sproj003 ♿
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 5/16/2024 in #djs-questions
React to message with emoji
I know it's probably something really simple but my bot sends a message not in response to an interaction. It should then react to that message with random emojis.
What am I missing?
9 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 5/7/2024 in #djs-questions
Bot permission in channel
I'm trying to get my bot to check if it has permissions to send a message in a channel. It will mainly be for text channels but also in forum posts and voice channels. Not sure what I'm doing wrong. I'm getting the IDs for the guild and channel from a database.
6 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 1/17/2024 in #djs-questions
get application commands
As part of the js file that registers my commands, I want to get the existing commands before registering the new one. This is for both global and guild commands. There is no connection to the client in this file and I'm using @discordjs/rest.
11 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 12/6/2023 in #djs-questions
View Guild Onboarding
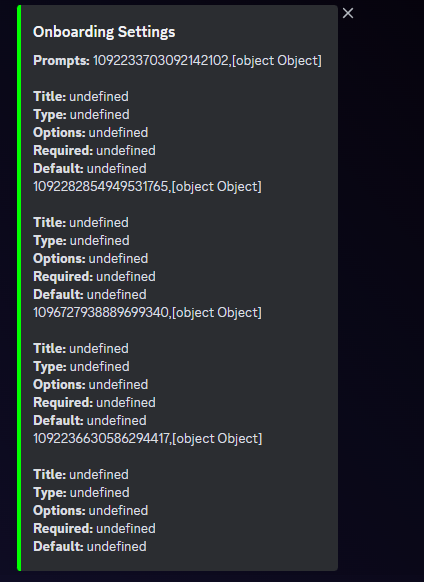
7 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 11/22/2023 in #djs-questions
Set GuildScheduledEvent image
What's the easiest way for me to add/change the cover image of a Guild Event?
4 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 11/8/2023 in #djs-questions
SelectMenuBuilder Union Types
I'm attempting to send a SelectMenu in a message but I'm getting this error
My code is in the attachment
When I remove the SelectMenus, it works fine
14 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 8/24/2023 in #djs-questions
Slash command channel types
Is it possible for me to filter out channels that are shown in the options by type. Here's my code:
Is it possible for me to filter the channels shown to just channel types text channels and voice channels but not forum channels?
8 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 5/5/2023 in #djs-questions
Not enough sessions
I got this error when starting my bot
What does this mean?
4 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 2/22/2023 in #djs-questions
Voice channel reactions
Is there a way for me to detect when a user uses reactions in a voice channel. Not the chat in the voice channel. Some kind of event?
5 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 2/21/2023 in #djs-questions
slash command string limit
Is there a limit to how many characters I can put in a slash command string option?
5 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 1/16/2023 in #djs-questions
use other bot slash commands
When I set up the application on Discord, I was able to let it use other bot's slash commands. How do I use this in Discord.JS?
8 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 1/10/2023 in #djs-questions
autocomplete not working
Autocomplete won't show in discord
12 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 11/7/2022 in #djs-questions
Get all users who have a role
I'm trying to get a list of User IDs for everyone in a server who has a particular role. Here is my code.
Camps is a json file
58 replies
DIdiscord.js - Imagine ❄
•Created by sproj003 ♿ on 10/11/2022 in #djs-questions
Message ID of first message in channel
I want the message ID of the first message in a channel to use as a link as a bookmark. Is this possible?
5 replies