Nicolas
Explore posts from serversCan't get fetch to work in server code
Hi all,
I have a simple form and action like so:
And my function being called is like so:
But for some reason when i try to submit the form and run this code, it only gets to right before the
fetch
since it console logs About to fetch...
but it never logs the response. There is no error being thrown, or anything at all. I checked the browser console and my server logs (showing About to fetch...
), but nothing shows after that.
What could be going wrong?6 replies
DTDrizzle Team
•Created by Nicolas on 8/11/2024 in #help
SQL string could not be parsed: non-terminated block comment at ...
Hi all,
I am using Turso and I added two properties to my users table
created_at
and updated_at
.
I successfully generated a migration file, which looks like this:
This is my schema:
When running the migration, i get the following error:
Any idea what's going on? This is a super simple migration, just adding 2 columns, so not sure what to do...
Thanks6 replies
CDCloudflare Developers
•Created by Nicolas on 3/16/2024 in #pages-help
How to Interact with Service Workers from Pages Functions
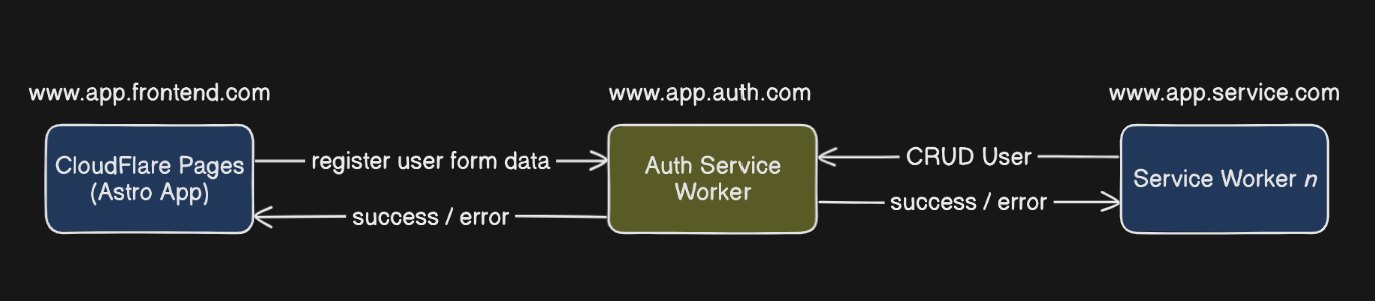
2 replies
No "mutation"-procedure on path
Hello all,
I am using the latest version of TRPC on my client and server. I am using React Query on my client and AWS Lambda on my server side.
When trying to perform a test mutation:
I get the following error
Error TRPCClientError: No "mutation"-procedure on path "insert_superUser"
I am using a Meta
to make sure only users of a specified role can call certain functions, like so:
3 replies
DTDrizzle Team
•Created by Nicolas on 2/14/2024 in #help
Getting error NeonDbError when pushing migration file
Hi all,
So i have a schema that defines custom type JSON objects for my NeonDB database, like so:
And I am trying to use it like so:
However, I am getting an error
NeonDbError: data type json has no default operator class for access method "btree"
So perhaps I am not specifying the custom type of json
correctly, but I could not find any examples on the drizzle website.
I also tried like (commads instead of semi-colons):
But it still lead to the same error.
Any thoughts on how to do this properly?
Thank you!4 replies
RRefine
•Created by foreign-sapphire on 2/4/2024 in #ask-any-question
Error Creating New Refine App
Hello all,
I am running into errors trying to start a new refine app.
Here is what I did:
npm create refine-app@latest
When running npm run dev
I get this error:
I am not sure what depends on graphql, so i don't want to remove it.
Seeing as this is just a simple refine app created from the official CLI, I am assuming this should work out of the box, but I may be wrong based on my configuration.
Thanks!5 replies
How to Incorporate MinIO with Windmill Docker Compose
Hi all,
I am new to Windmill and docker in general, and I am trying to incorporate MiniO so I can do file uploads for images and other files, however, I cannot get this to work with the docker-compose provided by Windmill and the official docker-compose provided by MiniO here: https://hub.docker.com/r/bitnami/minio
Attached is my docker-compose.
