Steadhaven
Explore posts from serversJCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 1/25/2025 in #java-help
Extend/Inherit java class - how to?
I have a repo with some kind of config in the style of a YAML file... As an example its something like this:
I wanted to extend it to deal with different system:
Its just an example, doesn't make sense necessarily.
Now in Java, in another repo, I want to account for this indentation/nested change...
How do I correct myself now? A lot of code and test use BirdProperty, but now theres two "systems" of them and I need to somehow also indent/nest my java code with some inheritentance or something.
Keep in mind the above Java and YAML code is probably wrong in itself, but this is sort of what the code and yaml change was from memory.
26 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 9/14/2024 in #java-help
Custom Spring Boot actuator HealthIndicator
I am reading a book that explains how to create a custom HealthIndicator in Spring Boot, but I don't find it satisfactory. It is calling an external API, and immediately just returns the health status as either up or down based on that.
Often, you need to check for the health of very specific components and services in your application. Or even specific code snippets running somewhere, that other applications may depend on. Furthermore, this can sometimes take a certain time to run, so the health status ought to be "down" while the specific internal code is still running and then return "up" when its up.
TL;DR: How can I create a custom HealthIndicator for specific code parts in my Spring Boot application? It has to be able to check more than once, e.g. if docker calls on the health end point it ought to check if its done yet or not. (my own scenario is that my app is doing some liquibase migration at its startup, and other docker services should wait for it to finish before they start theirs).
6 replies
Mmfad
•Created by Steadhaven on 8/31/2024 in #questions-and-advice
shoes for blue shorts/white t shirt
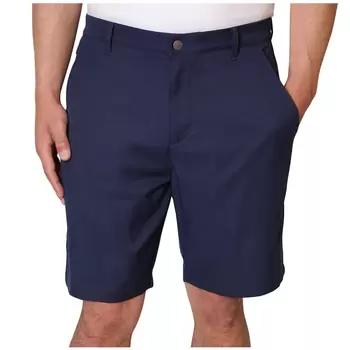
3 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 8/13/2024 in #java-help
Resources to learn and apply input/output streams?
Where can I learn about the practical application of input/output streams - especially in the form of consuming/producing HTTP requests (rather than local filesystem)? Things like consuming a HTTP request on a href to a download link, and pass it as a ByteArrayInputStream to Apache POI and make an excel file out of it.
Preferably in a easy to understand "ELI5" form. I don't care if its a book, video, article, or course... Whatever makes me get it properly and able to use it in production code would make me happy :)
Context: I am working as a tester, and have to test the project we are working on. But its a very complex application running in Docker, as well as online CI/CD with Jenkins and so on. I found that using HTTP request and input/output streams is the better way of handling data for testing functionality.
8 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 8/4/2024 in #java-help
Capture download data directly from browser to give to Apache POI workbook?
Hi,
I want to figure out how I "capture"/"get" the bytes of the inputstream when you press on a download link in the browser. Reason is that for my work, we have an internal tool that generates excel files, and I want to test that the generated file is an excel file. However, since we use docker etc, its easier to just capture the bytes/stream of data that comes when pressing the download link and give that to the following method:
Workbook workbook = WorkbookFactory.create(new ByteArrayInputStream(response.contentAsByteArray))
The above method is Apache POI workbook, it will create an excel file and if the data is not excel file, then it will throw an exception.
TL;DR: How do I get the response.contentAsByteArray
from a link? With selenium its easy to click on the link, but I don't know how to pass that along to the workbook?21 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 7/27/2024 in #java-help
How to easily edit XML file content in Groovy/Grails?
Hi,
How can I edit the content of an XML file using Groovy/Grails?
I need to be able to edit/add/delete some lines in an XML file that a method generates. The method is made by someone at my work and produces a specific kind of XML file with prefilled content. However, I needed to change certain parts of it to fit my needs, and I was suggested to manually edit it in this case.
How can I edit the XML file? We are using Groovy/Grails at work, so it has to be with that. Also, it would be nice if the edited XML file could be easily passed along in the code, to do other things with that instead of the original XML file.
