Martnart
Explore posts from serversDTDrizzle Team
•Created by Martnart on 8/30/2023 in #help
where(gt) with timestamp returns wrong result
Might be a SQL inherent thing, but I'm a bit puzzled as to why this happens:
Edit: for terseness I left out some code. I think the issue is clear
27 replies
Building with SSR false starts (and doesn't close) listeners
In my app I create a WebsocketServer on Port 3001.
If I run
npm run build
with default SSR setting (ssr: true
) everything works like a charm.
However, turning off ssr
, the WebsocketServer gets started and not closed => EADDRINUSE error. Cannot run dev
or start
afterwards.
After forcefully terminating the process, I can run start
with the built files and it works.
Any suggestions on how to avoid this?18 replies
DTDrizzle Team
•Created by Martnart on 4/28/2023 in #help
TS Errors in Custom Type citext example
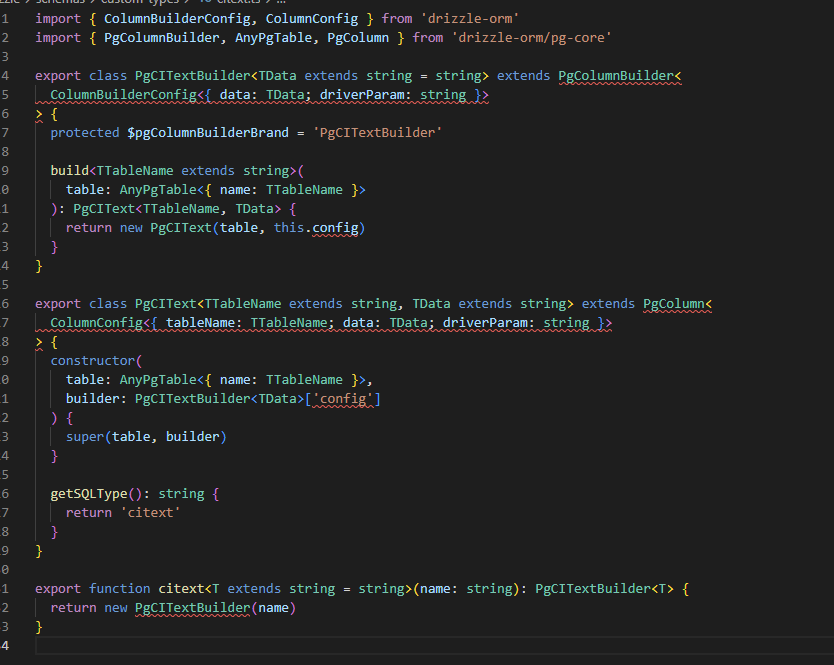
4 replies
DTDrizzle Team
•Created by Martnart on 4/27/2023 in #help
Check for empty string in postgres
Hi. First post. 🙂 Started looking into migrating our project to drizzle today and am loving it so far.
I want to add a not-empty check and from what I gathered from the docs and this example https://github.com/drizzle-team/drizzle-orm/blob/b003e523c637c81e2c522003db03d3d9bd98b723/drizzle-orm/type-tests/pg/tables.ts#L76 (which is the only one I could find) this code should work:
But it doesn't :/ the index works, but the check does not. There is no SQL generated for the migration but to be sure I also tested it in runtime after migrating but no luck. Any advice?
7 replies
Hydration issues on navigation while hydration is ongoing
With a structure like this, navigation is enabled before hydration is complete.
While hydrating, users can already use the sidebar to navigate to different routes. However, this leads to hydration errors and this also leads to an issue where two A components are "active" at the same time, i.e. "activeClass" is applied to two link components simultaneously.
Is there anything I can do to prevent this?
Edit: Might well be that I mis-analyzed the circumstances of this. Please excuse me if that's the case. The problem is very real, though 😮
1 replies
RouteDataArgs Type Error for nested RouteData
Wha'ts happening here? I tried to reproduce this in Stackblitz and couldn't. I'm guessing some TS config option maybe as type inference seems a bit messed up.
https://stackblitz.com/edit/solid-ssr-vite-pcbdkr?file=src%2Froutes%2Findex.tsx
However, locally, routeData return types are accurate and I get above error for this kind of structure.
3 replies
Make routeData blocking
Following scenario:
(app).tsx
route.tsx
Everything underneath (app) layout should be protected by auth. However, if I have it like this, the fetch of my route starts before the user has been verified.
What is the best way to overcome this, without having to go into every single routeData across my app and wrap everything inside some kind of effect that tracks some global user.
Basically, is there a way to make some part of a route blocking further execution until its data has resolved?
4 replies
document is not defined in HMR
I have some logic that is running in onMount and onCleanup that adds/removes a data-attribute from an element. It works. However when I make a change somewhere in my project, I get
document is not defined
error from HMR. It seems to be triggered by cleanNode
so I am pretty sure it's from the onCleanup
.
The code itself looks like this shouldn't happen though because I use optional chaining. Any ideas?
Thank you 🙂4 replies
Await resolution of previous routeData in nested routeData
I have a route that fetches some data.
In a nested route, I want to await the result of parent route data first, before doing another fetch
CSR works fine, because I navigate from the parent route and the data is available. SSR does not work.
routeData
apparently is running multiple times in SSR and even though my log shows that the data is there, the function still executes past the initial guard clause - probably only in the first passthrough (I get no log though :O) when the data is still undefined. How can I tell solid to wait for data()
to resolve before continuing on with the function execution? I'd like to avoid wrapping everything inside memos, effects, etc., which would mess up my returntypes and adds unnecessary complexity to something that should be solvable with a simple await
. I can't find any help in the docs regarding this. Thank you in advance 🙏3 replies
Ref either locally or passed via prop
I have a TextField that requires a ref to an input field.
So far so good.
But in case I have a parent component that also wants to ref the input, I am no longer sure how to handle it.
I have something like this
This works for outer ref, but breaks fallback inner ref. What is the correct way to handle this case? ❤️
5 replies
use:clickOutside not defined
Heyhey. I copy pasted the code from https://www.solidjs.com/tutorial/bindings_directives?solved but I get a runtime error
ReferenceError: clickOutside is not defined
. What am I missing here? I've added the TS declaration as mentioned in the docs here https://www.solidjs.com/docs/latest/api#use___ . My linter is complaining but I don't think it would have any impact on the solid compilation anyway?3 replies
SSR Hydration error in ChildRoute with Context set in ParentRoute and intermediate Context Update
I have a ParentRoute that retrieves RouteData and uses that data to populate a Context
In my child route, I get hydration errors when accessed directly via SSR. With Client-side navigation it works fine.
Context looks like this
Any ideas what went wrong here?
❤️
4 replies
Forward reference a Component's state in Template
I try to wrap a Trigger and a Display component in a state managing component like so
Is this possible? Background is, I want the triggering component to be dynamic. Might be a button, checkbox, input field, etc. And I would like to avoid having to hardcode every event handler
Maybe there's also a more straightforward way to achieve this instead of this approach?
Cheers!
15 replies