Hamza Ali Turi
TTCTheo's Typesafe Cult
•Created by Hamza Ali Turi on 8/27/2024 in #questions
Integrating AI assistant (fastapi/react) on the main website(laravel).
I’m currently developing a chatbot using FastAPI, LangChain, and React and am looking to integrate it into my Laravel application. I initially tried placing the React app’s build folder into Laravel’s public directory and created a route for it. While this approach works, I’ve encountered an issue: when navigating between routes, the chatbot also refreshes. This results in users having to wait for the chatbot to reload every time they switch routes, which is not ideal. Is there a better way to ensure the chatbot remains loaded and accessible throughout the entire site without having to reload each time the route changes?
3 replies
TTCTheo's Typesafe Cult
•Created by Hamza Ali Turi on 8/22/2024 in #questions
Need help in TipTap
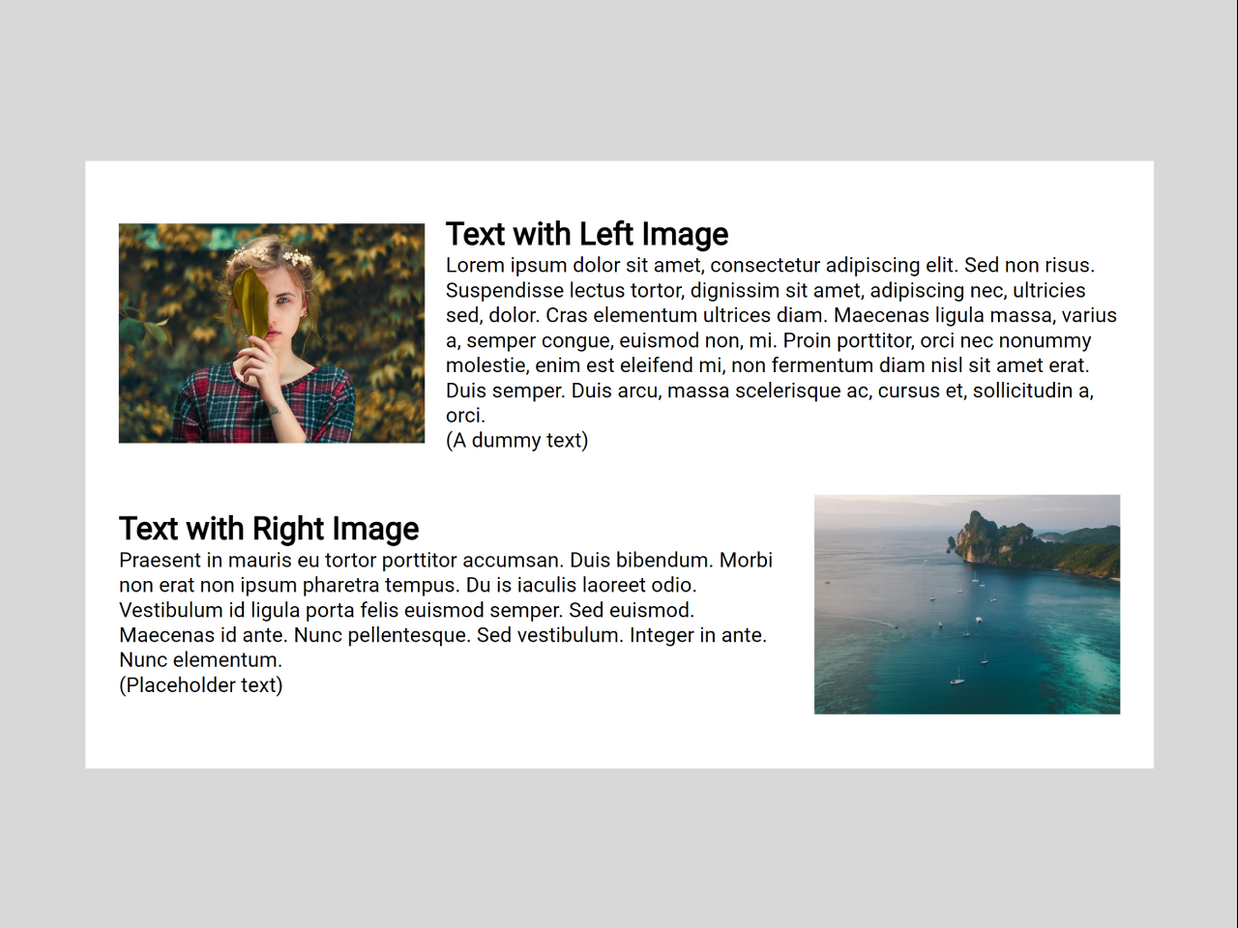
5 replies
TTCTheo's Typesafe Cult
•Created by Hamza Ali Turi on 12/24/2023 in #questions
Keeping the text data in a separate file.
I have this code that where I am mapping JSON data in the Accordian component. I was wondering whether is it good idea to do that or should I not bother with converting my text into JSON and then mapping it to a component. I do this practice because I think it makes the code look clean but now I am wondering is it really worth trouble?
2 replies
TTCTheo's Typesafe Cult
•Created by Hamza Ali Turi on 12/6/2023 in #questions
Help Needed: Code Review.
2 replies
TTCTheo's Typesafe Cult
•Created by Hamza Ali Turi on 11/24/2023 in #questions
Session & Cookies
Some time ago, I started backend development with node.JS, express.JS and mongo DB, I was completely lost on how everything works, over the months I have built a small understanding of how things work. I can now build a somewhat basic backend.
The above paragraph is for you to understand where I am coming from.
Right now I am figuring out how session and cookies work in a website using react.JS, prisma, express.JS, passport.JS, postgresql and node.JS (before for auth, I would use JWT tokens), When learning them, I went through the internet and came across explanations on their usage. They told me this, when logging in you have to store sessions in the database like this.
and when logging out first destroy the session from the machine with this code.
and then delete the session from the database, So lets suppose I use something like
So here is my question, when deleting the session from the database do I manually have to delete them like above or is there some inbuilt code for handling that, without using prisma. Also Some recommendation for backend topics will be appreciated.
6 replies