If it's any further help, then I am doing some End-to-end testing on it using Cucumber/Selenium, but probably not relevant.
4 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 6/30/2024 in #java-help
Cucumber fails to recognize steps definition (Intellij, Java Maven)
I created a java maven project (java v22) in intellij with junit, cucumber-junit, cucumber-java, cucumber-picocontainer, and selenium-java maven dependencies (all cucumber from io.cucumber v. 7.18.0,and selenium is 4.22.0, while junit is 4.13.2).
I created a "features" folder in the root directory, and inside there a file called Ebay_Home.feature with the content:
And then in ./src/test/java, I created a directory called "steps" with java file called EbayHome_Steps.java with the content:
The content (printing something) doesnt matter, but my problem is when I right click
Ebay_Home.feature
file and click Run 'Feature: Ebay_Home'
in intellij, I get an error about that there are no steps defined...
Anyone know how to fix this? Its a bare project - totally new that I just created these two files in.9 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 6/7/2024 in #java-help
How to figure out the proper variable type of fx a selenium webdriver method?
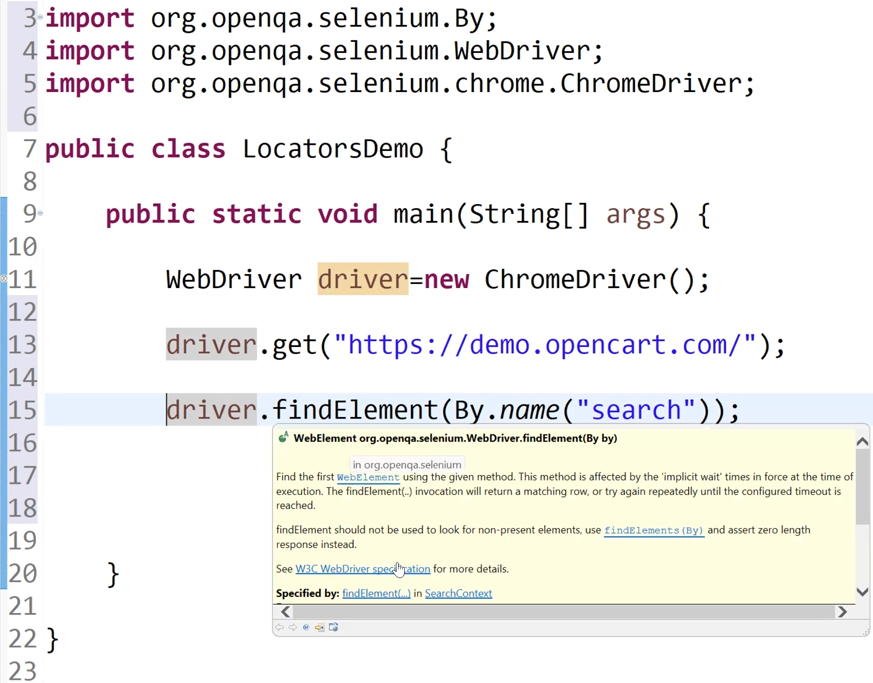
4 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/28/2024 in #java-help
How to learn Groovy? (fundamentals, incl.scripting and unit testing)
Hi,
I got a job as a technical tester, and they use Groovy for certain things at that job. I want to be prepared and learn about it for a smoother start at my job.
Besides the official docs, which I don't really feel is that explanatory, are there any up to do date way to learn Groovy properly?
I need to learn the basic of it, such as syntax, variables, operators, and control structures.
But I also want to learn the more advanced parts of Groovy such as the collections, functions, closures, and Groovy scripting.
Lastly, the most important thing is understand unit tests and integration tests with Groovy.
8 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/22/2024 in #java-help
Logic test help for a job interview

16 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/19/2024 in #java-help
Understanding the three steps of object declaration, creation, and assignment (easy)
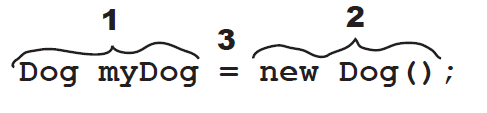
50 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/18/2024 in #java-help
ArrayList vs LinkedList sorting speed?
