vince
Explore posts from serversKPCKevin Powell - Community
•Created by vince on 4/15/2025 in #resources
Short PDF On Pricing Client Projects (Breaking the Time Barrier)
https://www.freshbooks.com/fbstaticprod-uploads/public-website-assets/other/Breaking-the-Time-Barrier.pdf
Useful information about how to price your freelance projects to clients. Touches on value-based pricing. Helped me a lot on how I 'should' price (though it's not always that easy in reality 😂) and gives you a new perspective on things. Worth to read, takes maybe 15-30 minutes!
1 replies
KPCKevin Powell - Community
•Created by vince on 4/14/2025 in #help
When to use `span` over `p`?
I feel like I've gone full circle 😂
Semantically,
p
is for... paragraphs.
So if I just have a sentence of text, should that be within a span
? Would you still put it in p
?23 replies
KPCKevin Powell - Community
•Created by vince on 4/13/2025 in #help
Hosting a PHP-based CMS on Digital Ocean
Any idea how to set up a CraftCMS site in Digital Ocean? It's a PHP-based CMS and I think they use MariaDB. Never did any hosting more complicated than Netlify and I have some experience with cPanel but that's about it, and I can't find any good articles specific for CraftCMS. Would appreciate some guidance!
199 replies
KPCKevin Powell - Community
•Created by vince on 4/9/2025 in #help
How would you BEM this?
ChatGPT is showing me:
But that doesn't look right to me. I suck at BEM but I always thought that
-
denoted the modifier.
I thought it should be something like this maybe?
9 replies
KPCKevin Powell - Community
•Created by vince on 4/1/2025 in #help
Some fun beginner projects we could do as a server
I'm interested in some ideas for beginner-ish project ideas people in here could team up with. Anyone have ideas? I'm open to anything
14 replies
KPCKevin Powell - Community
•Created by vince on 4/1/2025 in #front-end
Ideas on how to position input placeholder

