glutonium
Explore posts from serversKPCKevin Powell - Community
•Created by glutonium on 11/29/2024 in #front-end
quick question regarding making li focusable using tabindex
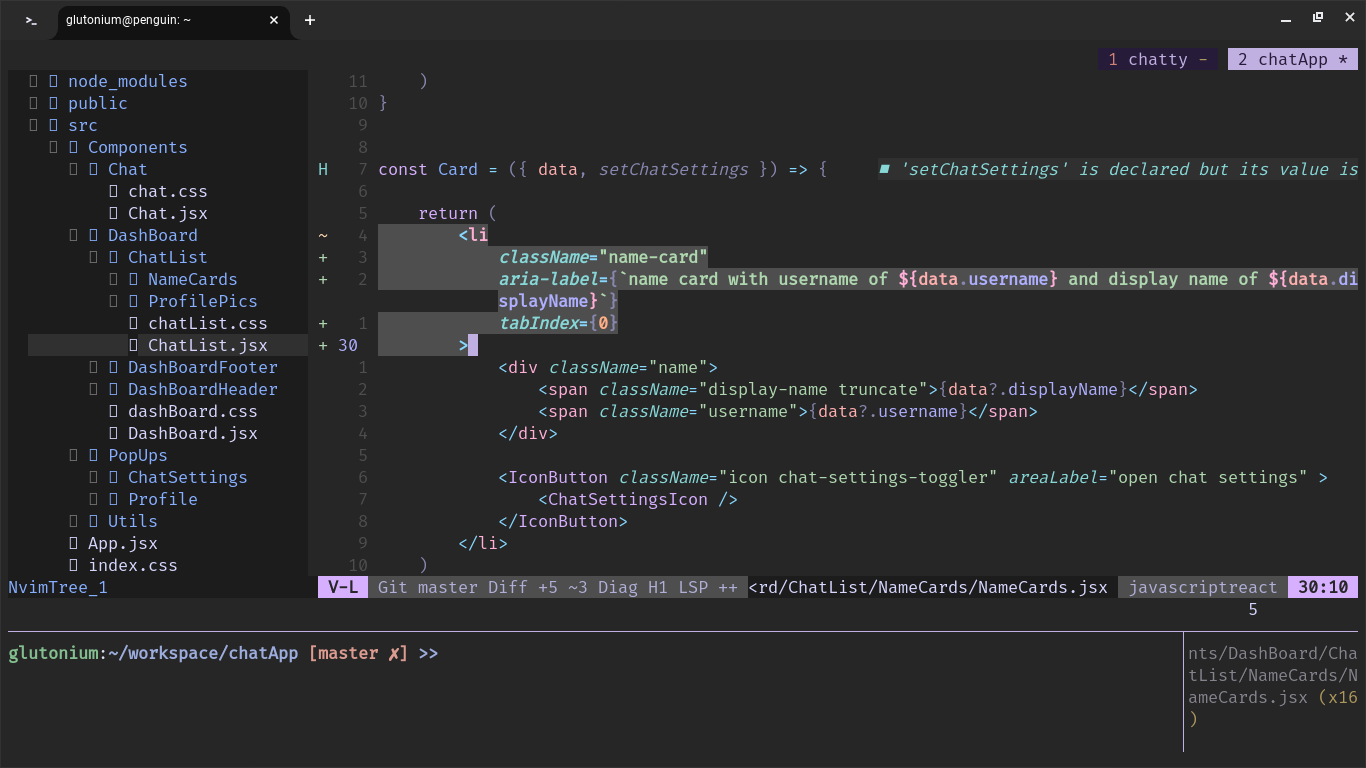
6 replies
KPCKevin Powell - Community
•Created by glutonium on 11/12/2024 in #resources
Create API with fake JSON data
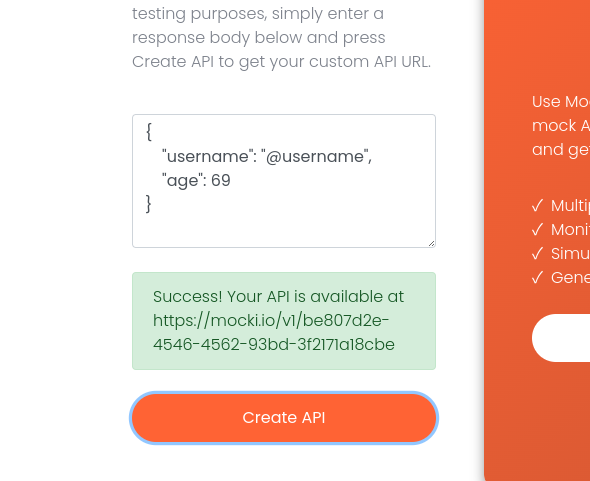
9 replies
KPCKevin Powell - Community
•Created by glutonium on 11/10/2024 in #resources
Understanding git and github
https://youtu.be/9ToMEN_Hys0?si=uJbRVItPv1hwMc3f
this is hands down the best git and gh introduction I have ever seen
would highly suggest anyone who wants to understand git and gh
1 replies
KPCKevin Powell - Community
•Created by glutonium on 5/25/2024 in #back-end
Necessity checking if video exists or not
here I am making a add comment to video controller
i am fetching the video id (mongoDB id) from url param and user id from req.user (a custom middleware is responsible to check if user is logged in or not and if so adds the user to req object)
then if video id is valid mongo id and comment content exists I am creating a new comment doc.
