Table custom filter validate
I have created a custom table filter to filter the product by price.
How do I validate that a minimum price is not greater than the maximum price or vice versa for maximum price.
php
Filter::make('price_filter')
->form([
TextInput::make('min_price')
->label('Minimum Price')
->numeric()
->minValue(0)
->default($min_magento_calculated_price),
TextInput::make('max_price')
->label('Maximum Price')
->numeric()
->minValue(0)
->default($max_magento_calculated_price)
->maxValue($max_magento_calculated_price),
])
->query(function (Builder $query, array $data): Builder {
return $query
->when(
($data['min_price'] || $data['max_price']) ?? false,
function ($query) use ($data) {
$query->with('supplier', function (Builder $query) {
$query->where('is_sync_on_magento', true);
})->whereBetween('magento_calculated_price', [$data['min_price'], $data['max_price']]);
}
);
}),
php
Filter::make('price_filter')
->form([
TextInput::make('min_price')
->label('Minimum Price')
->numeric()
->minValue(0)
->default($min_magento_calculated_price),
TextInput::make('max_price')
->label('Maximum Price')
->numeric()
->minValue(0)
->default($max_magento_calculated_price)
->maxValue($max_magento_calculated_price),
])
->query(function (Builder $query, array $data): Builder {
return $query
->when(
($data['min_price'] || $data['max_price']) ?? false,
function ($query) use ($data) {
$query->with('supplier', function (Builder $query) {
$query->where('is_sync_on_magento', true);
})->whereBetween('magento_calculated_price', [$data['min_price'], $data['max_price']]);
}
);
}),
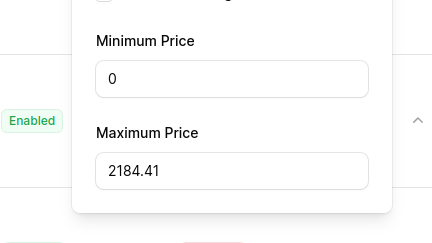
5 Replies
Can you try like this
TextInput::make('min_price')
->maxValue($max_magento_calculated_price),
TextInput::make('max_price')
->minValue($min_magento_calculated_price),
TextInput::make('min_price')
->maxValue($max_magento_calculated_price),
TextInput::make('max_price')
->minValue($min_magento_calculated_price),
Yes, I have tried this, but it does not work.
Hmm, how to validate table filter input? ->rules(... doesn't work..
use for fields
->gt('min_price')
//and
->lt('max_price')
->gt('min_price')
//and
->lt('max_price')
This rules doesn't apply on filter inputs..