61 replies
KPCKevin Powell - Community
•Created by vince on 3/13/2025 in #front-end
Parallax / floating effect
I'm trying to make a really simple floating effect where a foreground element moves at a different speed than the background. I understand I can use background-attachment: fixed for a parallax effect but I don't really want that; I just want the foreground elements to move at a different speed (if they have a .parallax class).
https://codepen.io/vince1444/pen/bNGYwBa
I can't seem to get this to work, and all the resources out there are honestly pretty bad; I can't seem to find anything that works well.
An example that comes to mind is Gumroad's old homepage (though I doubt anyone remembers that). I went to see how they implemented it again but realized they changed their homepage. I vaguely remember them using transform3d and perspective, and just changed the translateY on scroll but I don't remember exactly
5 replies
KPCKevin Powell - Community
•Created by vince on 3/3/2025 in #front-end
Confused by useEffect in React
I'm confused on how useEffect actually works. I know useEffect is used to trigger some sort of 'side effect' after the changes are committed to the browser. I'm creating a typewriter effect for an example here and I'm confused on the dependency array of useEffect. Obviously, whatever you pass to the dependency array is what will call the useEffect again if said value changes / updates. But why does this typewriter effect not work if I remove
index
from the dependency array?
If I remove it from the dependency array, it'll print out the first letter countless times. It's not actually updating the index state and instead using the old value. My understanding of useEffect is that as long as it reruns it'll use the updated state values in the component, even if it's not in the dependency array. This doesn't seem to be the case. So if any value is needed in useEffect I must pass it to the dependency array if I need its updated values after rerenders?
Example:
18 replies
KPCKevin Powell - Community
•Created by vince on 2/18/2025 in #os-and-tools
Advice on setting up a monorepo
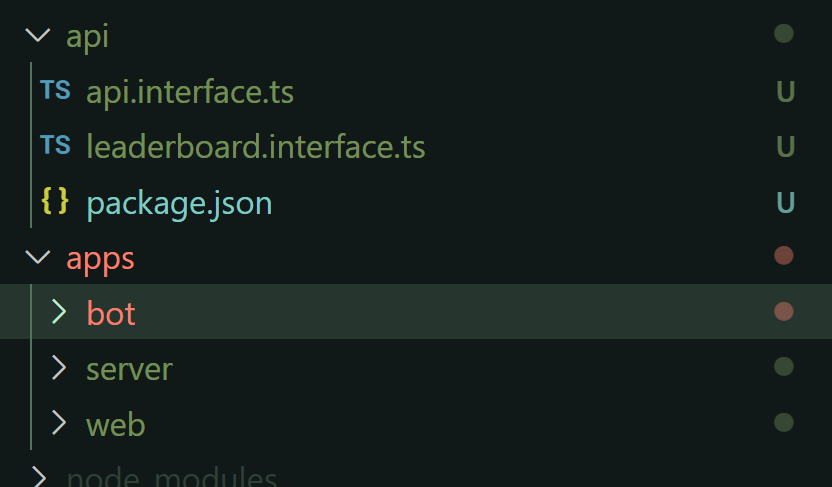
3 replies
KPCKevin Powell - Community
•Created by vince on 2/11/2025 in #back-end
Quick question about date and string types
I have a date property (e.g:
createdAt
) from the backend. I'm sending it to the frontend as JSON data. Since JSON only works with strings, what's a good way to handle this as my TypeScript definitions are Date
s. Should I rewrite my types to take Date | string
or should I make some type of parsing function?
I feel like if I make it a Date | string
, it'll be a bit ambiguous. I have multiple functions in the frontend that handle the createdAt
as a Date
, no parsing method needed. But when I get the createdAt from the backend, I'll need to know it's from the backend and parse it as a date in the frontend. Does that make sense? I'm trying to word it but I guess what I'm trying to get at is it might not be 'standardized' when working with it in the frontend. Typing that out, I realize what I could potentially do is rework my frontend methods that use createdAt to get the data from the backend, now that I actually have it setup, that way it'll be in a standardized format53 replies
KPCKevin Powell - Community
•Created by vince on 2/10/2025 in #os-and-tools
Testing iOS simulation in React Native?
I read about iOS simulation for building apps in React Native and it seems impossible to simulate iOS apps without macOS? Surely can't be the case right? Does anyone know of some workarounds so I can develop and test on Windows?
69 replies
KPCKevin Powell - Community
•Created by vince on 2/1/2025 in #front-end
TypeScript type error
Hi guys! I have a type error where I'm at a loss on how to proceed.
I'm writing a discord bot that fetches messages in a channel, filters them, and then transforms the data for my backend models.
Please see an example of the filter function:
I'm filtering for 3 things:
1) if the message is a text message,
2) if the message is written by a user,
3) if the message contains a specific reaction
Here is the
getMessageReaction()
method:
As you can see, I'm checking to see if the message contains a specific reaction via filter
and returning null if not.
The issue is when I go to mutate my data.12 replies
DIAdiscord.js - Imagine an app
•Created by vince on 1/29/2025 in #djs-questions
Fetch all messages in a text channel
Hey there! I'm trying to fetch all messages in a channel for my
/initialize
command. I'm looking at the faq example:
I think I need to modify the while conditional. The amount of messages in the channel is dynamic, so I can't base it off that. I don't want to manually copy the id of the oldest message either. I was thinking about approaching it by timestamp or by checking if there's a previous message before running it again. Are there any examples of this / suggestions? Thanks!25 replies
KPCKevin Powell - Community
•Created by vince on 11/1/2024 in #front-end
Sass Mixin vs Extends
I had a quick question I wanted to ask regarding this.
I have some files in a shared directory that I want to namespace.
I want
_forms.scss
to have reusable styling that I can use in other classes around the codebase. I don't need any argument features that mixin supports - I really just need it to work like extends. The problem is, I can't namespace extends. So if I try to do:
This doesn't work. I can however do this with mixins:
The problem is, this is leading to single line mixins:
My question is, is this a totally valid use case, or am I in the complete wrong direction and I should quit as a webdev? 😂110 replies
KPCKevin Powell - Community
•Created by vince on 10/31/2024 in #os-and-tools
How to use Webpack as a way to compile Sass -> Css?
This is probably a stupid question, but I have been trying this for the past 2 hours and can't figure it out. I just need a way to compile my regular .scss files (in every directory) into 1 .css file. I'm aware I can just use
sass --watch
, but I'd like to have some other features webpack leverages (like minifying, and again I know sass ootb can do this).
We use Laravel Mix, which is just a library on top of Webpack that can do this using mix.sass('entry.scss', 'output.scss')
, but I can't get it to work on default Webpack. The reason why I want to go from Mix -> Webpack is because I need to watch all files in my project and output it as 1 css file and Mix can't do this16 replies
KPCKevin Powell - Community
•Created by vince on 10/30/2024 in #front-end
Variable font-weight shenanigans

2 replies
KPCKevin Powell - Community
•Created by vince on 10/29/2024 in #os-and-tools
How do I install npm & composer on cPanel?
Having trouble finding a clear tutorial on how to do this, and whether this should be installed globally as the root or per cPanel user. Running Linux and I have access to the Yum package manager, so should I just install per-user using yum?
50 replies
DIAdiscord.js - Imagine an app
•Created by vince on 10/26/2024 in #djs-questions
Quick question on managers
I just wanted to ask a quick question on managers (specifically a
GuildMessageManager
): how do I use this? Is it a class I need to instantiate or what?7 replies
KPCKevin Powell - Community
•Created by vince on 10/24/2024 in #front-end
Should I future-proof this component or leave as designer intended?

8 replies
KPCKevin Powell - Community
•Created by vince on 10/21/2024 in #back-end
How to build this db model
Working on a NoSQL implementation of this discord bot me and my friend are setting up. What it'll do is iterate through all messages and get the amount of emojis each user has across all their messages.
I was wondering what a good model for this would be? Should we build a model for each User, and then iterate through all their emojis? I have this so far:
This way, we can just do something like:
17 replies