Trader Launchpad
Explore posts from serversDTDrizzle Team
•Created by Trader Launchpad on 4/29/2024 in #help
How to structure posts with categories using drizzle and postgres (supabase)
I have been working on how to set up businesses, categories, and businessToCategories (join) tables and properly querying so that I can pass an array of category ids and only get back the related posts. I am trying to use the query api so that my return types are consistent.
I should be able to ask for all posts with a category id of [1,2] and it return just those posts. Currently I am able to retrieve all businesses with their businessToCategories id and then finally the nested category name and icon. How can i filter via the initial where clause referencing a relation?
I have the three tables defined in schema and pushed live:
8 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 2/16/2024 in #questions
CTA v7.26.0 is giving "fetch is not a function" error
I have a boilerplate create t3 app@latest and installed 1 package the monday-sdk-js package.
It works fine with [email protected] (nextjs 13) but is throwing an error on [email protected] (next14). Next13 has to have the experimental server action flag and next14 does not. Same code on both boilerplates but on next14 I am now getting error:
Any idea why this is happening?
7 replies
Thoughts on how to integrate t3 app, connectkit web3 auth, nextjs middleware, and trpc
I am prototyping an application using t3 app with trpc, connectkit web3 auth.
I am wanting to use nextjs middleware to protect routes server side.
As part of the connectkit auth flow, I have my application wrapped in a ClientProvider:
Inside ClientProvider I have the web3 providers:
and here is const siwiConfig. It calls the /siwe route on initial load and sets a cookie using iron-session with the nonce inside, then updates that same cookie after authentiucation with wallet address and chain:
4 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 2/14/2024 in #questions
Thoughts on how to integrate t3 app, connectkit web3 auth, nextjs middleware, and trpc
I am prototyping an application using t3 app with trpc, connectkit web3 auth.
I am wanting to use nextjs middleware to protect routes server side.
As part of the connectkit auth flow, I have my application wrapped in a ClientProvider:
Inside ClientProvider I have the web3 providers:
and here is const siwiConfig. It calls the /siwe route on initial load and sets a cookie using iron-session with the nonce inside, then updates that same cookie after authentiucation with wallet address and chain:
4 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 1/29/2024 in #questions
Difference between NextAuth + firebase adapter, NextAuth + drizzle adapter, and just using firebase?
I have three t3 templates scaffolded.
One with Nextauth + firebase adapter.
One with Nextauth + drizzle adapter -> Planetscale
One with just Firebase (next-firebase-edge)
I am trying to understand the differences between these three and why I would go with one vs the other.
With NextAuth + drizzle, all auth and storage of the auth tokens is handled within my app and stored on my own server. Firebase services are not used at all.
With NextAuth + firebase adapter, the auth logic is handled in my app but the records are stored in Firebase. No users show in the Auth section of the Firebase UI console.
With just firebase installed (standard client only or next-firebase edge (allows ssr)) all auth logic is handled by Firebase, and I see no storage of auth tokens its stored by Firebase and I have no ownership. Users do show in the Auth section of the Firebase UI console.
Now lets say I want to add an expo app in a t3 turbo monorepo:
With NextAuth + drizzle, I understand that I would have to write my own logic to mimic what Nextauth does inside my webapp for auth.
With NextAuth + firebase adapter, its possible for me to sign in the user in to Firebase with a firebase customToken during the Nextauth auth flow. The user now shows in the Firebase Auth UI console. The user seems to have 2 sets of auth cookies now. One for nextauth and one for Firebase. Is this a bad practice? Could i now use the Firebase auth logic in my expo app (it works with react-native) even though I am using Nextauth for most of the logic in my webapp?
With just firebase, I conceptually understand that I can use the firebase packages in my webapp and mobile app to handle auth.
What is the best route?
6 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 1/28/2024 in #questions
Why is there no SessionProvider wrapping app in next13+ app router boilerplate?
When comparing a t3 app boilerplate with nextauth in pages directory vs app router, the _app.tsx in page directory is wrapped in an SessionProvider and not in app arouter.
Why is this so?
7 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 1/9/2024 in #questions
How to find single record in database using trpc and drizzle?
I am trying to do a simple database lookup finding a user by a custom field discordId.
I have added that id to my drizzle schema and pushed to the database:
and now I am trying to create a trpc route for getting the info:
but I am getting an error:
looking at a similar process with prisma, the database structures are models and not simple 'consts' should the databasse structure type be automatically inferred or so I need to custom define this?
11 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 1/8/2024 in #questions
How to?: Sign In With Ethereum and have a user record created?
I have an application running and just got Sign In With Ethereum 'working' with nextauth/drizzle orm adapter/planetscale, but nothing is stored in the database user records. When i log in with different oauth providers (discord) i get new records added.