Now my question is i am not checking if a video with that ID already exists or not, so Should I do a query for that or do i not have to since user will have to click a video in order to be able to comment on it
10 replies
KPCKevin Powell - Community
•Created by glutonium on 4/25/2024 in #front-end
Using JS to redirect instead of anchor tag
The
makeCard()
basically returns a div thats wrapped in an anchor tag.
And I am adding click event listener to all the anchor tags cause i have to store the id in local storage of the card that has been clicked .
This works but idk why something feels sketchy about this to me. Idk what. Does this approach have any drawback?49 replies
KPCKevin Powell - Community
•Created by glutonium on 2/6/2024 in #back-end
What does the backend server listens to??
when u make backend, does your server listen to your pages URL?? that is when u say host your webpage, it is hosted on a url. does the the back listen to THAT url? or the localhost of the machine that hosts the site?
The little so knowledge i have about backend thus far, FR and BE communicate with eachother via API. But the question i asked above, this occurred to me when I was thinking about how when u click a link, it redirects user to a certain route.. Now there 2 possible ways that can be done as far as I can think of
We just simply use anchor tags or js with location.href to simply add that route to the Link and redirect. As user lands on that page, static elements r rendered and dynamics infos r fetched using API from the backend and later rendered. In this case i suppose the server can just listen to the machines localhost??
2ndly, server listens to the users location and based on where user is server does it's stuff..Now idk if 2nd one is even a legit way to do it . but these r the 2 possibilities i can think of. Thanks!
9 replies
KPCKevin Powell - Community
•Created by glutonium on 1/29/2024 in #back-end
req.body returns undefined
haya!!. So today i was thinking of trying out backend. and i installed express and nodemon and started working on it. everything seemed to be working fine until I made a form in my html and tried to receive and log data in my server.js. But it seems like req.body always returns undefined . I know the server and client side is working fine cause when I submit, it gives my the error I coded in the
server.post()
. That means i am indeed sending post req to my server . Here is the github code the code: https://github.com/glutonium69/Codespace_1/50 replies
KPCKevin Powell - Community
•Created by glutonium on 12/2/2023 in #front-end
Issue with stylesheet
so i am this issue here as u can see in the video where my stylesheet is for some reason being added multiple times and being removed continuously. And u can see my page is also loosing styles for that when the stylesheet gets completely removed. I am doing a react project here and this is the first time i have encountered this issue. Idk what else info i should add here but pls do ask if theres anything i should let you know
here is my github repo for this project
8 replies
KPCKevin Powell - Community
•Created by glutonium on 11/25/2023 in #front-end
Confusion with SCSS
in the video that i added, u can see i have made a
utilities
folder for styling purpose where i have 2 files reset.scc
which is for css reset and variables.scss
which is fro global variable
now they r imported in my App.scss
i didn't install any scss pkg using npm, only a live scss compiler, beforehand when i made App.scss
and started the live compiler it made 2 files App.css
and App.map.css
and my compiled code gets imported in App.css
now after making the utility files when i started the compiler, it made both .css
and .map.css
for each of my scss file and compiled the code in their respective .css
file
but since i had them @imported './path'
in my App.scss
as well, the compiled code of my utility files are also in my App.css
so now i am confused about what to do. I thought it was supposed to only make a css file for my App.scss
and as other scss files will be imported here it'll compile all of them into one App.css
file
So my question is, am i getting it wrong or is it supposed to not work like it is rn?
