rubberburger
Explore posts from serversTTCTheo's Typesafe Cult
•Created by rubberburger on 3/30/2025 in #questions
Updating a different table based on changes from a settings table
How does one implement this in a way that won't crash a db?
For example, a different table (T1) references a central settings(T2) table, and when that setting changes, ALL rows in T1 must update with that new setting
To make it more complicated, T1 has rows which use the setting for calculations, so those values will have to be recalculated as well
----
For context, i'm currently creating a payroll system for a small company using next, postgres
I currently implement the solution to what i mentioned by having a 'bulk regenerate calculations' button (for example for the employee timesheets). Only problem is, with just around 100 of those, supabase already cuts the connection/times out
what would be a better way to approach this?
3 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 3/9/2025 in #questions
Mac: Disable window resize when moving mouse pointer to right edge of screen
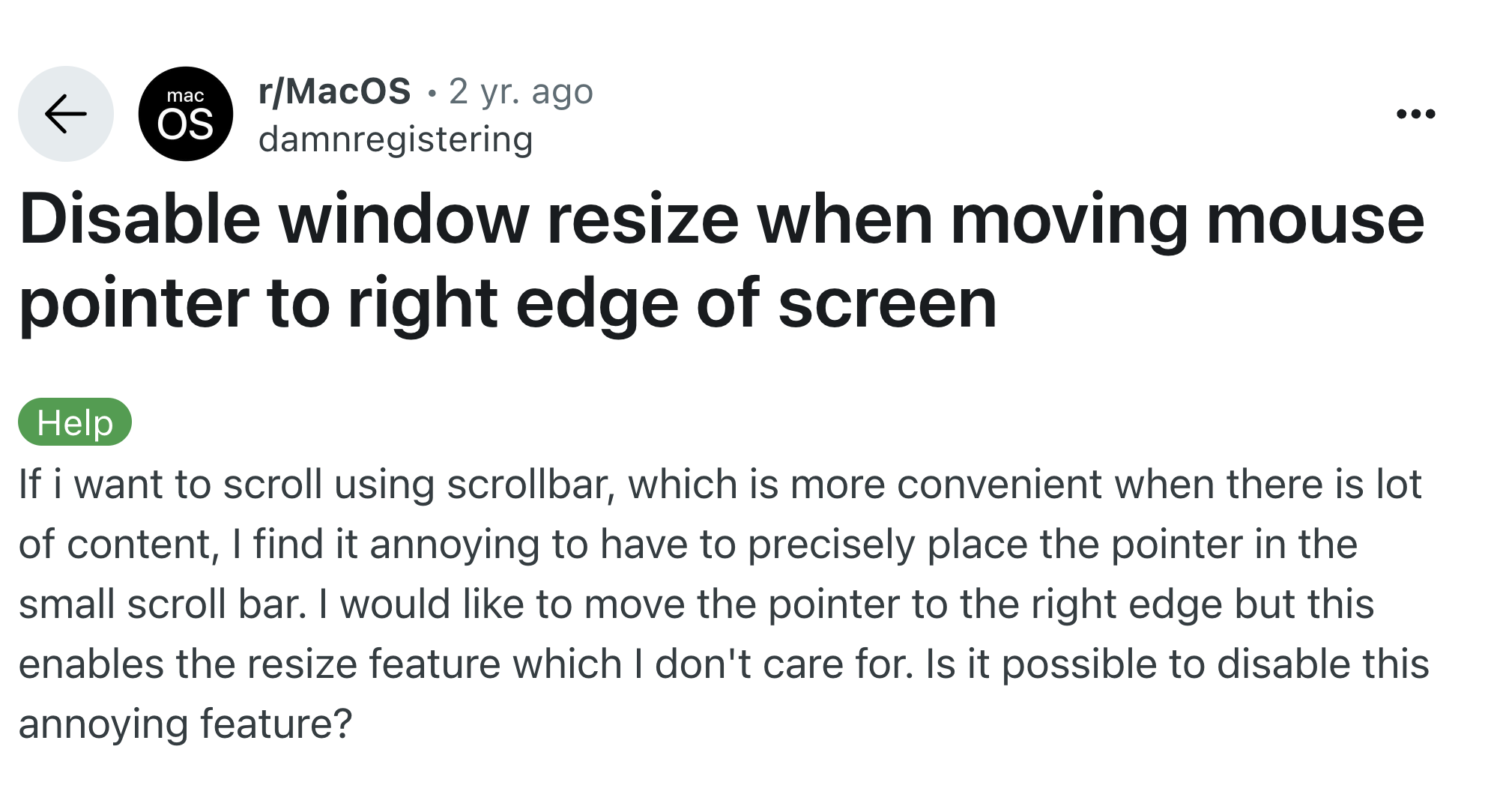
4 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 3/3/2025 in #questions
Looking for self-hosted metrics, monitoring suggestions
haven't really tried this before so i dont know much but can ya'll point me out to some guides, tech stack for these stuff?