Should this be creating new records in my database by default, or is the nextjs discord provider coded to do that, and I need to write that programming in the authorize callback to handle it?
Here is my nextauth auth.ts file:
5 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 11/10/2023 in #questions
[TRPC] instant updates in list without refresh
I have a working example of a frontend form adding a record to a database, and a list under the form displaying all the results.
In the current repo of t3, the post display on the front page only displays the latest post, not a list of posts and i do not see any invalidate logic to mimic.
At first, i had to refresh the page and app to get the latest record to show on the screen. Then i added a cache invalidation which seems to update the list automatically, but its a little glitchy so I am trying to confirm if I am doing this correctly.
Info in video:
https://www.loom.com/share/ebf9c606938c4025890e16a44e911191?sid=eb53301c-2b36-4a61-94c0-11a5d1c2d37e
2 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 11/10/2023 in #questions
[How To?] Generate uuids properly on cloudflare d1 sqlite...
I am running an app with Drizzle, TRPC, and Cloudflare D1 sqlite database:
My initial project databasse schema looks like:
I am trying to add a uuid column to the table in addition to autoincrementing the id column and making the other columns not required. First I am trying to ad a uuid column.
I have researched this in a couple places, cant seem to find what I am looking for. I want a uuid column that automatically generates a uuid when i insert a record. Seems to be possible with mysql but not sqlite?
Here is the uuid column I have been able to push through drizzle to the database:
I have been able to generate the migration (
drizzle-kit generate:sqlite --schema=./src/db/schema.ts --out=./migrations
)
This gets pushed to the database via wrangler with a wrangler d1 migrations apply production --local
command.3 replies
DTDrizzle Team
•Created by Trader Launchpad on 11/10/2023 in #help
[How To?] Generate uuids properly on cloudflare d1 sqlite...
I am running an app with Drizzle, TRPC, and Cloudflare D1 sqlite database:
My initial project databasse schema looks like:
I am trying to add a uuid column to the table in addition to autoincrementing the id column and making the other columns not required. First I am trying to ad a uuid column.
I have researched this in a couple places, cant seem to find what I am looking for. I want a uuid column that automatically generates a uuid when i insert a record. Seems to be possible with mysql but not sqlite?
Here is the uuid column I have been able to push through drizzle to the database:
I have been able to generate the migration (
drizzle-kit generate:sqlite --schema=./src/db/schema.ts --out=./migrations
)
This gets pushed to the database via wrangler with a wrangler d1 migrations apply production --local
command.4 replies
[How To?] Create a record in database on form submission...
I have an application using trpc, Cloudfare D1 database, and trpc.
I am trying to use a tamagui form component to insert a record into the database.
My trpc route:
My drizzle table schema:
My form component:
When i click the form button, i get a 500 internal error on api/trpc side. Can someone tell me what I am doing wrong, i feel like its obvious but im not getting it.
5 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 11/1/2023 in #questions
[Create-t3-turbo] Issues deploying fresh clone on windows...
https://www.loom.com/share/2891b0907a264e319f5204a912cc9d3d?sid=a8d8541b-1a89-49e7-8b57-31051321889e
I am creating a new t3 turbo repo. This morning I cloned it on my windows desktop,setup .env, did db:push and pnpm dev. I was met with an error:
I deleted, tried with the npx turbo method, same issue.
I then switched to my macbook, and was able to get a local dev running via npx and git clone, both worked.
I pushed the repo to github from my mac, recloned it back to windows, and am getting the same error on deployment.
On windows, changing the line :
on the main app page.tsx (line 10) lets the app load on windows.
All deployments work in production in vercel. I just cannot get the vanilla repo to work on windows.
References:
https://github.com/vercel/next.js/issues/53562
2 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 10/31/2023 in #questions
[Nextauth] How to use middleware with grouped routes?
I have an application where admin pages are in a grouped route /app/(admin)/dashboard rather than /app/admin/dashboard.
Is there a way for the nextauth middleware matcher to protect grouped routes?
8 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 10/28/2023 in #questions
[How To?] App Router : Whats the most efficient way to structure this layout?
