Difference between NextAuth + firebase adapter, NextAuth + drizzle adapter, and just using firebase?
I have three t3 templates scaffolded.
One with Nextauth + firebase adapter.
One with Nextauth + drizzle adapter -> Planetscale
One with just Firebase (next-firebase-edge)
I am trying to understand the differences between these three and why I would go with one vs the other.
With NextAuth + drizzle, all auth and storage of the auth tokens is handled within my app and stored on my own server. Firebase services are not used at all.
With NextAuth + firebase adapter, the auth logic is handled in my app but the records are stored in Firebase. No users show in the Auth section of the Firebase UI console.
With just firebase installed (standard client only or next-firebase edge (allows ssr)) all auth logic is handled by Firebase, and I see no storage of auth tokens its stored by Firebase and I have no ownership. Users do show in the Auth section of the Firebase UI console.
Now lets say I want to add an expo app in a t3 turbo monorepo:
With NextAuth + drizzle, I understand that I would have to write my own logic to mimic what Nextauth does inside my webapp for auth.
With NextAuth + firebase adapter, its possible for me to sign in the user in to Firebase with a firebase customToken during the Nextauth auth flow. The user now shows in the Firebase Auth UI console. The user seems to have 2 sets of auth cookies now. One for nextauth and one for Firebase. Is this a bad practice? Could i now use the Firebase auth logic in my expo app (it works with react-native) even though I am using Nextauth for most of the logic in my webapp?
With just firebase, I conceptually understand that I can use the firebase packages in my webapp and mobile app to handle auth.
What is the best route?
4 Replies
The best route depends on what you wanna own.
I'm kinda bias here because i don't really like to use NextAuth, but the first two case scenarios you give up parts of the App Auth Logic to NextAuth, and keep the database records. In your mobile app, you'll have or to mimic the NextAuth to keep the database tables the same, or create another logic creating more tables.
The third one you give up all the logic to firebase. The advantage here i think is that on the mobile app you can just use the firebase mobile version(if it exists, i don't really know) and don't worry about compatibility or modifying anything.
The best routes depends on what you wanna depend , what you're willing to do compatibility wise, and how important these steps are for you project.
Between these 3, i would go with the NextAuth + Drizzle, since it seems to me it's the one you would have more control of.
But considering that you will make a Mobile App, isn't it better if you own auth logic and use the same one across services?
@Matheus Lasserre
Lets say I use Nextauth + drizzle adapter (planetscale) and during the nextauth callback I generate a Firebase custom token and save it into the nextauth session:
and then wrap my app in a FirebaseAuthProvider that logs the user in with Firebase:
Then i use trpc and drizzle in my nextjs app to fetch and query manipulate data from my planetscale database. Their is account, user, session, and verificationToken tables in my planetscale database. There is also a user showing up in the Firebase Auth UI, with a uid that is the same as their UID in my planetscale database user table.
What is the implications if I now wanted to add an EXPO app? Would I use expo/react-native firebase package to sign in the user into Firebase only using custom token? Then use the Firebase UID returned to query the planetscale database from within my expo app? Would i need to interact with the nextauth database tables at all?
If i did this, I have cookies for both nextauth and firebase in the nextjs app.
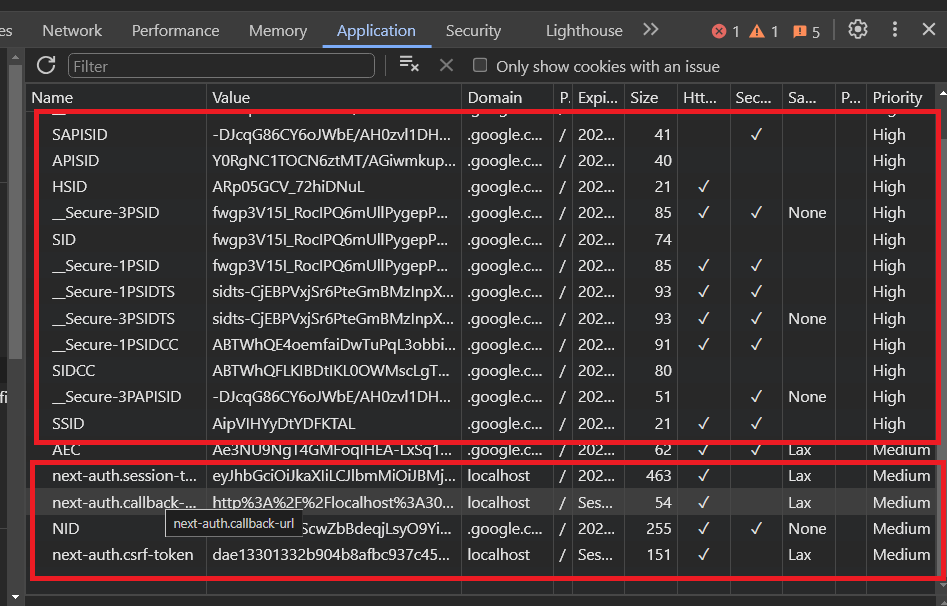
I don't really understand why would you want to have the user authenticated in both your app and on firebase. Wouldn't just one be enough?