Black_Wither
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 5/11/2024 in #djs-questions
Wrong user count
Every time that I run the command again, the users var will increase insane, for example every second +10 members. The first where I use the command after every restart, it will give me the correct userCount. Can anyone tell me how to fix that?
14 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 3/29/2024 in #djs-questions
Locale Descriptions
Hey I'm currently trying to use locale description with my bot. I have following code which is not working:
`
imports:
dependencies:
4 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 3/1/2024 in #djs-questions
MessageCreate API
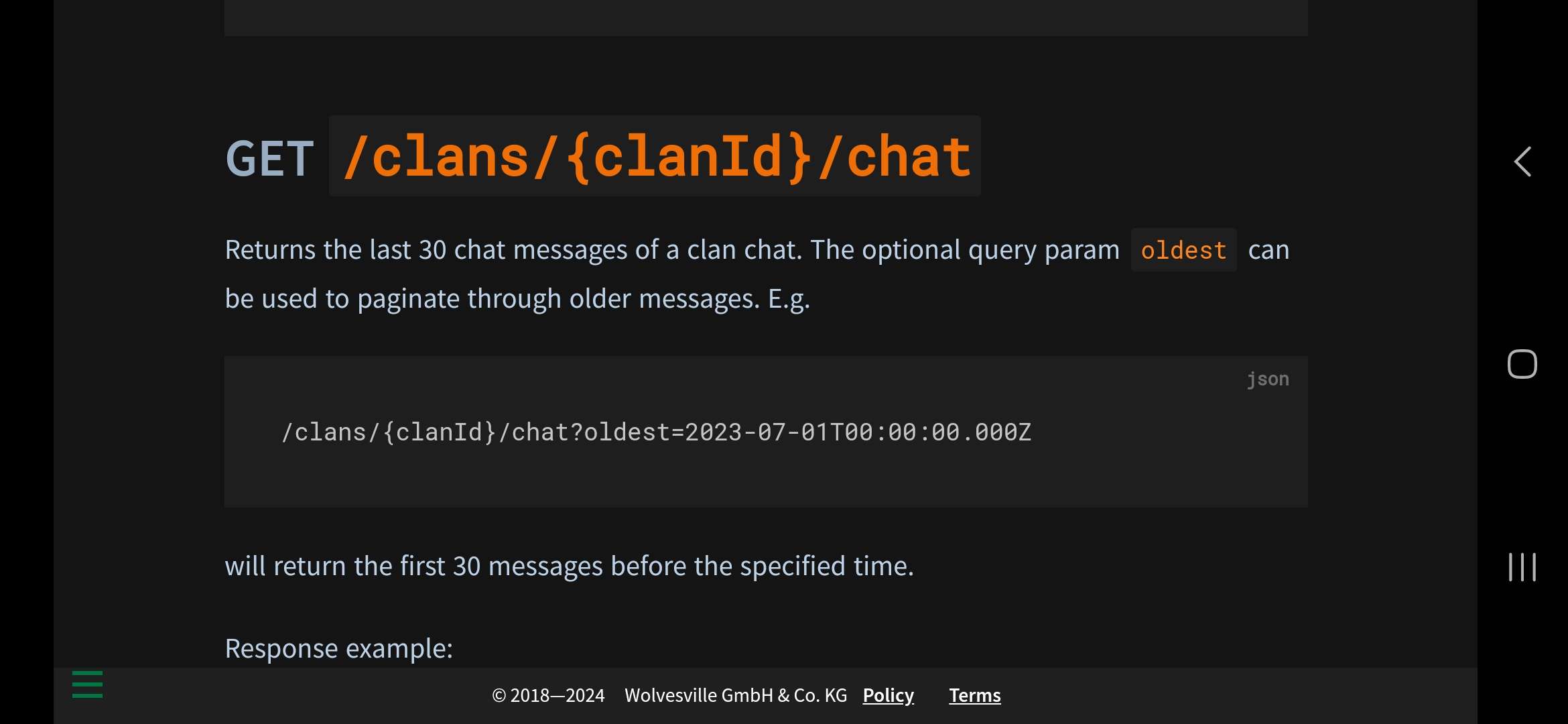
5 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 1/8/2024 in #djs-questions
Canva Image to .setImage()
How can I add a @napi-rs/canvas image to a embed as image in a .setImage function?
9 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 10/15/2023 in #djs-questions
Can‘t find message by id
I can‘t fetch a message by his id.
The error:
const clockInMessage = await user.messages.fetch(worker.clockInMessage);
3|segritude | ^
3|segritude | TypeError: Cannot read properties of undefined (reading 'fetch')
9 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 8/3/2023 in #djs-questions
REST dont make Slash Commands
My guild Slash Commands dont register any more
A few days ago, all was still working. But I added a context menu handler to my bot with a similar code what I has taken for my guild Slash Commands.
My problem is, that the context menus are still working after deleting the context menu handler file and the slash commands dont register.
12 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 7/28/2023 in #djs-questions
create context menus
I get no error, but no context Menus are created after start the bot:
6 replies
DIAdiscord.js - Imagine an app
•Created by Black_Wither on 5/27/2023 in #djs-questions
Create Forum Tags
Hey, how can I create Forum Tags? I try it on discord.js
8 replies