Erky
Explore posts from serversJCHJava Community | Help. Code. Learn.
•Created by Erky on 2/23/2025 in #java-help
How to sign executable jar with jsign or similar
So I have a game I want to share with a user who does not have Java.
Im using launch4j to convert my .jar to .exe.
I want to get rid of the antivirus warning.
I found out I can do this by signing the application.
I found out about Jsign for this.
Is this a good idea?
43 replies
How to overwrite a file that is used by another process?
I know which process is locking it, its a .net host instance, but I still want to overwrite my .log file (i want to clear it, when i press a button).
I keep getting
System.IO.IOException: The process cannot access the file
10 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/20/2023 in #java-help
In game loop, reset index to 0, go through 4 animations, then do something
can someone help me to show* all 4 images of the dying animation before setting to gameover*? it works for setting game over in the player class but when I try to set game over when walking on a trap (lava), it only shows the first animationindex and I don't know how to correctly show all images of dying and then set game over
Player
ObjectManager:
4 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/16/2023 in #java-help
How to increase jump height when holding space
I want the jump height to be depending on how long the player holds space. My question is how I can modify the airSpeed depending on the jumpTime (the time that player has held down space). Currently it's not adjusting jumpTime properly and I don't see how I can fix it. Here is my current implementation:
4 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/16/2023 in #java-help
Disable autojump in game
is there a way to not allow autojump when holding space? i don't want autojump.
problem is that when he lands, there is a split frame where inAir is false, so he is allowed to jump. it looks kinda buggy because you can autojump when standing still, or moving constant one direction, but not if you switch direction. here is the relevant code:
5 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/14/2023 in #java-help
Run jar file with JRE
I can't get to run a .jar file with JRE only. it only works with JDK 20
Exception in thread "main" java.lang.UnsupportedClassVersionError: misc/GameRunner has been compiled by a more recent version of the Java Runtime (class file version 63.0), this version of the Java Runtime only recognizes class file versions up to 52.0
I want to build a .exe with JRE bundled in but I can't even get JRE to run my application which was developed in JDK 2011 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/10/2023 in #java-help
Not hearing sound in IntelliJ IDEA
simply not hearing anything, the audio file works fine and the program runs without issues but for some reason cannot hear anything
14 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/8/2023 in #java-help
Login/register 308 error
I have a web app + database hosted in python which I have tested and works for creating, logging in and logging out user. Now I'm trying to make the requests in my android application written in java. However, I keep getting 308 error.
Here is my RegisterActivity.java
and this is the most important part of GsonRequest which should parse JSON into string:
Any help is appreciated!
55 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/4/2023 in #java-help
Collision with slopes in a game
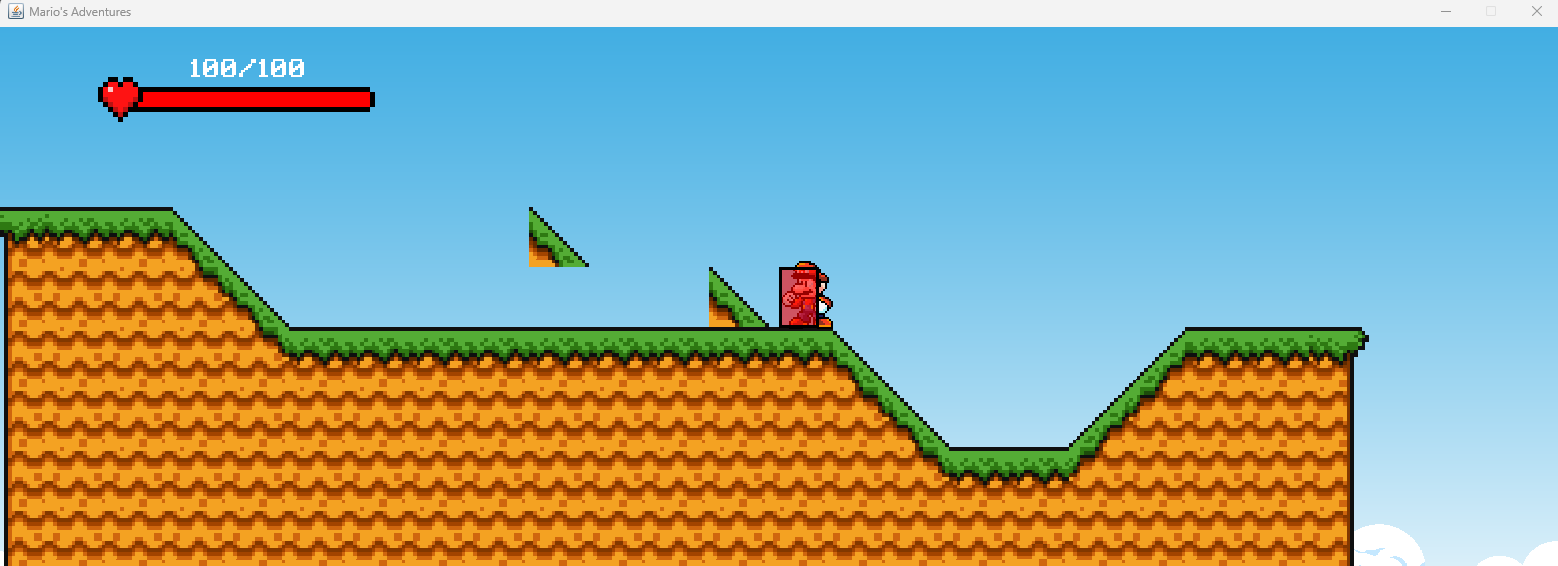
11 replies
JCHJava Community | Help. Code. Learn.
•Created by Erky on 4/3/2023 in #java-help
Java game slopes tile collision
I'm trying to figure out how I can make my player move on sloped tiles. My tiles all represent RGB values, and this is how I currently handle collisions and whether the player can move there or not:
And here I check if the tile is solid, and if so, the player can move to position and I update his hitbox.x (which is the top left position of the hitbox).
My question is, how can I adjust the player hitbox y position based on what section of a sloped tile he is standing on? I have the section of the tile he is standing on but I don't know how to update the "solidity" of a sloped tile.
92 replies