Revaycolizer
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Revaycolizer on 7/6/2024 in #questions
Unable to upload files after deploying on vercel
This is how I implemented it but, does not seem to work as I get an error stating that no such file or directory '/vercel'
3 replies
CCConvex Community
•Created by Revaycolizer on 3/26/2024 in #show-and-tell
Messaging web app
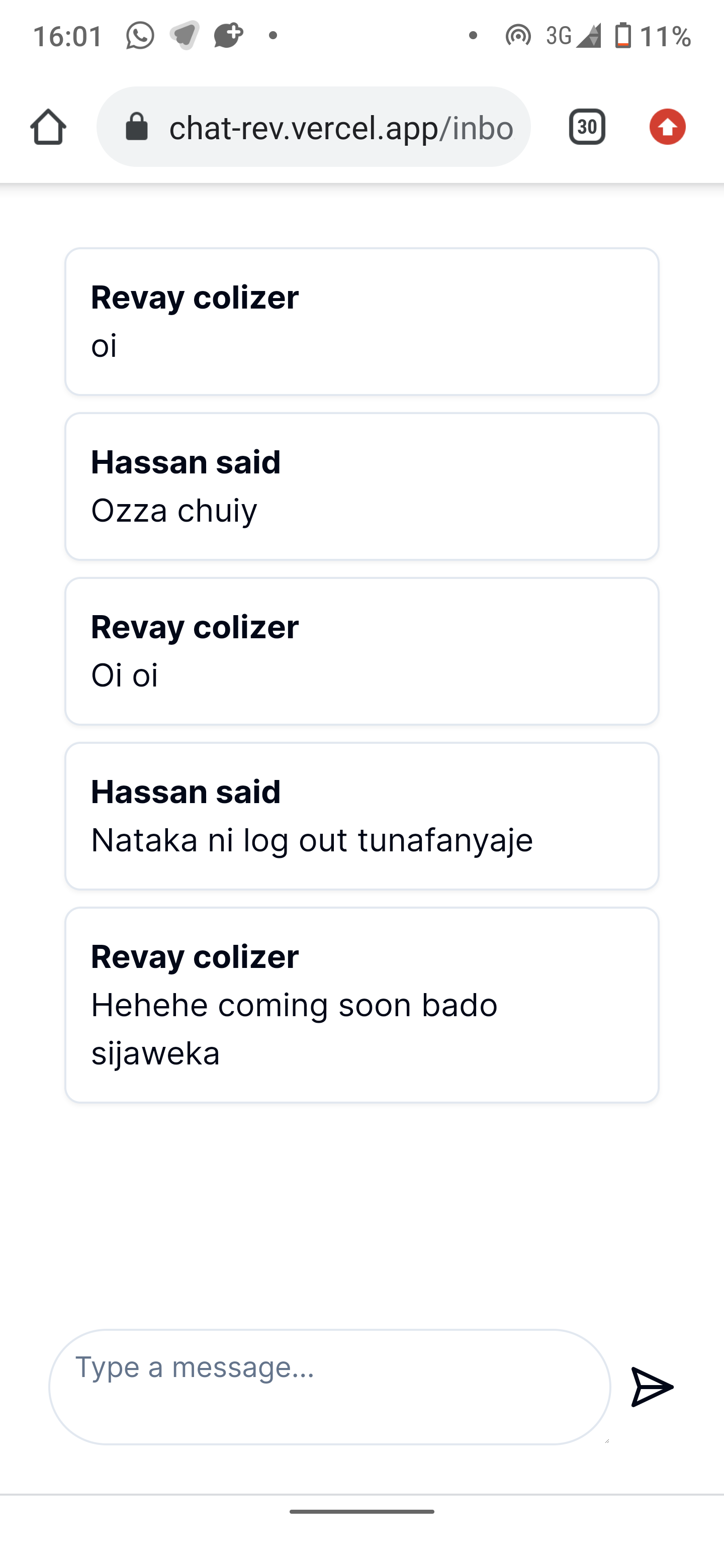
63 replies
CCConvex Community
•Created by Revaycolizer on 3/24/2024 in #support-community
Realtime data not working anymore
My project was successfully returning realtime data, but this time until I refresh my page, what may be the cause?
2 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 10/17/2023 in #questions
Conditional Rendering problem
I am having trouble when a user likes he/she cannot unlike the post thus causing undefined value to be sent to the API endpoint using source code below
2 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 10/5/2023 in #questions
Consuming API's created by nextjs
Consuming API's create by nextjs in flutter but getting this error
Check no 'Access-Control-Allow-Origin' header is present on the requested resource. Below is the source code of my next.config.js
Also my route handler I'm consuming
2 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/20/2023 in #questions
Route handlers errors after updating
Guys anyone updated to version 13.5.2 and getting this error
Expected "Response | Promise<Response>", got "Promise<NextResponse <any> | undefined>"
2 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/8/2023 in #questions
Football live Stream Package
Which football live stream package should I use for nodejs and is it safe to use?
8 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/5/2023 in #questions
Implementing Middleware
Hi guys been implementing Middleware to protect admin and user page but to some points I failed to protect two pages /adupload for admin to access and /home for only user to access. Below is the link to how I implemented it
https://github.com/Revaycolizer/next-learn14/blob/main/middleware.ts
3 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/5/2023 in #questions
Some of the conditions are omitted in middleware
some of conditions are not checked by the middleware as when a user is authenticated and navigates to /register he should be redirected back to /home but it does not do that only /login condition works but others do not work using codes below
6 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/5/2023 in #questions
Fetching data in server Component
Is there a way I can fetch data from my api generated in my api routes in server Component rather than writing the route handling logic in my server Component
3 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/4/2023 in #questions
Error:localhost redirected too many times
Whenever the user is authenticated and a web page is refreshed I keep getting this error "error:localhost redirected too many times try to clear cookies". Using codes below b
4 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 9/2/2023 in #questions
Implementing get Request with Prisma
I impleented login route using prisma and also Redis is being used for session management but when a user is logged in another device and the other user opens the website in another device without logging in the returned user is the one who was authenticated from the other device, How can i fix this below is the source codes
3 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 8/31/2023 in #questions
Implementing login with Prisma
Below is the source code of my api but I figure out that any one entering credentials when an entered email resemble that of the one in the database, the user is authenticated without checking the corresponding password, how can I fix this, as the stored password is hashed?
20 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 8/30/2023 in #questions
Vercel Caching Data during Deployment
Anybody hosted a website in Vercel recently, as when a data is fetched during deployment, it caches it even if the data is changed, so everytime a data is changed I need to click redeploy again, how can I fix this?
Tried
also tried
But nothing works
9 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 8/23/2023 in #questions
Storing and retrieving cookie
Anyone successfully stored cookie in one route handler and retrieving it in another route handler?
23 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 8/21/2023 in #questions
Storing and getting cookie in api
Anyone successfully created a login api and stored cookies using prisma and then created an API to fetch the cookie?
2 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 8/19/2023 in #questions
Fetching data from API endpoints
Is there a way to fetch data from client Component without exposing API endpoints on frontend?
9 replies
RRefine
•Created by absent-sapphire on 7/22/2023 in #ask-any-question
Route protection
Anyone ever used route protection using refine and Zustand for state management
4 replies
TTCTheo's Typesafe Cult
•Created by Revaycolizer on 7/12/2023 in #questions
Handling cors using axios
How can I perform XMLHttpRequest using axios
4 replies