Java game slopes tile collision
I'm trying to figure out how I can make my player move on sloped tiles. My tiles all represent RGB values, and this is how I currently handle collisions and whether the player can move there or not:
And here I check if the tile is solid, and if so, the player can move to position and I update his hitbox.x (which is the top left position of the hitbox).
My question is, how can I adjust the player hitbox y position based on what section of a sloped tile he is standing on? I have the section of the tile he is standing on but I don't know how to update the "solidity" of a sloped tile.
68 Replies
⌛
This post has been reserved for your question.
Hey @Erky! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when you're finished. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
@Fischstaebchen im trying to focus on simply setting the hitbox to be at the appropriate height when passing a sector, i can calculate slope angles when i first get this to work. just having trouble with the overall code approach, like
how can i update the hitbox.y position to be at the right height? because when setting it like this, he just keeps bouncing because he thinks he is in the air. i have a method isEntityOnGround that checks if the bottom left and bottom right of the hitbox is on the ground, and if so, moves the hitbox down to the ground. so i need to add a checker before that somehow
so if i disable falling mechanics, it looks decent when moving fast, but when moving slow i slowly float up to the top tile for some reason?
so this one checks if im in the air or not
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I mean, In my game I only have two types of slopes which is diagonal from top left to top right or vice versa
the slope is just an image, which i have defined as non-solid so i can walk through it
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
walk through the slope from the right side
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
so I try to adjust the hitbox according to what section of the tile its standing on. if in section 4 (the leftmost) the tile is almost solid at the top, so make a small change to the hitbox, if in section 1, the tile is nearly transparent and hitbox should almost be on the floor
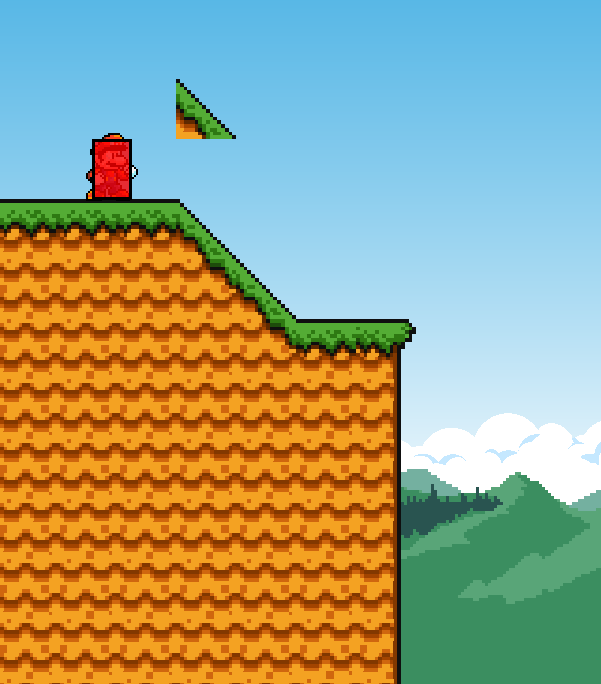
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeah thats the problem, this isnt a good solution i dont think. thats why it clashes with the falling mechanics which also sets the hitbox.y
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
what do you mean define the slope as a hitbox? how would i do that?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
https://discord.com/channels/648956210850299986/1092511181215899779/1092543191405822032
so i draw the map with rgb values and I define if they are solid or not in the
this is their RGB value which is how i can check a specific tile on the map
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
no, the map is loaded via a png

each pixel represents a tile
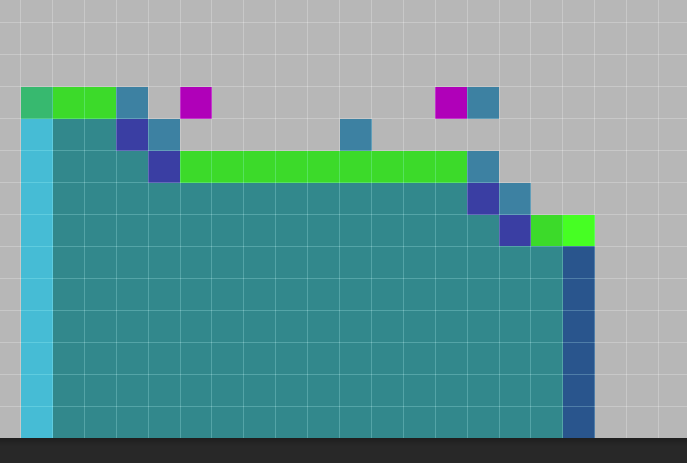
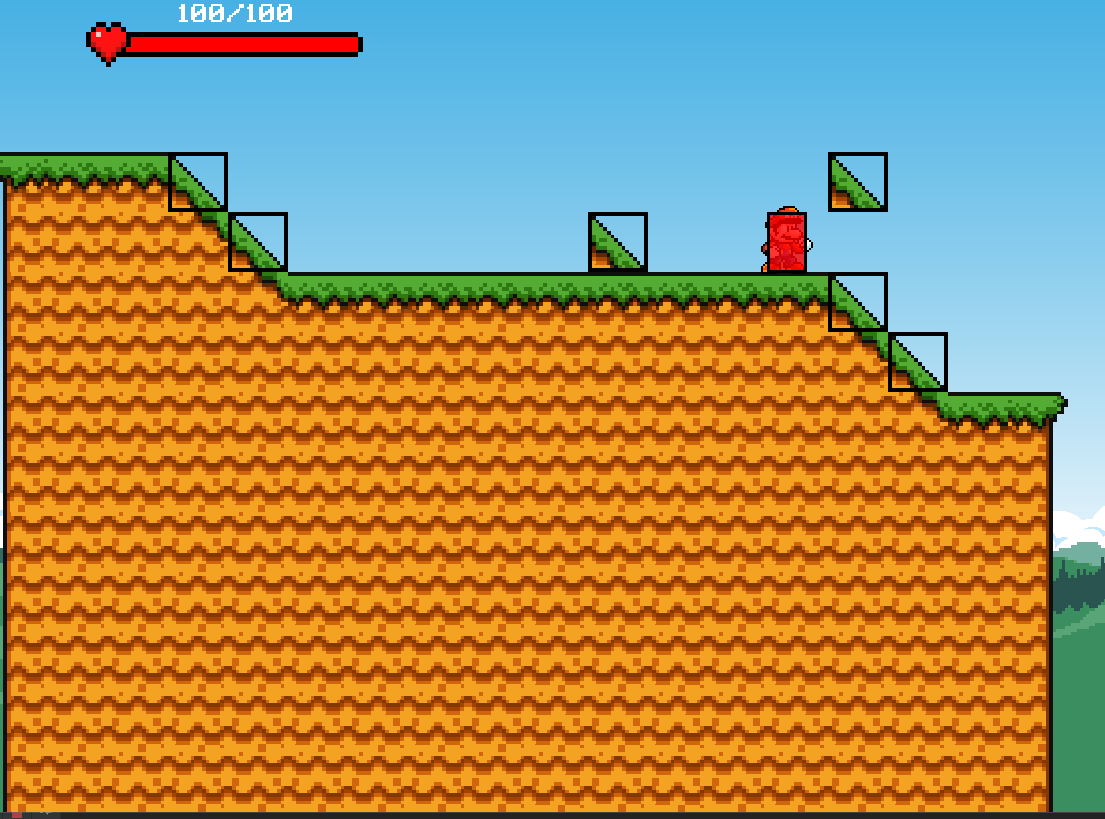
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeah
so how should i approach this