tnx ❤️1 replies
KPCKevin Powell - Community
•Created by glutonium on 11/18/2023 in #front-end
run function once but globally in react
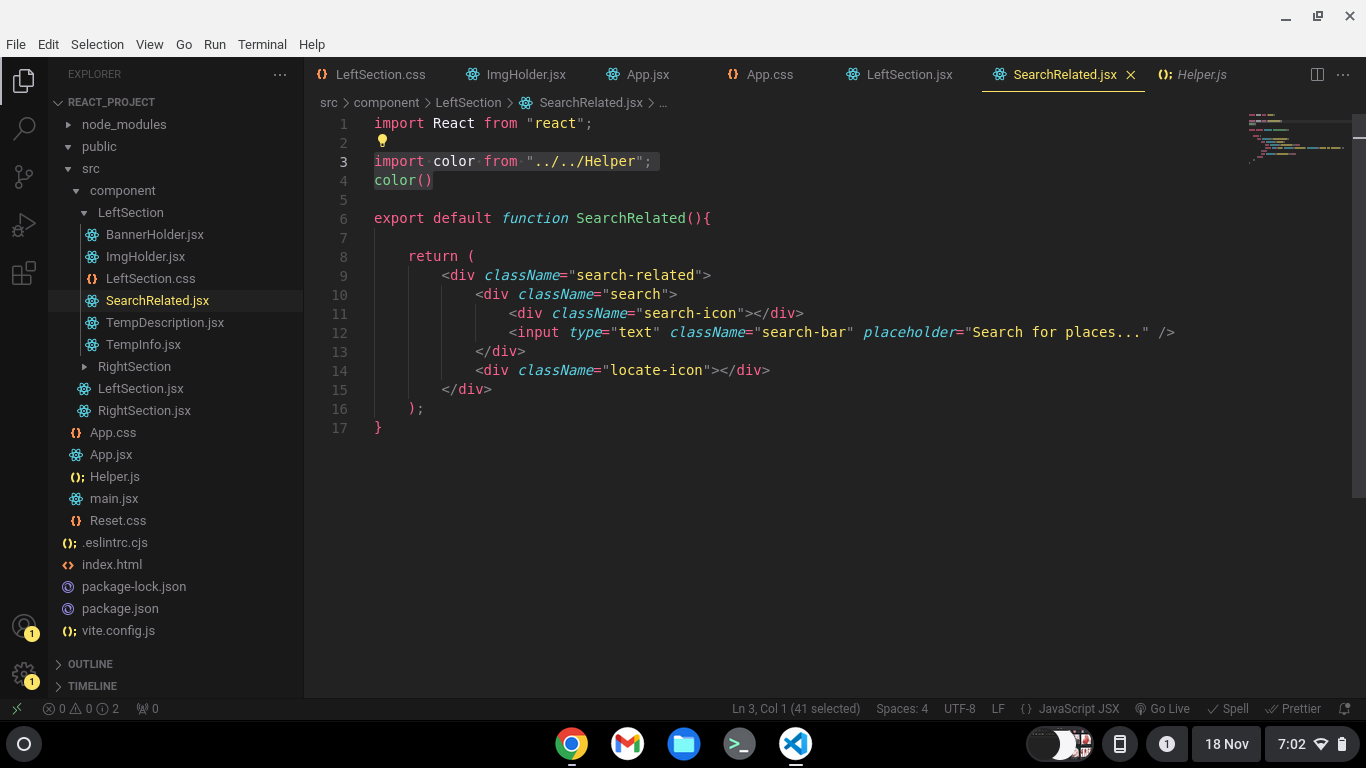
6 replies
KPCKevin Powell - Community
•Created by glutonium on 11/6/2023 in #front-end
can clamp() be used for anything other than font-size
well I know what clamp() function is or what it does
I've only seen people using it for font-size but i wanna know if it is possible to use it anything else
for example, margin, padding or gaps
I also know about min() max() functions that we can use but i once used clamp() for gaps and it worked quite amazing
but since i never saw anyone using it , I'm sort of confused if it's bad or unorthodox to use it for anything other than font-size
tnx ❤️
5 replies
KPCKevin Powell - Community
•Created by glutonium on 9/22/2023 in #front-end
random error on console
k so i was coding and things were fine but all the sudden console started showing the following errors
i thought could me my code and i eventually ended up commenting my whole script but the error is still there.
anyone know how to fix?
here's the pen in case for the need of code
https://codepen.io/-bloop-/pen/RwEQgrY
6 replies
KPCKevin Powell - Community
•Created by glutonium on 5/25/2023 in #front-end
Bond with the nearest ball.
https://codepen.io/-bloop-/pen/jOeJzqr
in this given codepen, u can see there's 30 balls each with their respective x and y velocity.
the intention of the code was to make so that each ball forms a bond with it's nearest ball. But apart from the first ball, all of the balls don't actually create a bond with it's nearest one unless a ball is very very close to it. Does anyone how why it happens or how I can tackle this?