I am getting the error:
All other containers are launching just fine, but the
windmill-windmill_server_1
errors out with the above error and keeps restarting.
Am I not assigning the proper linkage to MiniO to the correct service in the docker-compose?
Any guidance on this would be great, not sure if I am thinking of this properly.
Thank you!6 replies
Creating inner context for AWS Lambda Context Options
Hi All,
I have been using tRPC for many routes, and I have started to realize I need to call certain routes on the server-side (lambda / serverless in my case), and so I was trying to follow the documentation on how to create an inner context / outer context so I can call my tRPC routes from other routes, but I am stuck and I am confused on how to go about doing it for AWS lambda context as the documentation example is rather simple.
This is what I have so far:
5 replies
DTDrizzle Team
•Created by Nicolas on 10/11/2023 in #help
Infer Types from Partial Select Prepared Statement

5 replies
DTDrizzle Team
•Created by Nicolas on 10/9/2023 in #help
Null fields not working as expected
Hi all,
I am using Drizzle ORM with Zod validation for useForm, and I am running into issues with fields that are allowed to be undefined / null, but I get Zod errors stating that the value of the optional fields (when left empty) are
invalid_type
and in required.
I was able to fix this by refining the field as follows:
This allows the city
field to be left blank, but by default, even though i didn't specify notNull()
on that field, Zod would error out saying that the field is required.
I am using Turso DB, so this is my schema:
Notice for all fields by the id
, organizationId
, and name
, they are optional; however, Zod treats them as ZodNullable
but they should in fact be treated as ZodOptional<ZodNullable<ZodString>>
to allow my fields to be empty (as is the intended affect).2 replies
Basic Inference not Working
I have been struggling to get basic type inferencing to work with trpc, react, and Drizzle ORM.
This is what my server-side router is returning from the
list
method:
Where, if I hover over the response
in the return, it says the type is:
However, on my client side, it says the type is any
for the following:
Why is this basic type inferencing not happening?
I am using Drizzle ORM to infer the return type on the router response; could this have something to do with it?
Does trpc not work well with ORM's that use inference?4 replies
DTDrizzle Team
•Created by Nicolas on 9/30/2023 in #help
notNull() is still letting field be optional
I am performing the following for SQLite:
However, when I perform:
export type NewProduct = typeof products.$inferInsert;
It says that id
is optional (this is what it shows when i hover over NewProduct
)
How can I fix this?8 replies
DTDrizzle Team
•Created by Nicolas on 9/30/2023 in #help
Explanation on execute() vs all() vs get() vs run()
I am using
prepare()
to make prepared statements for Turso (so SQLite), and the docs use prepare.all()
for SQLite instead of prepare.execute()
as is used for PostgreSQL and MySQL, why is that?
Same goes for prepare.get()
for SQLite instead of prepare.execute()
When do I know when to run those different queries for SQLite?
Thank you!1 replies
DTDrizzle Team
•Created by Nicolas on 9/29/2023 in #help
Issue Pushing Schema to Turso
Hi All,
I made a schema.ts with
sqliteTable
statements, and I created a drizzle.config.ts
as follows:
And when I try running pnpm drizzle-kit push:sqlite
, I get the following error regarding that it cannot find module better-sqlite3:
Am I not pushing the schema properly with the command drizzle-kit push:sqlite
?
Thank you!3 replies
DTDrizzle Team
•Created by Nicolas on 9/27/2023 in #help
How to make sql.placeholder() type safe
I have the following prepared statement:
However, when I use this like below:
const response = await listDeployments.execute({ orgId: input });
The orgId
is not type safe, I can enter whatever string I want, and there is no way to protect this call from receiving something other than orgId
Is there a way to make it such that the caller must pass in orgId
?
Thank you!4 replies
DTDrizzle Team
•Created by Nicolas on 9/23/2023 in #help
Neon and Drizzle ORM: Can my schema.ts create my tables in Neon?
