ENTITY when attempting to sign up a new user
Hitting another issue with Better Auth where I get an error when attempting to do the following:
I receive this error:
I'm wondering if this is because Better Auth does not like the fact that I modified the primary keys of the default Drizzle tables it generated for me to use
uuid
instead of integer
?
Appreciate any and all help with this3 replies
BABetter Auth
•Created by MaveriX89 on 1/21/2025 in #help
Getting INVALID_USERNAME_OR_PASSWORD Error when using correct credentials
I am new to Better Auth and am using the
username()
plugin to enable username/password logins in my ElysiaJS backend. I have the following configurations for both the server and client for Better Auth:
I made some changes to the default Drizzle tables that Better Auth generated in my project:
With the above said, when I am doing local development, I am spinning up a Postgres docker container and run a custom seed script that I have to populate the tables with fake data. I'm probably not doing something correctly there in that step because when I attempt to log in with the fake admin
user I added to the UserTable
, I keep getting INVALID_USERNAME_OR_PASSWORD
back as a response.
Here is a small snippet of what I am doing:
Has anybody else run into this problem when using the username login?1 replies
BABetter Auth
•Created by MaveriX89 on 1/21/2025 in #help
Help in resolving CORS issue with Better Auth
This is my first time using Better Auth and I am pairing it with Elysia and a Solid SPA. The Elysia backend is a dedicated backend-for-frontend that will serve the frontend in production so they will share the same origin url.
I am currently running into CORS issues when I attempt to sign in using the username plug-in. Below are my configurations:
For my frontend SPA, here is my
vite.config.ts
. The thing to note about it is the server.proxy
configuration I have defined. When my frontend makes a request to http://localhost:3000/api/*
it'll route it to port 8000 instead as that is the port where my ElysiaJS backend runs on in a sidecar shell.
My frontend Better Auth client config is the following:
Moving on to the backend side of things, here is my Better Auth server config:
And here is my Elysia setup for Better Auth:
On the frontend, when I attempt to execute authClient.signIn.username(...)
I get the following error:
Clearly, http://localhost:3000
origin is not on that list which is why I'm getting hit with CORS, but I guess I'm wondering the question why since http://localhost:3000/api/auth
is there. And what is the recommended way to resolve this? Hoping to avoid explicit origins..1 replies