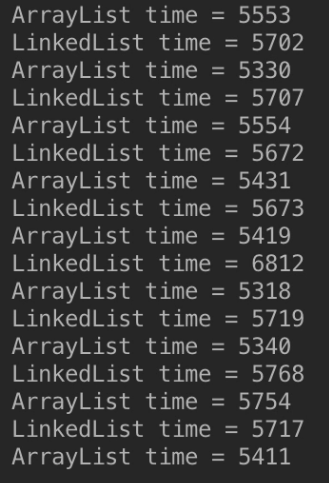
22 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/17/2024 in #java-help
CopyOnWriteArrayList iterator behaviour?
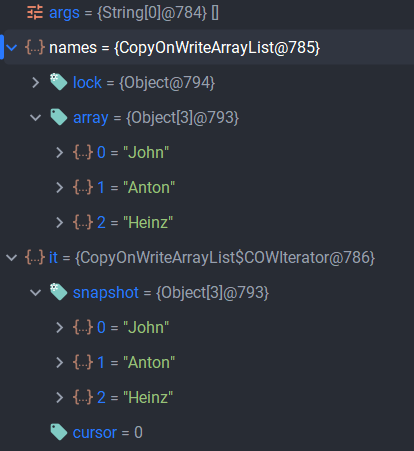
4 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/17/2024 in #java-help
System.err shows up before System.out in terminal?
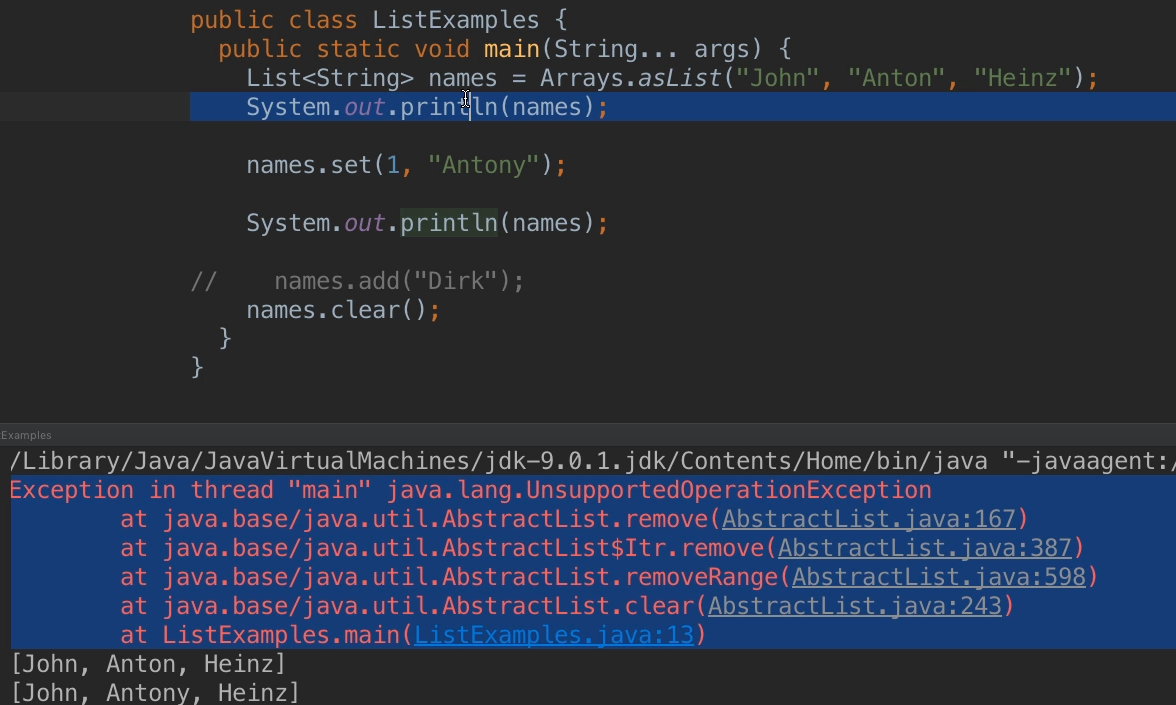
23 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/17/2024 in #java-help
Cannot resolve symbol 'println'
I got this error when I try to print:
Cannot resolve symbol 'println'
What? I never seen that error before. Its a simple print statement?6 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/16/2024 in #java-help
Is this a good learning path to follow?
https://javaspecialists.teachable.com/p/data-structures
This course goes through
java.util.**
and teaches them all. I was just curious whether the topic list he has is a good thing to go through? Wise way to spend time learniing something new?