As the title suggests, I was wondering if Drizzle ORM can create my tables in Neon from my schema.ts, or if I need to first create my tables in Neon with SQL, and then just create my schema.ts to reflect those tables.
Thank you!
7 replies
RRefine
•Created by old-apricot on 8/28/2023 in #ask-any-question
Add Type to useForm
How do I make the object that I am manipulating, with the help of useForm hook, type safe?
For instance, I know I can do things like:
But how do i ensure
email
is type-safe with respect to some interface? For instance, I have a user
interface that I would like to apply to my useForm, so that way I make sure I only perform the proper useForm actions on the fields that exist for user
5 replies
RRefine
•Created by absent-sapphire on 8/22/2023 in #ask-any-question
MUI Inferencer Can't Detect Object Inside Entity
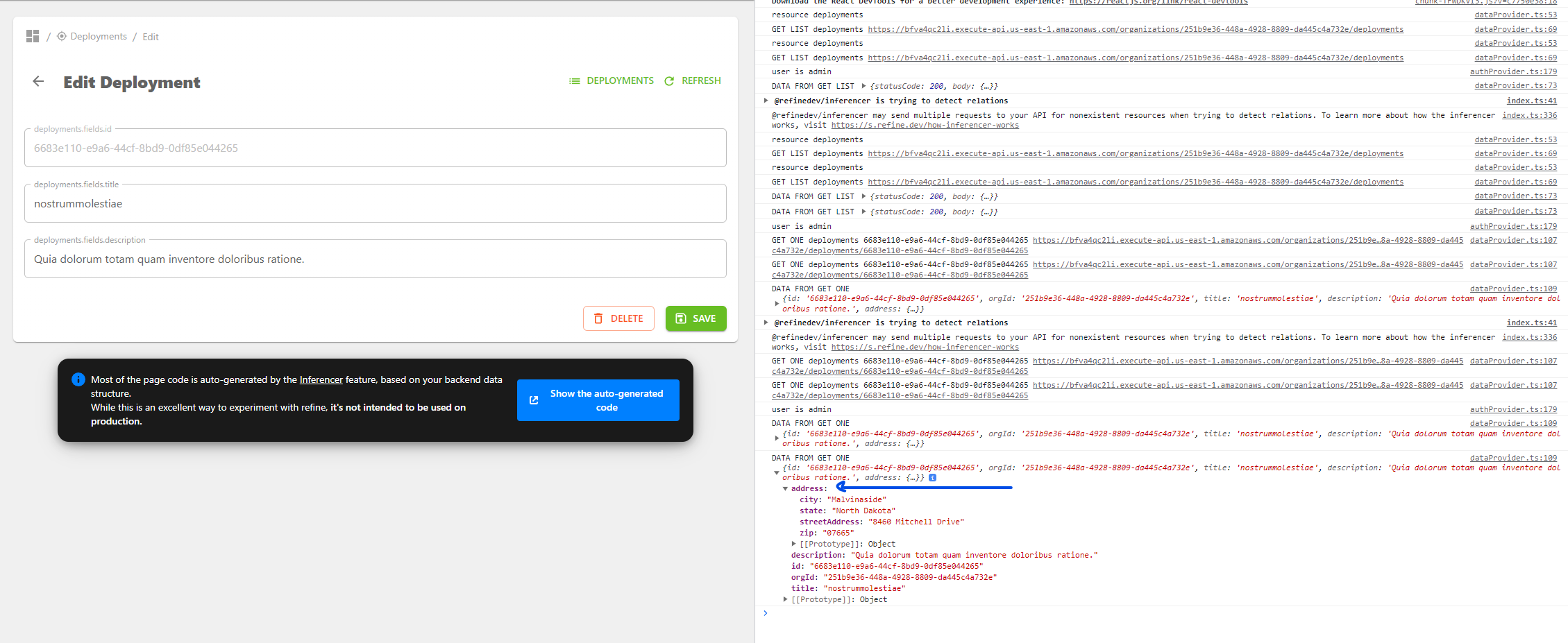
5 replies