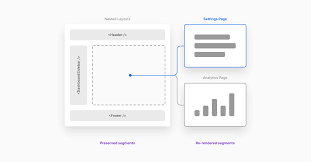
4 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 10/9/2023 in #questions
T3 Turbo : Error: Cannot find module 'autoprefixer'
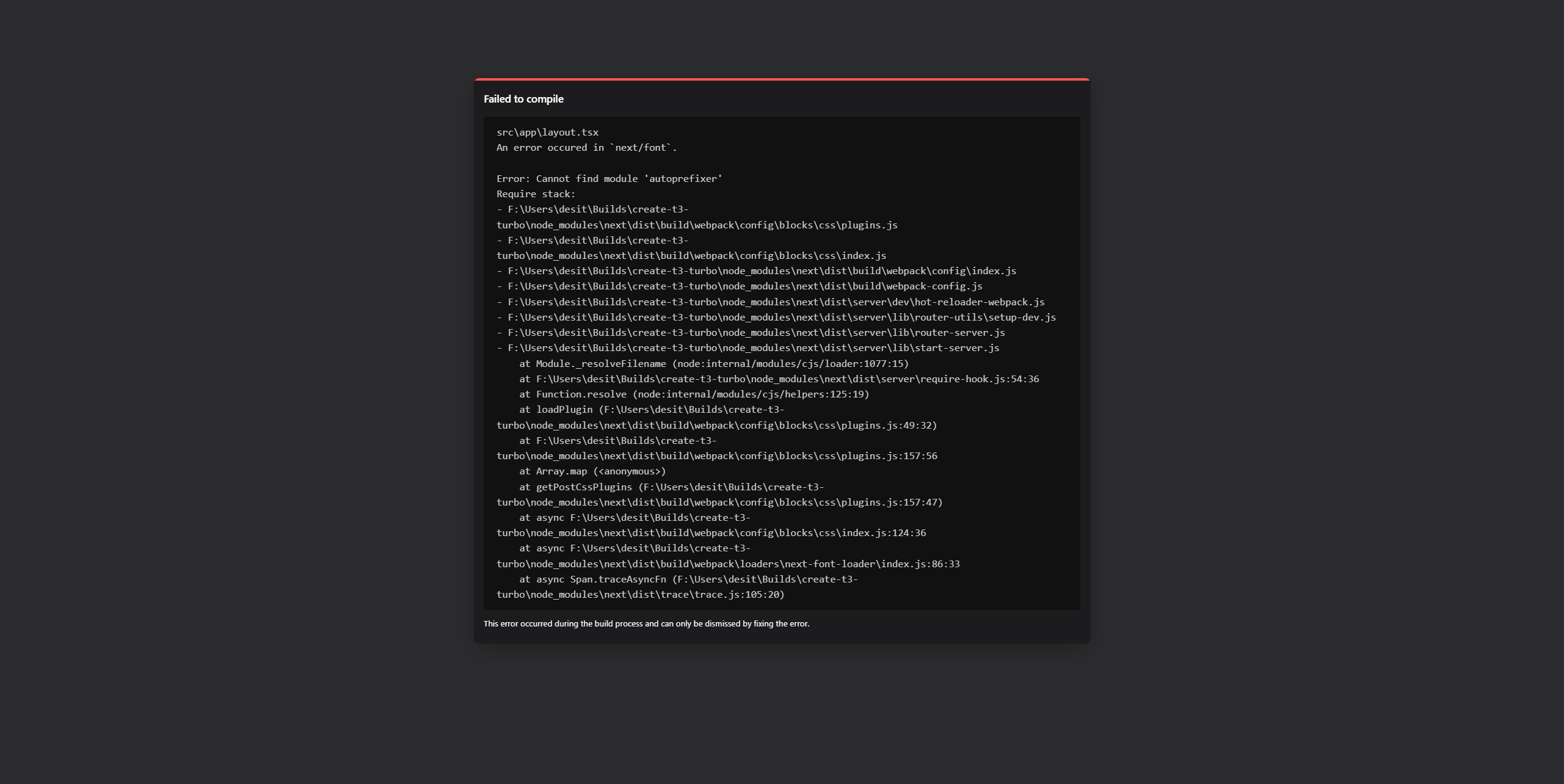
2 replies
TTCTheo's Typesafe Cult
•Created by Trader Launchpad on 5/22/2023 in #questions
[TRPC or Prisma or React Native issue?] Do not know how to serialize a BigInt
I og started a thread on the TRPC discord but am also posting here to see if anyone else has a better solution for this issue. I am trying to figure out exactly where this issue originated from.
OG link: https://discord.com/channels/867764511159091230/1110257286707937380/1110257286707937380
I am calling a trpc query in a component based on a currently selected item in a flatlist, and getting error:
9 replies
[How To] Properly use trpc UseQuery based on currently selected item
I have a component with a video element and a flatlist. I want to utilize a trpc query to get the videoUri based on the currently selected item.
Here is my working component:
55 replies
[HOW TO?] Call trpc endpoints from vanilla nextJs api routes
Spinoff from an og thread here:
https://discordapp.com/channels/867764511159091230/1032301198990135347
HI! I am wanting to do the same thing...call a trpc route from within a nextjs public api endpoint.
I am using a fresh t3-turbo-with-clerk project which has trpc in packages/api (@acme/api)
here is my apps/nextjs/src/pages/api/post.ts file
28 replies