RedRoss
Explore posts from serversPPrisma
•Created by RedRoss on 11/11/2024 in #help-and-questions
How generete enum type from hasura?
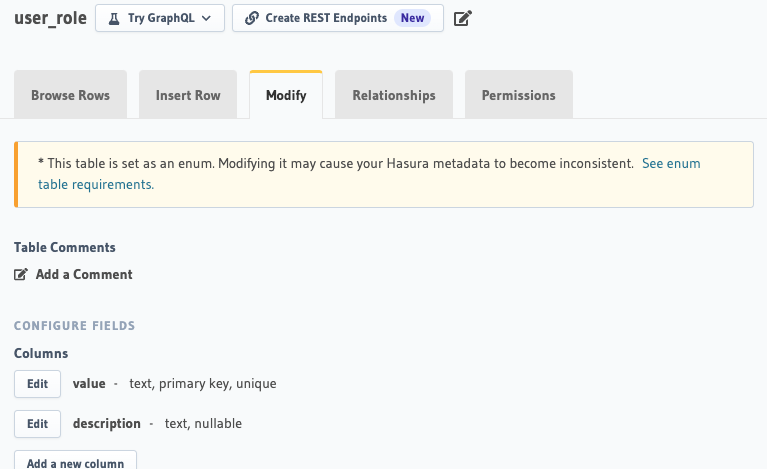
10 replies
PPrisma
•Created by RedRoss on 10/23/2024 in #help-and-questions
how can I implement an abstract class to apply the repository pattern?
I use nestjs and would like to implement the pattern repository as it is used in typeorm
5 replies
CDCloudflare Developers
•Created by RedRoss on 2/22/2024 in #pages-help
How do I generate the mp4 link to watch my video that I uploaded on cloudflare stream?
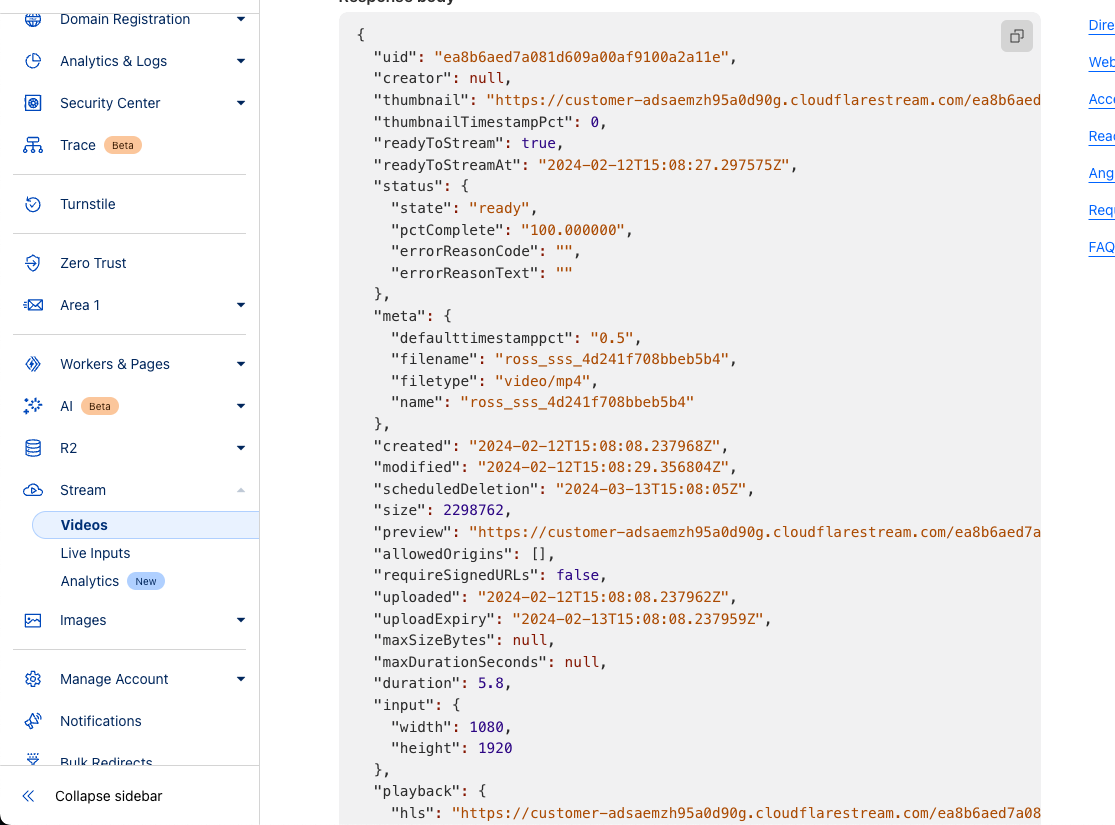
3 replies
CDCloudflare Developers
•Created by RedRoss on 2/8/2024 in #general-help
how can I retrieve the url when I upload a video with tus client js?