(Its way too expensive, so its not about whether the course is worth buying, because I wont, but I want to use the list to learn)15 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/16/2024 in #java-help
Indexing in JPA to ensure a good performance when searching for yearly data?
Hi,
I am trying to figure out if my indexing makes sense:
I need to be able to quickly lookup yearly payments that a given company have received for a given year.
4 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/13/2024 in #java-help
Translating basic UML diagram into Java code?
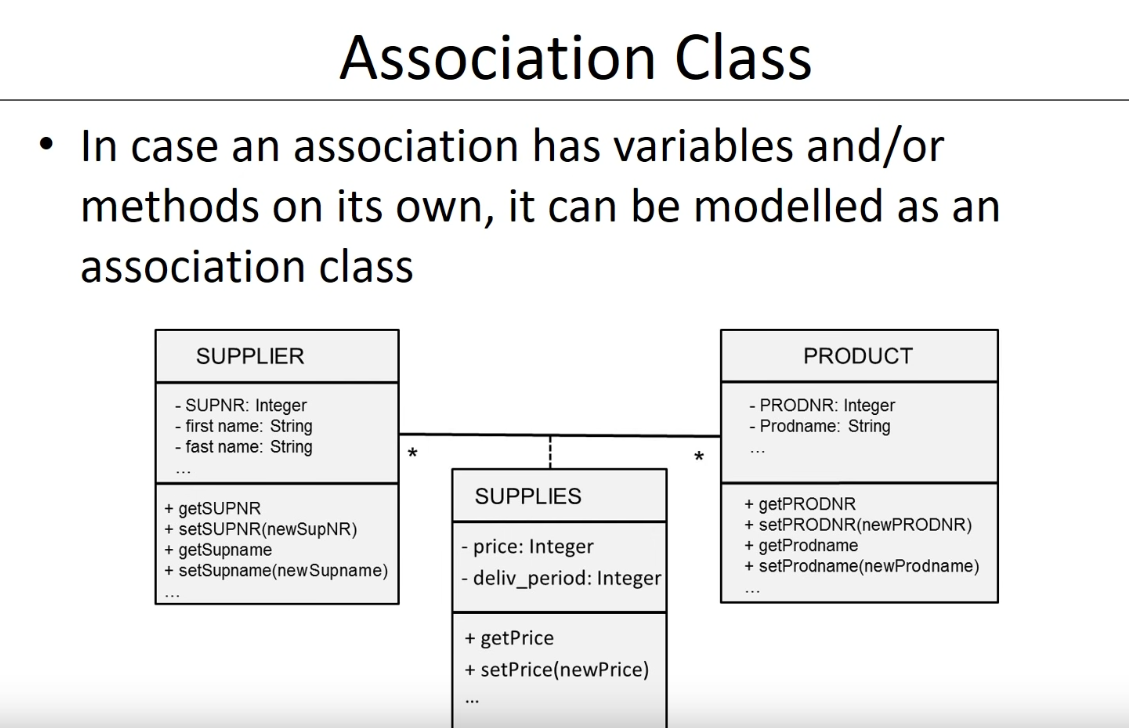
8 replies
JCHJava Community | Help. Code. Learn.
•Created by Steadhaven on 5/7/2024 in #java-help
JUnit's Assertions.assertThrows first argument?
Hi,
I am trying to understand the first argument
Assertions.assertThrows
takes.
I know it has to be the expected exception that will be thrown, and in this case we expect it to be RuntimeException
.
I am confused as to what the .class
means? I know what a class is but never seen it used with a dot after some object.58 replies