I'm using next on the frontend, express on the backend, hosted on a VPS with coolify
I'd like to be able to check logs, see resource usage, etc
what's the best way of going about this?
btw, found that coolify can install grafana (which i haven't tried before), but i'd like to try installing it myself, which im not sure how to do
do i add it to my existing docker compose? or create a new compose file?
also curious about stuff like sentry, posthog/umami are they compatible with grafana and the likes, etc.
2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 2/15/2025 in #questions
Client-side Exception Occurred
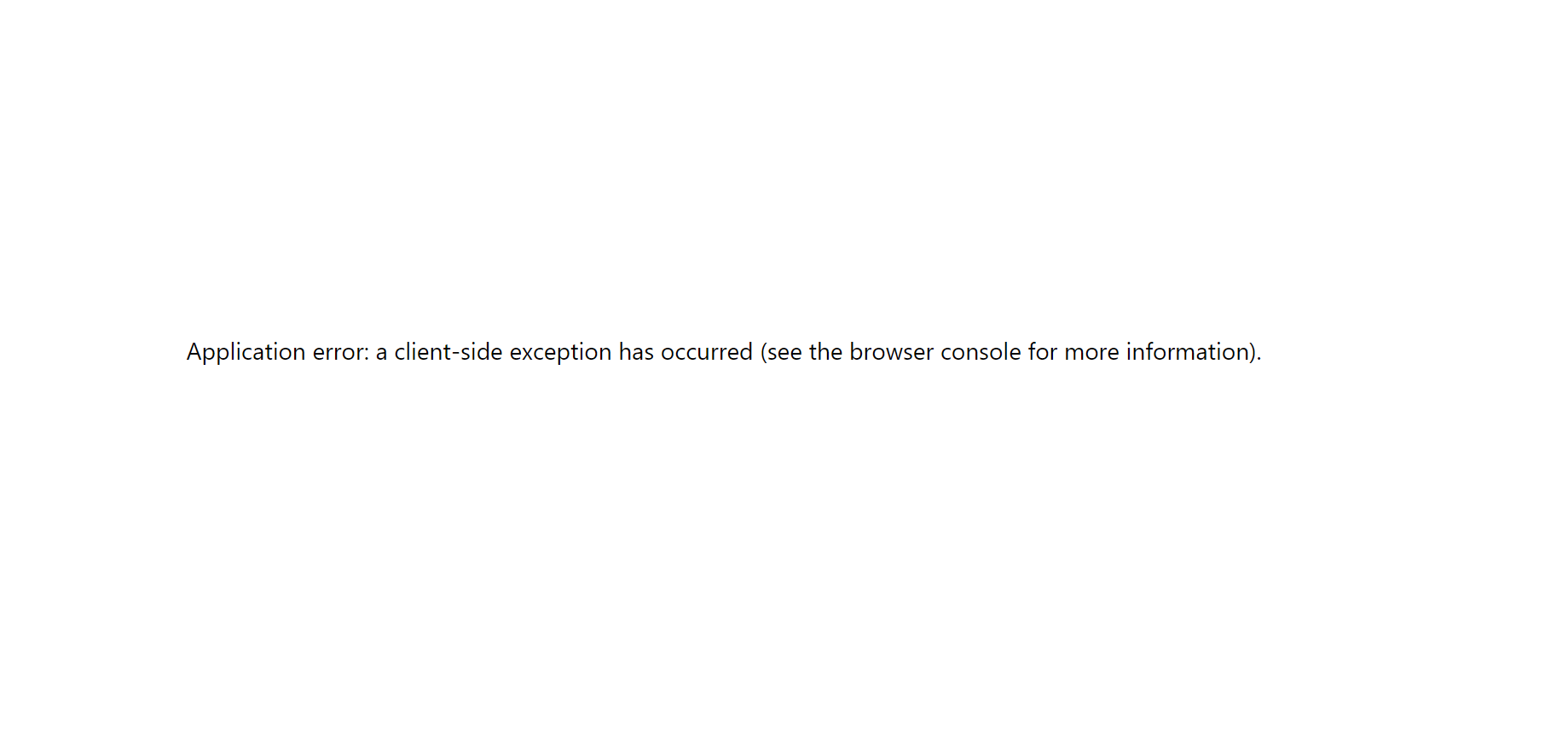
1 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 1/12/2025 in #questions
15in MBA vs 14in MBP
Currently on the lookout for a new laptop, and wondering if i should go for which one
any of ya'll have experience with both? tbh i'm a bit more inclinced to pick the MBA just for the bigger screen while still being portable
only thing that makes me second guess is that i live tropical country (so higher ambient temp), and i also do ML (although i'll prob outsource higher workloads to the cloud)
What do you think, which one should i go for?
---
Also, i'll prob go with 24gb of RAM (unless 16gb is enough for workloads that include docker, i don't think it is)
8 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 11/30/2024 in #questions
Coolify vs Dokku
Can i get some opinion on these two, i.e. which i should go for
For context:
- NextJS, React Native, Express Monorepo
- PostgresDB