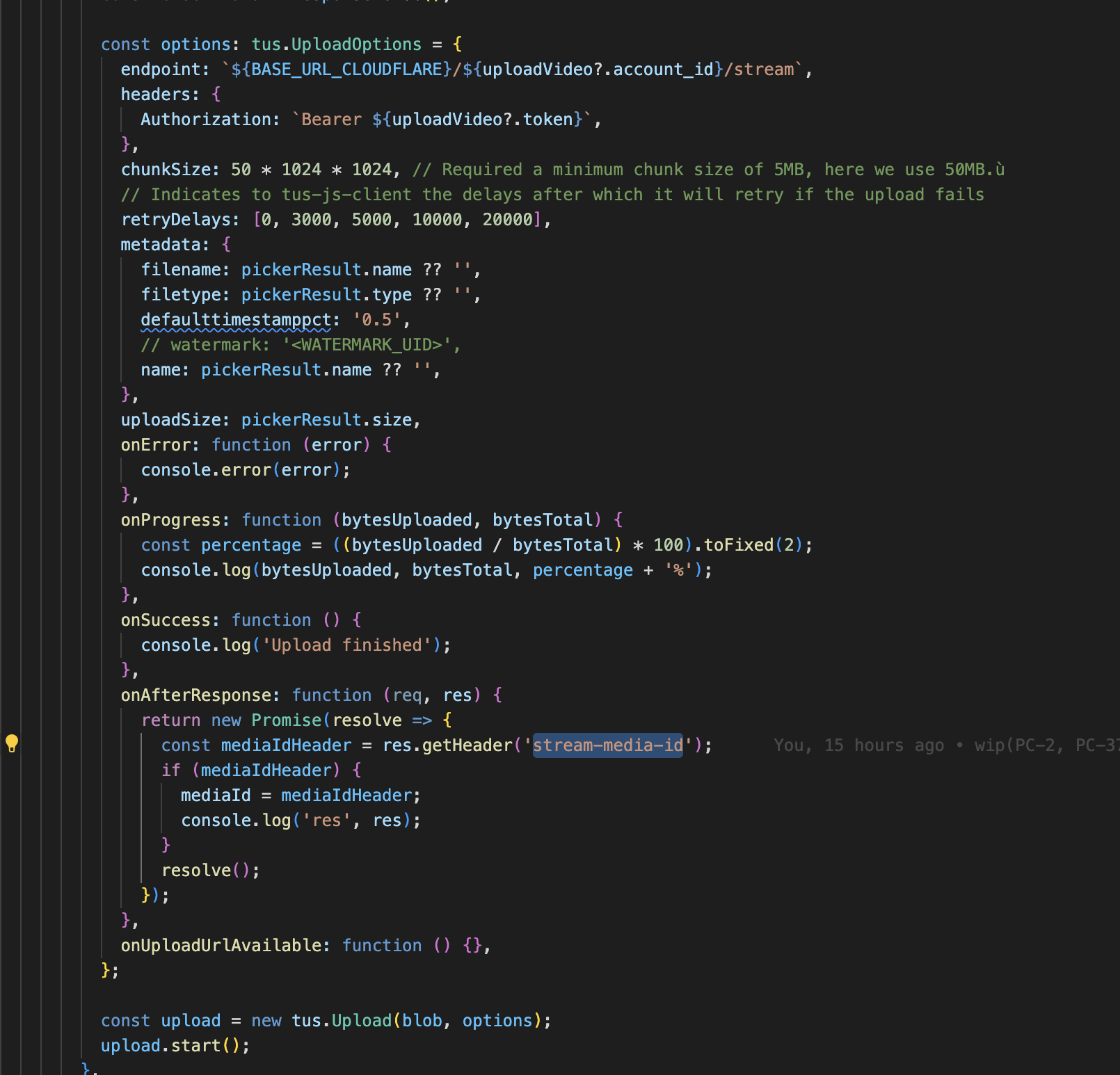
1 replies
CDCloudflare Developers
•Created by RedRoss on 1/25/2024 in #general-help
With react native what are the steps to take to upload a cloudflare video?
