taalhaataahir01022001
MModular
•Created by taalhaataahir01022001 on 11/14/2024 in #questions
Parallelize Issue
This is my code:
I get the following output error:
But if I un-comment the last three lines of code, it works fine. Can anyone please help!
4 replies
MModular
•Created by taalhaataahir01022001 on 10/8/2024 in #questions
Reshape MAX API OP giving error
My code:
Error:
candidate not viable: argument #1 cannot be converted from 'List[Dim, 0]' to 'List[Dim, 0]'
4 replies
MModular
•Created by taalhaataahir01022001 on 8/29/2024 in #questions
Dynamic Shapes to MAX GRAPH API
Giving dynamic shape to the graph is giving me error. My code:
Error:
But if I do It works fine. And the issue is only with concat function. I've tried giving dynamic shapes with matmul and it's working fine. Can anyone please guide what I'm doing wrong here?
3 replies
MModular
•Created by taalhaataahir01022001 on 7/26/2024 in #community-showcase
Inference CNN model in mojo (yolo implementation)
I've been working on making a simple inference model in mojo🔥 . A lot of work is still needs to be done and in progress but i made few accomplishments. Wanted to share with the community. I'm new so would love to get feedback on what other things I can try to improve my inference 😄
https://www.linkedin.com/pulse/implementing-yolo-mojo-talha-tahir-an6jf/?trackingId=UDEBRDekTZS%2FQcCEJIBPmg%3D%3D
https://github.com/10x-Engineers/Mojo-Yolo
2 replies
MModular
•Created by taalhaataahir01022001 on 6/4/2024 in #questions
Time taken in Inference session
This is my code:
Output:
Why creating an inference session and loading the graph into the inference session to create model taking a lot of time i.e., around 4 seconds?
Also is there a work around to reduce this time as I have to create different models for my use case.
MAX version: max 24.3.0 (9882e19d)
4 replies
MModular
•Created by taalhaataahir01022001 on 5/27/2024 in #questions
LLVM Intrinsics
I'm trying to use llvm.matrix.transpose intrinsic like following:
But Mojo is crashing:
My input and output types looks OK as I've followed it from here: https://llvm.org/docs/LangRef.html#llvm-matrix-transpose-intrinsic
Also I've tried using other intrinsics and they are working fine. e.g., llvm.vector.reduce.add:
And it outputs 46 correctly.
Can anyone guide me what I'm doing wrong while using the llvm.matrix intrinsic?
7 replies
MModular
•Created by taalhaataahir01022001 on 5/21/2024 in #questions
x86 Intrinsics
How to use x86 intrinsics in Mojo? Also I see in the documentation (https://docs.modular.com/mojo/stdlib/sys/intrinsics/llvm_intrinsic) on using llvm_intrinscs. Can someone please share some example on how they've used them. I'm trying to use it but getting error:
ERROR:
5 replies
MModular
•Created by taalhaataahir01022001 on 5/21/2024 in #questions
Max Engine Understanding
Can anyone please guide me what's the purpose of MAX engine? This is what I understood:
It's some compiler which compiles the model graphs to boost up their performance. But it's not the Mojo compiler.
Assuming I write my whole model in Mojo (I implement all the APIs required for the model in Mojo myself). Then Which compiler will be better? If Mojo is going to be faster (assuming I write the best possible code with Mojo 🔥 ) then what's the purpose of MAX Engine 🏎️ ?
9 replies
MModular
•Created by taalhaataahir01022001 on 5/16/2024 in #questions
C/C++ Interpol
https://docs.modular.com/mojo/roadmap#cc-interop
When will we have this feature gven in the roadmap? Also right now is their a way to use C++ through mojo?
2 replies
MModular
•Created by taalhaataahir01022001 on 3/26/2024 in #questions
String to float
How can i convert string to float32?
e.g.,
atol is not helping as it's used to convert to integers and not float.
4 replies
MModular
•Created by taalhaataahir01022001 on 3/12/2024 in #questions
GRAPH API MOTensor not working
Facing issues with graph API tutorial:
https://docs.modular.com/engine/graph
What is this MOTensor?