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeah , so how can I adjust the player hitbox then based on the coordinates inside the tile?
should I send over the github?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
thanks
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
just really wanna get these sloped tiles working it would be really awesome 😄
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
40
public static final int TILES_SIZE_DEFAULT = 40;
public static final float SCALE = 1.5f;
public static final int TILES_SIZE = (int) (TILES_SIZE_DEFAULT * SCALE);
put then i scale it a little bit, but TILES_SIZE is the value I use to get the tile position
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yes
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeahh i guess
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
what do you mean make sure that its correct?
where do i go from here
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
i think the most logical step would be to update the slope position directly in here, so i check if the tile is sloped and in that case i can determine the behavior in updateslopeposition. because here i can access the hitbox all corners and then i can define how the slope looks and whatnot
and then i return that the tile is solid after that, so i should be able to fall through it if coming from the top or the right side
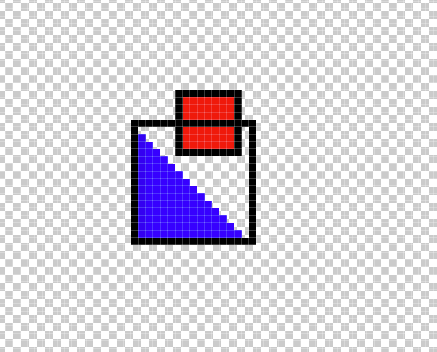
so if im coming from the top and falling down through it, i want to keep falling through and then depending on the position of the slope, set the hitbox.y
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yes thats true, can i add a checker there, so that it exits and doesnt go further if im in a SLOPED_TILE like this:
if tileValue == SLOPED_TILE
return
only problem is i dont know how to get the tileValue inside my entityInAir method, been stuck on that for a while
feels like i have everything i need with my section solution where i divide it into 4 sections, later i can optimize this surely, but i just want to set the hitbox.y inside here but i dont know why it isnt working as expected
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
wait whaat x == y?
i feel like continuing with my solution, just need to set hitbox.y here somehow , thats my main question
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
do you know how to set hitbox.y correctly and avoid making the player fall? i have this variable falling that i could adjust also
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
moveToPosition
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
y
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
because in the arguments, i check if they can move to the next x position, or the next y position
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
ok 👍
appreciate your help 🙂
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
oh ok thanks! will keep looking myself, good night 🙂
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived. If your question was not answered yet, feel free to re-open this post or create a new one.
still working on this
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived. If your question was not answered yet, feel free to re-open this post or create a new one.
Post Closed
This post has been closed by <@272473391041347585>.