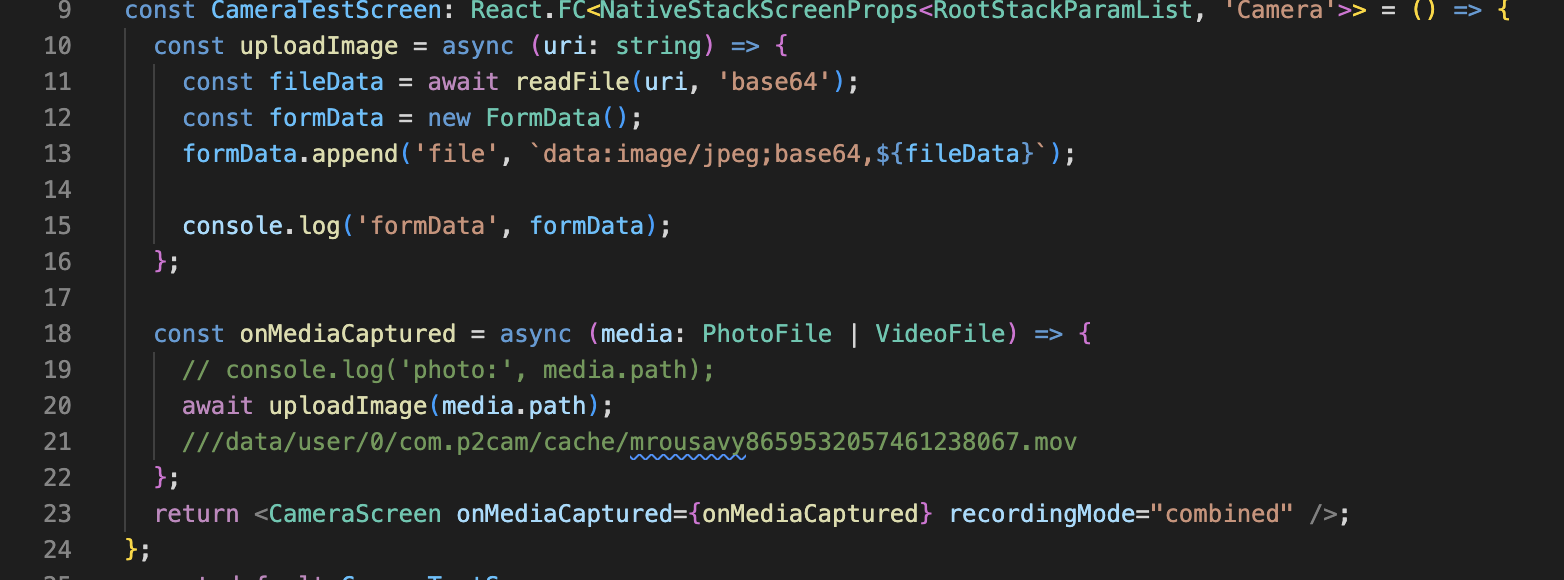
2 replies