kinsyu
Explore posts from serverscreateTRPCReact cannot be named without a reference to x
Hey, I have a monorepo where I have a separate package for trpc (@repo/api) which I'm trying to use on the frontend.
When trying to create
createTRPCReact
I'm getting the following type error
Here's my code on the react app
And here's the code in the api package
9 replies
DTDrizzle Team
•Created by kinsyu on 5/25/2024 in #help
onConflictDoUpdate excluded with a dynamic column
Hey, I'm trying to perform an insert where it's possible that the record already exists, therefore I'm using
onConflictDoUpdate
, the problem I'm running into is that I can't dynamically set the column to update, here's my current approach:
I'm getting the following error when executing this
4 replies
TTCTheo's Typesafe Cult
•Created by kinsyu on 4/30/2024 in #questions
T3 Turbo - Run a typescript app with node
I'm using create-t3-turbo starter, only the tooling and the web app side of things. Within this turbo repo I'm trying to run a simple typescript app, I can't seem to figure out how to run it with the existing tsconfigs. All the examples I've seen either use
expo start
, next start
or nitro
.5 replies
DTDrizzle Team
•Created by kinsyu on 12/1/2023 in #help
There's not enough information to infer relation
I am trying to use drizzle studio but I'm running into the following issue,
There is not enough information to infer relation "__public__.collectionsTable.tokens"
.
Here's a simplified version of the schema.
In short, each collection can have multiple tokens, but each token can belong to only one collection.
I haven't had any issues with this schema for around the 6 months that we've been using it, but we wanted to try out drizzle studio and ran into that issue. The database is running on Planetscale, not sure if that's relevant.3 replies
TTCTheo's Typesafe Cult
•Created by kinsyu on 5/21/2023 in #questions
tRPC Middleware consuming memory limit on Vercel
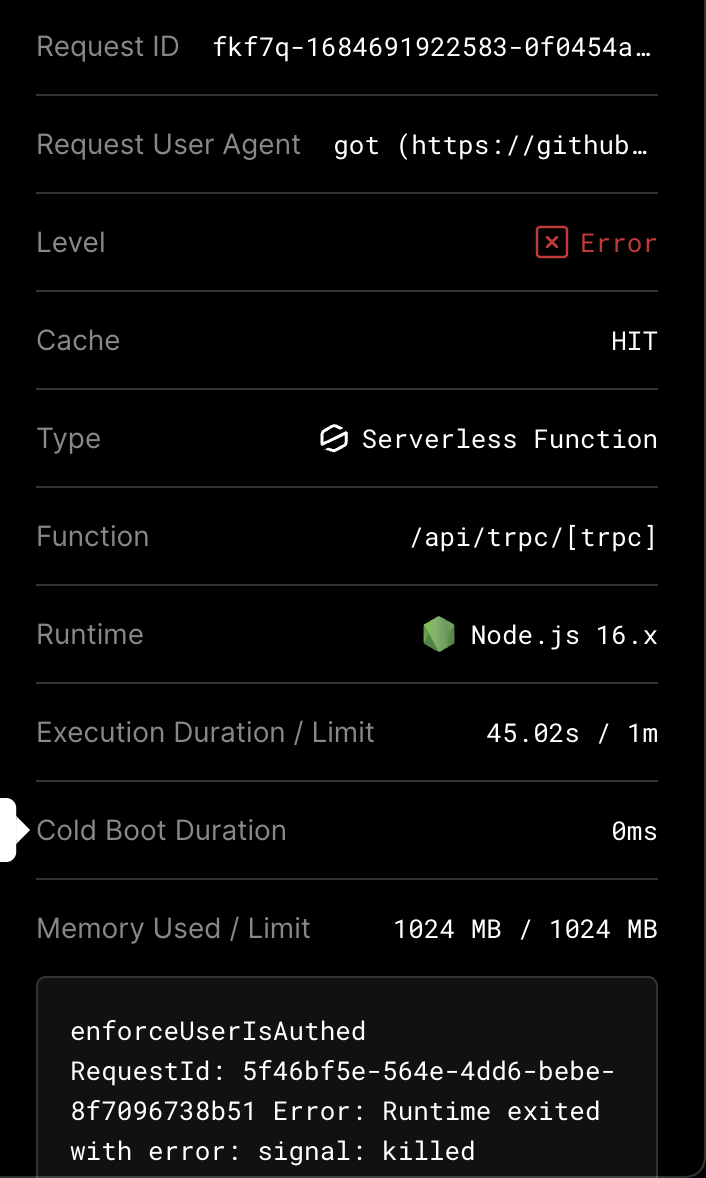
2 replies
TTCTheo's Typesafe Cult
•Created by kinsyu on 5/12/2023 in #questions
Refresh a single React Server Componetns
I have a dashboard where I want a certain piece of data to update every 10 seconds, what's the best way to do this with Server Components? The only approach I've found is to use
startTransition
and router.refresh()
inside a useEffect
with an interval.12 replies
TTCTheo's Typesafe Cult
•Created by kinsyu on 4/26/2023 in #questions
Change return type based on value of parameters
I'm trying to change the return type of a function based on the value of the arguments passed to the function itself. I'm struggling to find the right documentation for it, could anyone point me in the right direction
Here's an example of what I want:
4 replies
TTCTheo's Typesafe Cult
•Created by kinsyu on 4/20/2023 in #questions
Jotai Bug?
Hey, anyone here that has worked with Jotai and is willing to lend me a quick hand about a bug I'm running into? been trying to debug this for 10h and I just don't understand.
I have a simple set up that consists of three parts.
1.- API Calls to fetch an array of data
2.- atom with query that calls the data
3.- a derived atom that spreads the array and joins it with some default data ( for the sake of this example, we'll only use the data returned from the api)
This is the code:
The issue is the following when checking in the
fetchTokens
function, the console log doesn't find any data, but when checking in the allTokensListAtom
function, it does find a result. I have absolutely no idea how this is happening.
The atoms are not being set anywhere, only being read.2 replies