ReferenceError: XMLHttpRequest is not defined on api endpoint
I'm deploying a NextJS project using next on pages. I'm getting a
"ReferenceError: XMLHttpRequest is not defined"
when trying to run the code block below. It sounds like the worker behind the pages is trying to use XMLHttpRequest
instead of the normal fetch? How do i fix this?
here's the dev branch of my site: https://dev.cf-next-cpa.pages.dev/
The endpoint in question is this:
https://dev.cf-next-cpa.pages.dev/api/spotify_auth/refresh_tokens
-----
The funny thing about this though is that my code has been running perfectly fine before this (for like 400+ commits/deploys) then all of a sudden it stopped working now.
I'm also using supabase but it doesn't seem to be the issue since the error only occurs exactly at the fetch
call.
Did something change with CF workers? The code works just fine locally btw ,also i use the pages
dir instead of the app
dir.11 Replies
this is my prod url btw: https://jamstart.app/
been doing some debugging and it seems like the issue really is with the endpoint, but I'm not sure exactly why, I've also checked that I don't use XMLHttpRequest anywhere in my code
I've checked that supabase does return valid tokens and tried those tokens manually with the spotify API
----
update/edit:
I just checked again, and apparently the problem happens for all of the endpoints, not just the endpoint i mentioned above. It's a bit weird though that sometimes the endpoints would work, sometimes they won't (for example, try searching for songs using the search bar).
When doing this (calling the api endpoints), sometimes the endpoint/worker would throw an error (the
XMLHttpError
thing), sometimes it won't. See images below.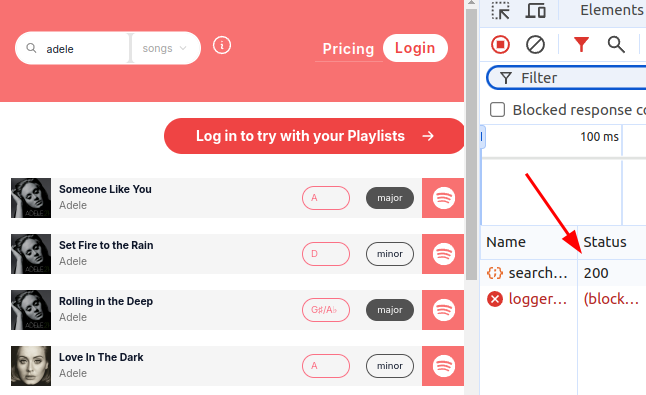
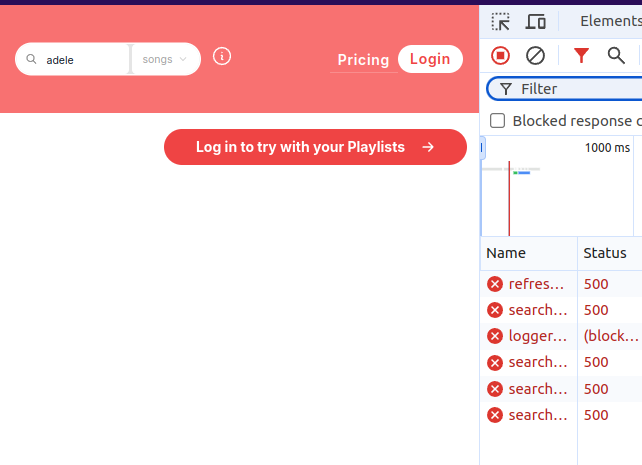
anyone else experience anything similar to this?
I tried deploying it to vercel just to check, and the same code worked
I'd still like to use CF Pages though if possible
the weird thing is that it seems like the problem only happens when the requests are coming from the browser. If it's through curl/postman, it works just fine, and i'm not sure why
@j3llyb3ans hi, I'm facing a similar issue in my preview environment. Have your problem been solved?
My project do not use XMLHttpRequest of course, and code is not changed. I'm contacting the support.
Error:
Nope, spent about a week trying to solve it unfortunately but I moved to vercel for now
Seeing your error however, I'm now wondering if it might be an error related to
gSSP
, maybe we would try removing gssp and see how it goes
thanks for pinging me btw, I hope this works
Oh also, I wonder if moving to the app dir would solve this issue, it would take a while for me to try this out since my app is pretty big
lmk how it goesThank you for your response, I'll think about it a bit.
Got the same issue after upgrade to next-on-pages 1.13.2 which introduce a global scope change on globalThis, revert to 1.12.1 solve the issue
also had same issue on 1.13.2, and the downgrade fixed it for me
@yuripave @borsss
any chance you guys could share your build command and workers compat date? (and maybe other relevant build settings)
holy s**t guys I can't thank you enough for this, it works!
Downgrading to 1.12.1 fixed the issue.
What was your compatibility_date set to? I tried downgrading but it doesn't seem to work. Im currently using 2024-11-11 as my compatibility date.