I'm not that familiar with docker yet (i only know the basics), and afaik dockerizing my DB makes it stateless/isolated right? so backups, logging, etc are handled separately or something?
I'd also need
- ability to spin up a staging environment online
- maybe provision postgres and redis
- (in the future): horizontal scaling maybe (tho i'd prob just scale vertically until i can't)
- analytics and things like sentry, etc.
2 replies
CDCloudflare Developers
•Created by rubberburger on 10/2/2024 in #pages-help
Easiest way of persisting function logs
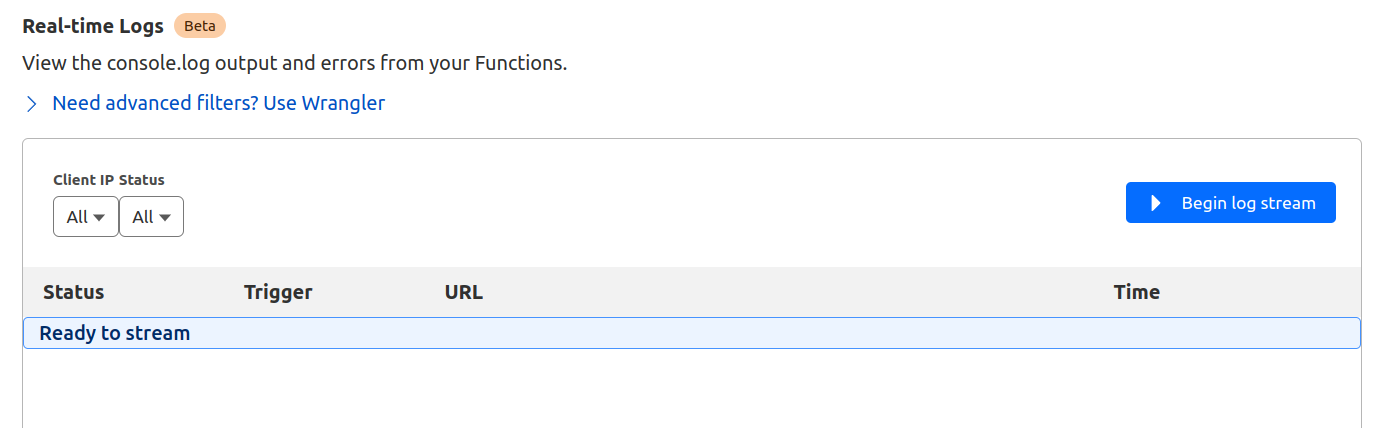
3 replies
CDCloudflare Developers
•Created by rubberburger on 9/28/2024 in #pages-help
How to point CF domain to CF pages project
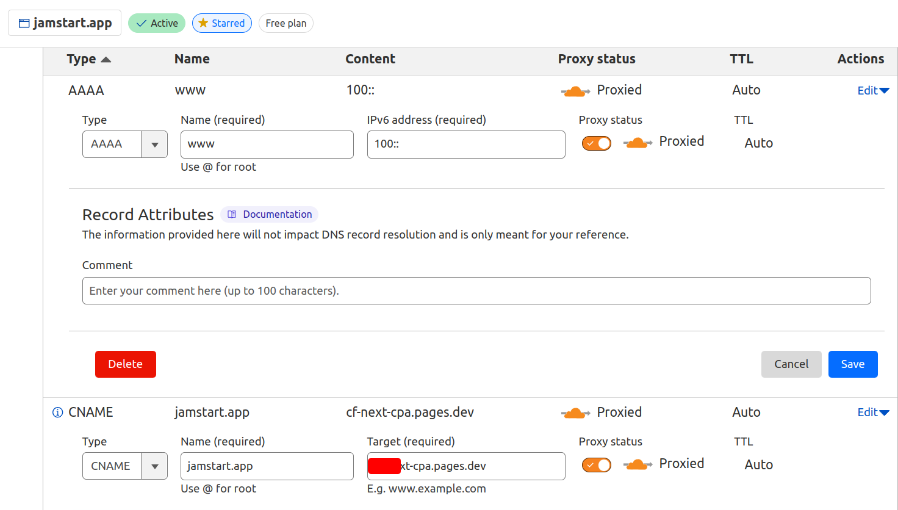
8 replies
CDCloudflare Developers
•Created by rubberburger on 8/8/2024 in #pages-help
ReferenceError: XMLHttpRequest is not defined on api endpoint
I'm deploying a NextJS project using next on pages. I'm getting a
"ReferenceError: XMLHttpRequest is not defined"
when trying to run the code block below. It sounds like the worker behind the pages is trying to use XMLHttpRequest
instead of the normal fetch? How do i fix this?
here's the dev branch of my site: https://dev.cf-next-cpa.pages.dev/
The endpoint in question is this:
https://dev.cf-next-cpa.pages.dev/api/spotify_auth/refresh_tokens
-----
The funny thing about this though is that my code has been running perfectly fine before this (for like 400+ commits/deploys) then all of a sudden it stopped working now.
I'm also using supabase but it doesn't seem to be the issue since the error only occurs exactly at the fetch
call.
Did something change with CF workers? The code works just fine locally btw ,also i use the pages
