ideceddy
TTCTheo's Typesafe Cult
•Created by ideceddy on 5/4/2024 in #questions
Code seems to stop execution
Im trying to send an email using resend. Locally, the email sends just fine, but when I host it on Vercel, it looks like the function stops execution. One moment the code is running spitting out logs and then it goes silent. It stops right when i get to the call to send the email.
I don't see any timeouts so im confused as to why it fails. It is kind of strange the way I call the function and that is the only thing i can think.
Its a server action that calls a server side trpc query. That query fires off a chain of functions that should result in an email.
In trpc land
And i start the chain with
2 replies
TTCTheo's Typesafe Cult
•Created by ideceddy on 2/23/2024 in #questions
Sharing data across client & server components?
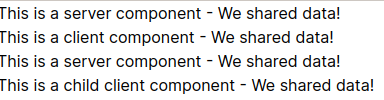
4 replies
TTCTheo's Typesafe Cult
•Created by ideceddy on 6/12/2023 in #questions
How can I run a query only one time when the page loads?
I am trying to create a record of a session when the page loads so I can gather user metrics. Initially I thought that I could create a state variable
(true | false)
Then in a if statement I check if the state is false run my query then set the state to ture.
When I try this I get an error:
Error: Rendered fewer hooks than expected. This may be caused by an accidental early return statement.
When I run the query outside of the if block anytime the component remounts a new session record is created in my database. My goal is to create a session when the page loads, get the id of the new session added to my database and use that ID to update the session periodically as the user interacts with my application.
Can I please get some guidance on how to do this?
As some extra context when I try to use my query in a useEffect block It errors out saying that I cant run a hook inside a hook.53 replies
TTCTheo's Typesafe Cult
•Created by ideceddy on 6/12/2023 in #questions
no-floating-promises error when using TRPC query.
I have this query using TRPC but am getting the following error. I feel like I should be resolving the prommis and don't get why this is happening. At a quick glance can anyone give some guidance?
2 replies