steven preadly
Explore posts from serversKPCKevin Powell - Community
•Created by steven preadly on 11/3/2024 in #front-end
How can I solve this swiper styling issue in the video ?
hello i am using Laravel to create a books store there are a section for highly ranked books i am using swiper Api in this swiper and below is the code the swiper in js and a video of that happiens whe relad the page
15 replies
✅ Use() Extension Overload
I'm working with the Use method in ASP.NET Core and have encountered two overloads. The first, taking HttpContext and RequestDelegate, is clear to me. However, I'm struggling to understand the second overload where the use lambda accepts HttpContext and Func<Task>. Some articles mention that this approach leads to additional allocations per request. Could someone elaborate on what these extra allocations are and how they impact performance?
7 replies
✅ Factory-based middleware activation in asp.net core
this part of the docs says that Factory-based middleware is activated per request while when i register this middleware in app service container it is registered as a transient or scoped , this part confuse me is it registered twice
https://learn.microsoft.com/en-us/aspnet/core/fundamentals/middleware/extensibility?view=aspnetcore-8.0
25 replies
✅ ASP.NET Core: Confusion with builder.Services.AddTransient() and App Lifetime Management
I'm new to ASP.NET Core, and I'm encountering some confusion regarding service registration. In the code snippet below:
We see that builder.Services.AddTransient<RequestCultureMiddleware>() is used to register the RequestCultureMiddleware service with a transient lifetime. However, I understand that the WebApplication.CreateBuilder(args) method builds the application configuration, while service registration is performed at the application level using app.
My question is: Why can't we register services like RequestCultureMiddleware directly with app.Services.AddTransient<RequestCultureMiddleware>() instead of using builder.Services? What's the underlying principle behind this separation?
13 replies
✅ Extension Methods
this is an extension method that i have read in the docs , my question is when i use this extension method as below code in that case the
this
keyword bind the sentence string inside method as i did not pass any parameter correct?
8 replies
✅ Thread and Threading
i have asked this question on the #help-0 an here is the link https://discord.com/channels/143867839282020352/169726586931773440/1269020486952816671 according to conversation with @TeBeCo i