dir instead of the app
dir.18 replies
CDCloudflare Developers
•Created by rubberburger on 1/1/2024 in #general-help
Help with DNS resolution (using CF pages and CF domain name)
I currently have my site hosted using CF pages and bought a domain using CF's registrar,
it works fine and all for the most part where if i type domain.app, it displays the contents I have deployed using CF pages (i set this up using pages' "custom domains")
after i set this up tho, there was this notice on the dashboard saying something like i have to set up the A,AAAA and CNAME records such that
www.domain.app
would also redirect to domain.app
so i tried (as seen on the screenshot) but it didn't work
what'd i do wrong? (it's my first time dealing with domains so i'm pretty unfamiliar with these stuff)
btw, the CNAME record here is the record automatically set by CF after i linked the domain name to my pages project6 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 10/25/2023 in #questions
AI copilot/code intellgience
does anyone know of a code intelligence/AI copilot thing but with embeddings (i.e. takes your codebase as context), something kind of AI i can ask questions when i want to but takes into account my codebase as context (not AI autocomplate)
i know sourcegraph does this, but i remember seeing another program on YT but couldn't remember its name (it's not Kite/Copilot/Tabnine)
2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 9/19/2023 in #questions
Just a question about the current state of Next's app router
Almost every new video on YT rn uses the app router instead of pages.
