artus
KPCKevin Powell - Community
•Created by artus on 12/5/2023 in #front-end
Javascript Intervals
i have used
clearInterval()
but placed it in wrong line
tysm ❤️7 replies
KPCKevin Powell - Community
•Created by artus on 12/5/2023 in #front-end
Javascript Intervals
7 replies
KPCKevin Powell - Community
•Created by artus on 6/14/2023 in #front-end
Problem at replacing Node elements (input to text)
HTML:
Javascript:
2 replies
KPCKevin Powell - Community
•Created by artus on 6/14/2023 in #front-end
Auto disabled button when added by js
i found the prob, its in my other function to rename task:
3 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #ui-ux
Make page looks clean and more "intuitive"
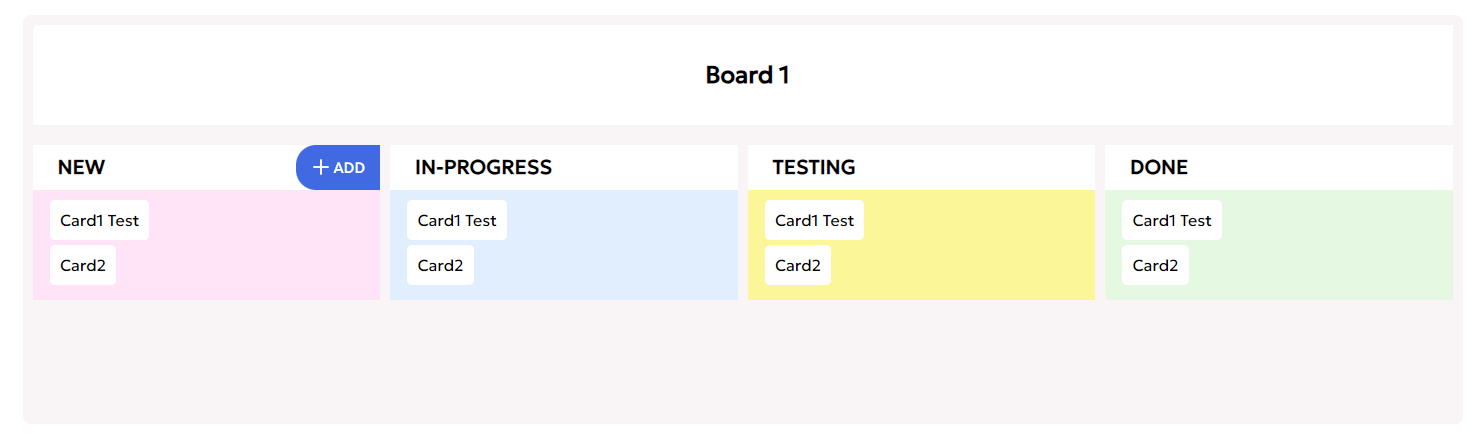
10 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #ui-ux
Make page looks clean and more "intuitive"
done
10 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #ui-ux
Make page looks clean and more "intuitive"
any suggestions about button coulor?
10 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
I'll start using it someday 😆
74 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #ui-ux
Make page looks clean and more "intuitive"
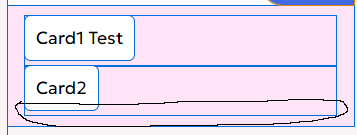
10 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #ui-ux
Make page looks clean and more "intuitive"
its on middle but under "Card2" is another div with
disaply: none
and it make it looks like its not centered
ill add margin-left to cards so they will be equally with title10 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
ik i have to much divs but everytime im making new project i forgot about that and they just stay forever
74 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Adding items to div with title that user can change anytime by clicking on it
sorry that i didnt post solution before
20 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
should i change the post title, cause we did it 100% not as long as text
74 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
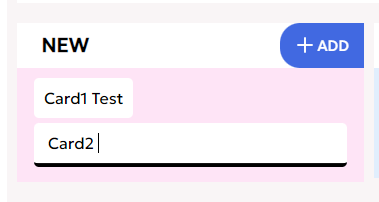
74 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
i just lagged for a moment XD but thanks for that too
74 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
yee i figured it, thanks so much it works ❤️
74 replies
KPCKevin Powell - Community
•Created by artus on 6/11/2023 in #front-end
Edit input field on :focus
what is "children elements"
74 replies