It's not available to me :/
2 replies
MModular
•Created by taalhaataahir01022001 on 1/18/2024 in #questions
Access Command Line arguments
How Can I access Command line arguments in mojo like we do in python using sys:
Trying the same in mojo:
Yeilds the following error:
error: 'argv' expects 0 input parameters, but 1 was specified
print(sys.argv[1])
4 replies
MModular
•Created by taalhaataahir01022001 on 1/14/2024 in #questions
Create 1D array of objects
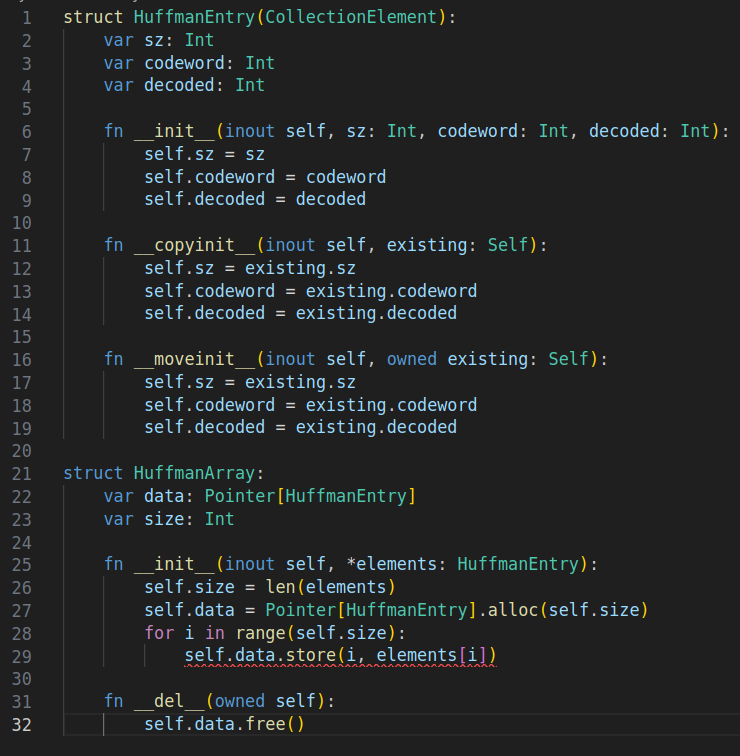
17 replies
MModular
•Created by taalhaataahir01022001 on 1/8/2024 in #questions
How to use f-string in mojo
I want to write a ppm file in mojo using f-string pattern. e.g., thingss like:
out.write(f"P3\n{pic.size_X} {pic.size_Y}\n255\n")
out.write(f"{clamp(round(r))} {clamp(round(g))} {clamp(round(b))} ")
etc. But I can't use f-strings in mojo. Plus if I try to do like this:
out.write("P3\n",picture.size_X, picture.size_Y,"\n255\n")
It gives me the following error:
error: no matching function in call to 'write':
out.write("P3\n",picture.size_X, picture.size_Y,"\n255\n")
~~~^~~~~~~~~~~
/home/talha/Desktop/mojo/jpeg/C-code/Mojo/main.mojo:1:1: note: candidate not viable: callee expects 2 arguments, but 5 were specified
Can someone please help me how to write files?
4 replies
MModular
•Created by taalhaataahir01022001 on 1/5/2024 in #questions
How to convert a byte to integer?
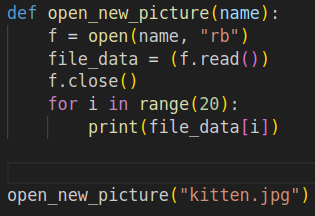
4 replies
MModular
•Created by taalhaataahir01022001 on 1/4/2024 in #questions
Dynamic Vector of objects
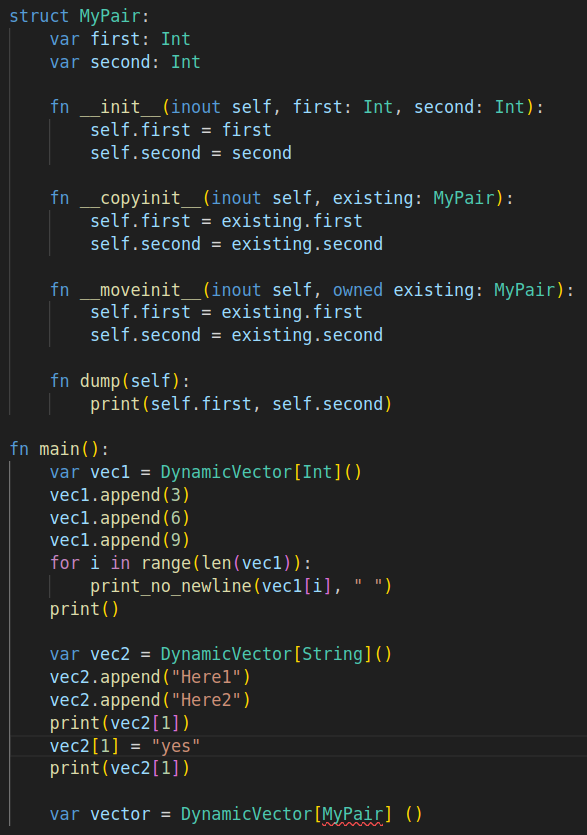
3 replies