I was initially hesitant to switch to app because of some caching issues, but a lot of libraries rn (like clerk and supabase) have been favoring app over pages.
I'm just wondering, have the caching issues been fixed? It's the only thing holding me back anyway.
2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 8/26/2023 in #questions
postgres(i.e. supabase) functions and triggers with drizzle ORM
i'm about to start leaning drizzle (can't use prisma because i deploy to a full-edge environment).
before i dive deep into drizzle though, i'm wondering, how does one handle/create functions triggers using drizzle? doesn't seem like something one would put inside the schema file.
couldn't find info on their docs either
2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 7/16/2023 in #questions
Paypal webhooks
I've successfully set up paypal subscriptions inside a nextJS app
whenever i subscribe using the paypal buttons, it works just fine, but the only event that's fired is
BILLING.SUBSCRIPTION.ACTIVATED
if i have my subscriptions be billed monthly, should i wait one month for the PAYMENT.SALE.COMPLETED
event?
i was expecting both BILLING.SUBSCRIPTION.ACTIVATED
and PAYMENT.SALE.COMPLETED
would fire when a user first subscribes, then PAYMENT.SALE.COMPLETED
for the next payments
Is it normal behavior for that only BILLING.SUBSCRIPTION.ACTIVATED
is fired at first, and (maybe) only subsequent payments are PAYMENT.SALE.COMPLETED
?
or have i misconfigured my subscriptions or something? (though afaik, i did just a simple subscription)3 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 7/13/2023 in #questions
Clerk auth 'authMiddleware' vs 'withClerkMiddleware' and setting cookies
(https://clerk.com/docs/nextjs/middleware)
I'm trying to set two cookies inside authMiddleware (afterAuth) and it works for setting the first cookie, but any subsequent cookies aren't set at all.
If i try to do it using the older 'withClerkMiddleware', it works tho.
i'm doing it simply like:
is there a way to set two cookies using authMiddeware?
i'm fine with using
withClerkMiddleware
but there's a deprecation warning
What exactly happens when the method is deprecated? Will it stop working?2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 7/11/2023 in #questions
Google sheets API and restricting scopes
Is it possible to somehow restrict API use to only creation/updating of google sheets?
I'm currently referencing this (https://developers.google.com/identity/protocols/oauth2/scopes#sheets) and it seems like the only options are either readonly, or full control of files, it'd be nice to be able to specify more granular control/limits to the file manipulation.
2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 7/10/2023 in #questions
Clerk and provider access / refresh tokens
I'm currently using supabase for auth. One thing i found lacking though is that it doesn't automatically refresh the provider's (.e.g google) refresh token. I've already tried nextauth and afaik nextauth doesn't have this funcionality as well.
So i googled if this is something clerk can handle, found this (https://clerk.com/docs/authentication/social-connections-oauth#o-auth-access-token-wallet ).
Just wanted to confirm that as the docs say, whatever OAuth provider i use, i use call this endpoint (https://clerk.com/docs/reference/backend-api/tag/Users#operation/GetOAuthAccessToken ) and clerk will give me an access token from the provider, while handling the refreshing of the tokens if necessary?
2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 6/29/2023 in #questions
Paypal webhooks with NEXT's edge runtime
I'm currently testing paypal webhooks locally and it runs just fine on the normal runtime but when i turn on edge, i can't get the body of the request (in particular
webhook_event
which is necessary for this: https://developer.paypal.com/docs/api/webhooks/v1/#verify-webhook-signature_post )
any ideas why i can't get the body at all when using edge?2 replies
TTCTheo's Typesafe Cult
•Created by rubberburger on 6/18/2023 in #questions
Paypal subscriptions: how to verify successful sandbox payment
i'm currently experimenting with paypal + nextjs for subscriptions
i think i got it right so far
code goes something like this:
looks like it worked
how do i check if the payment is successful tho? (can't find any info on the developer sandbox accounts about any ongoing subscriptions) (i can't use stripe where i live btw)
2 replies