Alex Mason
KPCKevin Powell - Community
•Created by Alex Mason on 2/23/2024 in #front-end
Mantaining field alignment across rows
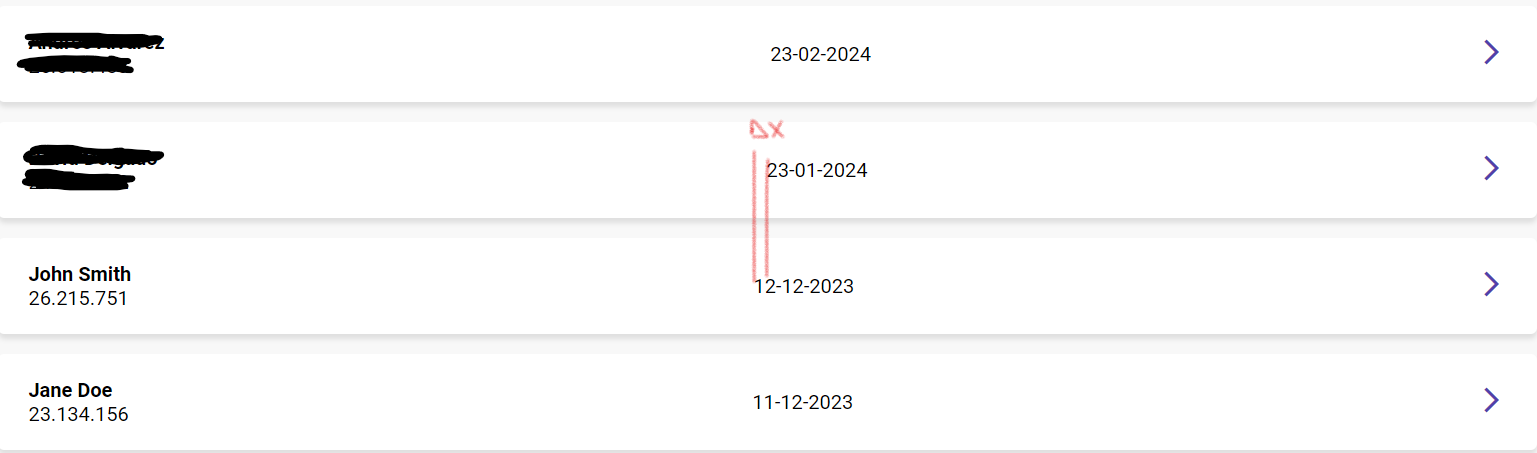
9 replies
KPCKevin Powell - Community
•Created by Alex Mason on 2/9/2024 in #back-end
Trying to update a single movie property with PATCH
Hello, I'm building a REST API and I'm implementing a patchOneMovieById method, that takes an id and a an object of properties to update, I've split my code in the following files: movie.router.ts, movie.controller.ts, movie.service.ts and movie.repository.ts
Here's the code for the service and repository:
The issue is, when I update a single property on a movie, the other properties are deleted and I get an output such as:
1 replies
KPCKevin Powell - Community
•Created by Alex Mason on 1/20/2023 in #front-end
Working with text width
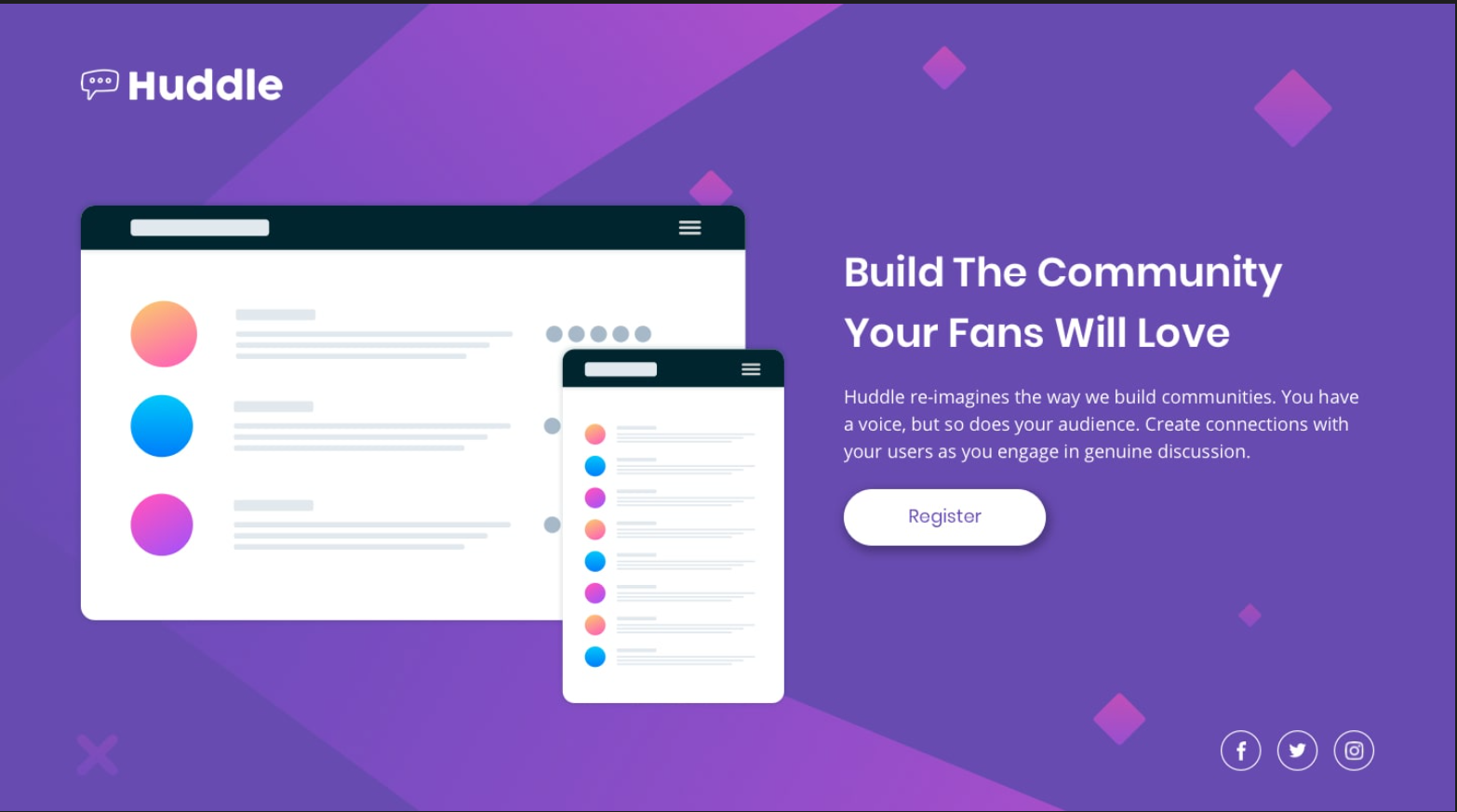
7 replies
KPCKevin Powell - Community
•Created by Alex Mason on 1/11/2023 in #front-end
Error concatenating strings in a for loop
Hello, I'm trying to generate a random string of length 12
What I'm trying to do is, first, generate a random character, using the ASCII table starting at 21 till 127. Then with the String.fromCharCode, I get the character equivalent of the number generated.
The idea is that, I have an empty string and I use the += operator to concatenate whatever char I get from the loop, easy peasy lemon squeezy, nothing out of the ordinary, BUT, and this may be a conceptual mistake, when I run this code I get a reference error saying that
id has already BEEN DECLARED
but I don't see myself redeclaring it so I don't understand.
Also, I've tried with the .concat method and it still gives the same result. Using const doesn't work either.7 replies
KPCKevin Powell - Community
•Created by Alex Mason on 12/17/2022 in #front-end
JS Ignoring my if condition
I'm trying to add the value of a selected radio button to a span after clicking a submit button.
Here's what my HTML looks like
And here's the JS for this:
I'm getting the following error: Cannot set properties of null (setting 'innerHTML')
So I don't understand why I'm getting that error if the .innerHTML part should only be applied if the selectedRating is not null
27 replies