How can I run a query only one time when the page loads?
I am trying to create a record of a session when the page loads so I can gather user metrics. Initially I thought that I could create a state variable
(true | false)
Then in a if statement I check if the state is false run my query then set the state to ture.
When I try this I get an error:
Error: Rendered fewer hooks than expected. This may be caused by an accidental early return statement.
When I run the query outside of the if block anytime the component remounts a new session record is created in my database. My goal is to create a session when the page loads, get the id of the new session added to my database and use that ID to update the session periodically as the user interacts with my application.
Can I please get some guidance on how to do this?
As some extra context when I try to use my query in a useEffect block It errors out saying that I cant run a hook inside a hook.Solution:Jump to solution
I am trying to create a record of a session when the page loads so I can gather user metrics. Initially I thought that I could create a state variable
(true | false)
Then in a if statement I check if the state is false run my query then set the state to ture. ...37 Replies
You're adding data to your DB, so you should be using a mutation.
If a procedure isn't designed to be re-ran at any arbitrary time, it most likely shouldn't be a query
maybe
refetchOnWindowFocus: false
on useQuery can helpAlso you could run the query in
useEffect
, you just can't use the hook, you need to use the client directly via api.useContext()
refetchOnWindowFocus
might do the job but IMO it's a bandaid fixThanks Moving over to a mutation is what I needed to get this to work.
But I don't see a way to get the data returned from the mutation.
I even tired to create a async function to wait for the promise returned. All I ever see is undefined.
When I look at the logging that trpc ( I think ) returns I can see its returning the data at the end of my session router.
return {id: create_session.id }
But I get nothing back from the mutation.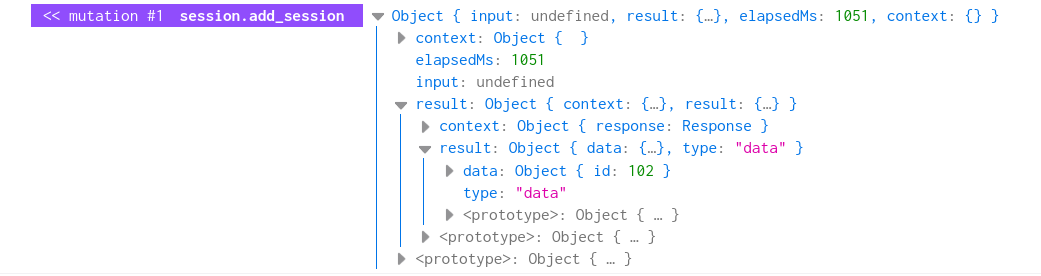
Oh,
mutate
doesn't use async
. You can either provide it with onSuccess
callbacks etc, or use mutateAsync
which will work fine in your async function
useMutation
does return a data
field though, you may as well use that rather than a separate stateI tired to get the return object data from the useMutation but I must have some gaps in my knowledge.
What I ended up with was the onSuccess method and that did the trick.
I'll mark this one as solved 😄
Thanks for all the help ❤️
In the first example, why do you still call
set_session
? data
has everything you need already inside itOh right that was the issue 😄
ayyy nice
I guess the state was not updated yet. its 3AM here :p
also do future you a favour and don't destructure the
useMutation
Like this? I see that destructing in the chirp app but it makes sense if I am going to do more mutations.
oh no does theo destructure
nooooooo theo is cringe
all my homies hate destructuring w/ tanstack
sadge
TBF it looks cool
and the
.
is so far on the keyboard.i hate
isLoading: isPosting
what keyboard layout are u using haha
.
for me is one down from my ring fingerI am a goblen and type with one hand at 3AM
lmaooooo fair
Thanks Again you are the best 😄
happy to help!
I don't think this will run
You can't mutate or query in a useEffect
?? sure u can
we use useEffect for firing our analytics mutations
Trpc procedures?
you can't
useQuery
or useMutation
inside a useEffect
, but you can mutate
and refetch
rspc, basically rust trpc
it's all tanstackBut useMutation is for the backend and useEffect is client based
i don't get your point
Works on local and the edge 🙂

useEffect
is just a mechanism for running code in response to a state change
triggering a mutation in there is fineit's even in the react docs https://react.dev/learn/you-might-not-need-an-effect#sending-a-post-request
You Might Not Need an Effect – React
The library for web and native user interfaces
Maybe things has changed though
it's not
useMutation
exactly but it's the same ideaI remember trying this a while ago and TypeScript was yelling at me
It was yelling at me for useMutation but not mutate.
yea i have a feeling you may have been using
useMutation
in the useEffect
in which case you can't bc it breaks the rules of hooksOh, i see your point now
Your talking of the mutate from the destructed response of useMutation, not .mutation on the backend
ye ye
destructed responsebetter not be destructured hehe