Godspeed
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Godspeed on 5/18/2023 in #questions
Stripe Webhooks
Hi, I've been struggling with the Stripe Webhooks for a while.
Here is my webhook route (src/pages!/api/webhooks/stripe.ts):
No matter how I change this, I get a 500 response without a body in Stripe.
This has become a bit messy from what I had at first.
Is there some config in T3 in other places that I need to modify to allow for webhooks from stripe? Or am I missing something?
I have the correct webhook secret, the correct stripe api key, and it sends webhooks to my vercel url.
6 replies
TTCTheo's Typesafe Cult
•Created by Godspeed on 5/15/2023 in #questions
Extend or duplicate generate SSG helper functions?
Hello! In the T3 0 to prod video, Theo creates a function to generate an SSG helper.
If you want to use a similar concept for other types of data as well (not just userId), is it best to add more fields to
ctx
or to create brand new functions?
Are there any specific best practices for SSG helpers in general?2 replies
TTCTheo's Typesafe Cult
•Created by Godspeed on 5/9/2023 in #questions
T3 hydration mismatch - div in div
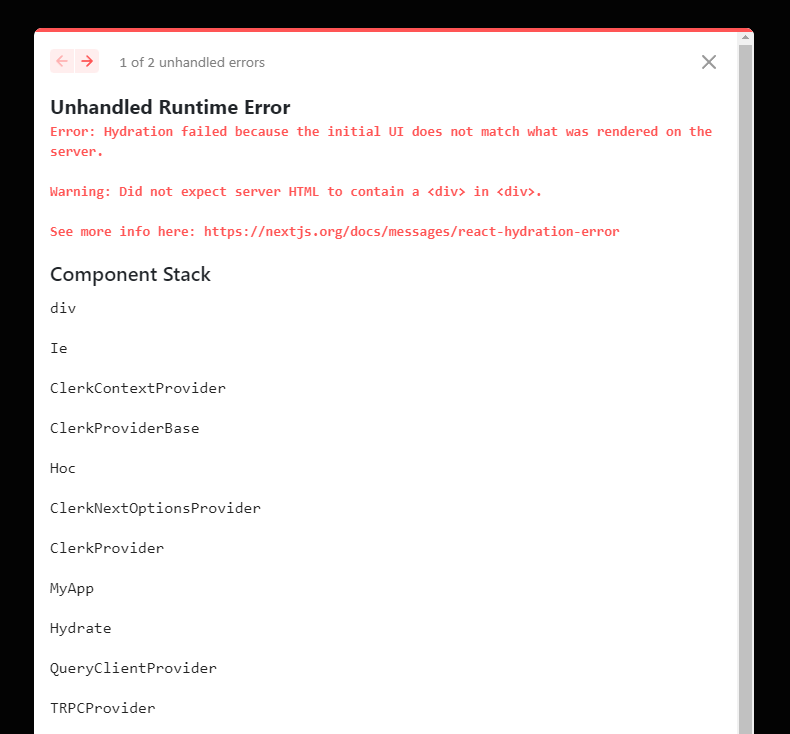
8 replies
TTCTheo's Typesafe Cult
•Created by Godspeed on 5/5/2023 in #questions
Using T3 for AI agents and similar products
Hello - I'm new to T3 and JS/TS, but I'm a .NET dev working with MAUI and Blazor.
Really want to explore T3, but I have some (maybe stupid?) questions.
I find it unnecessarily hard/verbose to use AI tools like LangChain and OpenAI APIs in .NET - especially when I can do pretty much anything from chatbots to agents in Python with 10-300 lines max.
I love Blazor, but frameworks like Next or Svelte are just so much more performant, better documented, plus Github Copilot is way better here than in .NET/Blazor.
I want something that performs better and is easier to manage.
Other than dabbling a bit by making some projects in SvelteKit, I dont really know much about JS/TS web development, but have followed Theo a long while and finally interested in trying the T3 stack.
I read that T3 isnt necessarily meant for microservice setups etc, but I assume it is still possible to let it use APIs/microservice endpoints.
I dont really know how good the dev experience is in TS for AI stuff, but there are tons of JS libs so I also assume that it is pretty straightforward.
I want to combine learning better, more performant web frameworks and also trying out building some new AI agents or chatbots with OpenAI and LangChain etc.
One thing I really want to try is to let users spin up multiple agents at once, maybe as processes running in Docker images.
Kinda clueless on how to set this up still, but I'm hoping T3 and TS can do the job.
Is the T3 stack a good fit to try these sort of projects?
Maybe it's nice to integrate it with some Python backends/APIs as well?
Are there some things one should be aware of with T3 when it comes to scaling web apps?
3 replies
Running PowerShell to install MSIX from .NET MAUI
I am trying to implement a custom auto-updating solution, as the Appinstaller program has issues with the latest Windows Security Update.
I am running a process that checks for new update, and if there is one, it downloads the new MSIX.
I am able to download the MSIX file, and I am trying to install it using the PowerShell.Core.SDK, with the following code:
I am having issues getting the
Add-AppxPackage
command to work from the instantiated PowerShell. I retrieve errors from ps.Streams.Errors.
The main error output I get is {The 'Add-AppxPackage' command was found in the module 'Appx', but the module could not be loaded. For more information, run 'Import-Module Appx'.}
.
Thus, I tried adding scriptContents.AppendLine("Import-Module Appx");
.
This only resulted in the error {Operation is not supported on this platform. (0x80131539)}
.
Googling a bit, I found a GitHub issue referencing this error, https://github.com/PowerShell/PowerShell/issues/13138.
Thus, I added the flag to it as such: scriptContents.AppendLine("Import-Module Appx -UseWindowsPowerShell");
.
I am not sure if this is a good solution on its own, as some comments mentioned that this flag is not supported on Windows 11.
Anyway, adding this flag avoid the last error, but returns us to the first error {The 'Add-AppxPackage' command was found in the module 'Appx', but the module could not be loaded. For more information, run 'Import-Module Appx'.}
.
Q: How can I avoid this error? Am I missing something? Is it not possible?
I need this to work on a variety of Windows machines (personal, consumer units). Is there a better approach than the PowerShell.Core.SDK?13 replies
MSIX URI is inaccessible in Appinstaller
Hello,
I have an Appinstaller and MSIX hosted in a public Google Cloud Storage bucket, meaning they have directly accessible URIs on the form "https://storage.googleapis.com/{bucket-name}/{file-name}".
However, the Appinstaller gives the (not very detailed) message "Error in parsing the app package" when I attempt to run it.
The Windows Logs for AppXDeployment give no useful insight.
I have tested this with MSIX Troubleshooter (https://www.advancedinstaller.com/msix-troubleshooter.html), and it tells me that the Appinstaller URI is "accessible" and the MSIX URI is "not accessible".
If both are hosted in the same public bucket, how come the appinstaller sees MSIX as inaccessible and the other one not?
The Appinstaller looks like this ish:
11 replies
Disable prerendering on a single component in Blazor WASM
I currently have prerendering enabled in my Blazor WASM hosted project (in
_Host.cshtml
through <component type="typeof(App)" render-mode="WebAssemblyPrerendered" />
), and prerendering works.
However, I have one component in my app that should not be prerendered, and it's prerendered state would only cause confusion by my users. Is there a good way to exclude a single component from prerendering?31 replies