have changed the code to be like below by question is now
T1.join() will block the main thread till it resolves , and then the T2 will block the main thread and the T1 Thread till it resolves is that correct
24 replies
✅ positional parameters
dose this sentence
You can use positional parameters in a primary constructor to create and instantiate a type with immutable properties.
form docs means that the record create a primary constractor that contains the parameter , or it means that i can create a primary constractor inside the record type with the parameter , as i read the record creates by default a primary constructor and a proparites that is inilized using this constractor31 replies
why the Clist.Contains(customer) is not getting true in that case ?
why the Clist.Contains(customer) is not getting true while the new object that i created is already on the list dose that means that i have to override the Equals method in the customer class
i have tried to override the Equals() method in the customer class but, what was intersting is that it works
32 replies
✅ I have been hired in a company that is still using c# with webforms asp.net
I have been hired in a company that is still using c# with webforms asp.net , they dont have the intension to migrate to aspCore i am one of the juniors in my team what should i do can any one give me opinion? do i have to study asp.net webforms fully because i have only the basics of it and them as a update for my self i study asp.net core ? or what should i do
21 replies
✅ what will be the default value of the `params int[] value` if i did not pass it while calling M
what will be the default value of the
params int[] value
if i did not pass it while calling method , do this will be trated as an empty array of ints
22 replies
KPCKevin Powell - Community
•Created by steven preadly on 6/29/2024 in #front-end
what are the ways that i can follow to mange the height in card created using bootstrap
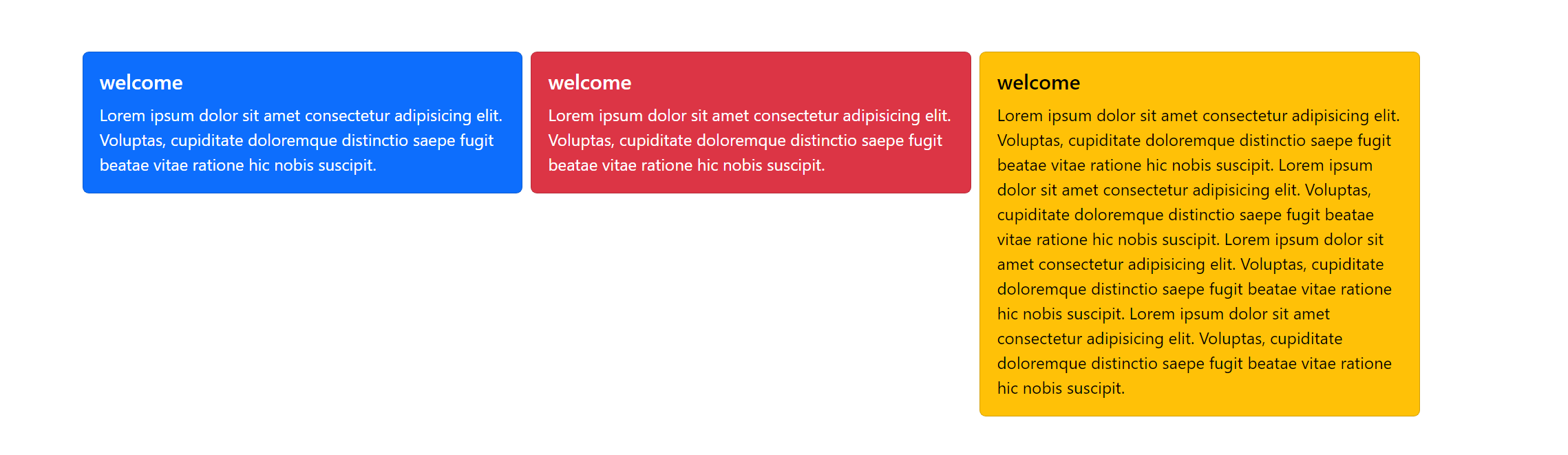
18 replies
KPCKevin Powell - Community
•Created by steven preadly on 6/20/2024 in #front-end
Why is the <div> with position-absolute and start-100 positioned outside the container?
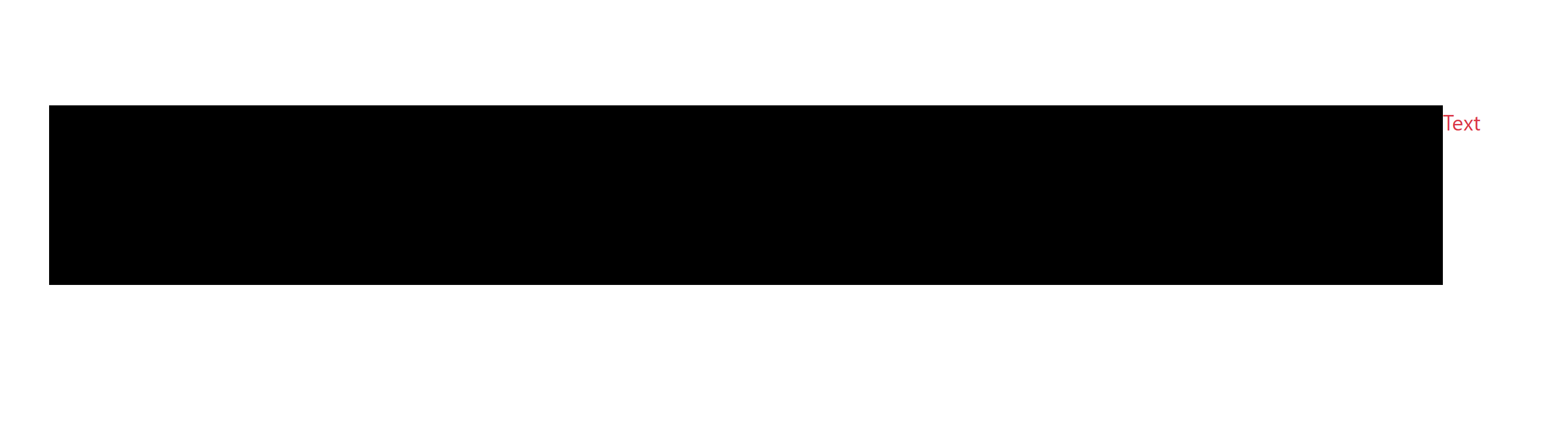
17 replies
Calculating Student Grade Book Average (GBA) in Percent - Encountering Zero Value Issue
I'm working on a student class with properties mark and totalMark. My goal is to define the totalMark property to compute the GBA as a percentage. However, every time I implement the calculation, the totalMark value consistently returns 0.
63 replies