Capture download data directly from browser to give to Apache POI workbook?
Hi,
I want to figure out how I "capture"/"get" the bytes of the inputstream when you press on a download link in the browser. Reason is that for my work, we have an internal tool that generates excel files, and I want to test that the generated file is an excel file. However, since we use docker etc, its easier to just capture the bytes/stream of data that comes when pressing the download link and give that to the following method:
Workbook workbook = WorkbookFactory.create(new ByteArrayInputStream(response.contentAsByteArray))
The above method is Apache POI workbook, it will create an excel file and if the data is not excel file, then it will throw an exception.
TL;DR: How do I get the response.contentAsByteArray
from a link? With selenium its easy to click on the link, but I don't know how to pass that along to the workbook?13 Replies
⌛
This post has been reserved for your question.
Hey @Steadhaven! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
what error do you get?
I didn't get further. The workbook line is from some other part of our code base.
My main confusion is how to get the stream of data that comes when you click on the download link. I want to pass that response to the workbook, and see if it accepts it as an excel file. I don't care about the content further than that, except if its a proper excel file or not.
I don't have access to the file system (that would be easy, just give workbook the file) since its like a docker container which runs both locally and in git/jenkins CI/CD.
Maybe HTTPBuilder from Groovy is a solution?
this can probably be done in java, give me a sec i will try and see
will be great
I have been stuck with this work task for too long
pretty hard to understand I/O, especially when its not local files on my own system
like how would one capture/"intercept" the data from the download link
should be just simple get call
This was chatgpts try with groovys RESTClient
this works
oh nice, so the httprequest only needs the link to the download? e.g. say I want to download the small file of the test file website: https://www.thinkbroadband.com/download
Would I then just pass it the appropriate url: http://ipv4.download.thinkbroadband.com/20MB.zip
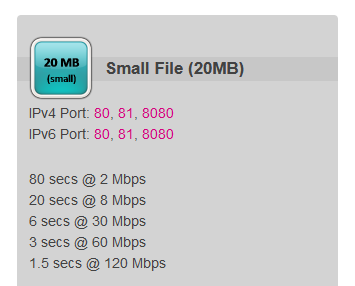
yes
thanks so much, I was stuck with this for weeks
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
Post Closed
This post has been closed by <@305362010374406144>.