I've added descriptive comments in my code so it'll be easier to understand what each codeblock does. tnx ❤️
3 replies
KPCKevin Powell - Community
•Created by glutonium on 5/18/2023 in #front-end
trying to solve css
so if I have
and I do
doing
would work but if I do
it doesn't work. why?? don't they both change the value of the variable?
39 replies
KPCKevin Powell - Community
•Created by glutonium on 5/18/2023 in #front-end
how can i make it smooth on mobile
the pen
https://codepen.io/-bloop-/pen/KKGGYpj
now it's the backdrop-filter that's causing the problem.. How can I make it smooth??
20 replies
KPCKevin Powell - Community
•Created by glutonium on 5/18/2023 in #front-end
does it work for unsotred array?
since it's checking if
arr[middle]
is less than or bigger than the target if u given unsorted array for example
and we r looking for 3 then
it'll first land on 1, then check if 1 is smaller or bigger than 3. Since it's smaller than 3 it'll look on the right side but 3 in on the left side.
so does that mean it'll only work with sorted array or am I getting something wrong??
i do know how to sort an array btw xD41 replies
KPCKevin Powell - Community
•Created by glutonium on 5/18/2023 in #front-end
Binary Search vs For loop
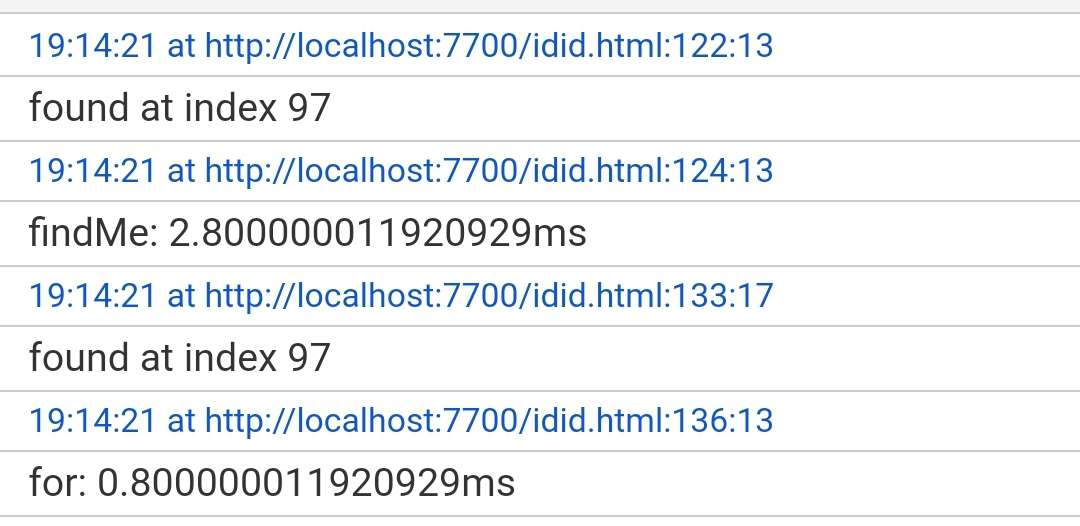
6 replies
KPCKevin Powell - Community
•Created by glutonium on 5/18/2023 in #front-end
loops and forEach
if i have for example ,
u can at any point i can stop the loop from doing any further loops using break
but if I have something like,
is it possible to add something similar to
break
in the if condition that'll stop the forEach from doung further operation on the items from arr2??11 replies
KPCKevin Powell - Community
•Created by glutonium on 4/24/2023 in #front-end
hop issues
https://codepen.io/-bloop-/pen/MWPprJV
so i basically just have a simple arc.. everytime u click it is supposed to hop.
which it does, but the problem is, if u keep clicking, it seems as if with every click, it hops higher and higher, and doesn't hop the same height. why is it happening and how can I tackle this problem?
also currently, when u tap it just teleports to the calculated position, i would like it to gradually go up like it does when it comes down.. how can I achieve that as well?
tnx❤️❤️
1 replies