Maskoe
Access files in a docker image
I am completely defeated.
All im trying to do is access a file with type = content and output = preserver newest in docker
this is my dockerfile
I tried all kinds of different combinations and debug logs for about 10 hours
I cant find any combination that works. I just want to access this file. I can see its in the docker image.
With the debug logs im getting the correct path, its just not there if I do File.Exists. If I check locally or in prod within the terminal of the image, its there.
this is in my csproj
7 replies
Does Hangfire create a new instance of my aspnetcore api?
Based on the documentation and some tests I did, it doesnt, but I'd like to be sure.
I dont trust the docs and myself too much in this case.
https://docs.hangfire.io/en/latest/index.html
These method invocations are performed in a background thread and called background jobs.So it doesnt create a a new instance of my webapi every time it executes a job right? What I played with was this So this actually does put out 1 2 3 4 ... So it IS the same instance of my webapi, which I can tell by the singleton service. If it was a different instance, it would start at 0 every single time. Now you should never do this, BUT that means hangfire jobs actually do work with application state somewhat. Obviously you cant guarantee your app hasnt restarted and hangfire executes a job right after. Even in FireAndForget scenarios. 🤔
3 replies
Razor MVC UI reuse
I cant believe that I cannot figure this out, but how do I create the equivalent of a react or blazor component in MVC Razor?
I have this code
That I want to turn into <SimpleEditor asp-for="PimId/> or something similiar. But I just cant find the right direction.
ViewComponents? TagHelper?
I think its more difficult because these are ModelExpressions. PimId comes from the Model of the original component. This has to be one of the most common reuse patterns to ever exist, but there is nothing online.
4 replies
Manually execute default ModelBinding from AspNetCore
For reasons I am in an ActionFilter and want to transform the
IFormCollection
I have into a model class.
This is basically ModelBinding, I want to avoid writing a bunch of reflection and typing stuff myself.
Where is this logic? I found Microsoft.AspNetCore.Mvc.ModelBinding.ModelBindingHelper
, but I dont have access to it somehow.
I basically just want something like ModelBindingHelper.TryUpdateModel(myForm) or my HttpContext
or my ActionExecutingContext
.
I know I have A model in my ActionExecutingContext
, but its not the one I need. Basically I want a simple way to bind form data to a model and I know aspnetcore does this internally. Where is this code? Can I use it? Can I steal it?1 replies
❔ Coldstart issues?
I've been working on my own very small projects and MVPs and noticed something common to them.
I seem to have insane cold start issues for the most mundane things.
I'm talking sending a request to an endpoint, and creating a row in a database via ef core.
This is especially noticable when the app is hosted in azure. My usual setup is an app service and a Azure Database for PostgreSQL flexible server using aspnetcore and ef core.
Its only ever the first request that is slow. It happens locally too, just far less noticable. The first request to my endpoint is 225ms, the same call 2 minutes later is 16ms. Thats more than 10x faster.
What could cause this? Especially hosted since im using an app service and a database server. There is nothing serverless here.
I have a simple request taking 2 seconds. Its very noticeable. It will be <500ms after the first one is through.
Eventually (hours? minutes?) it seems to reset back and the first request is back to taking 2 seconds.
I got detailed ef core logging. The queries, locally, are executed in 1-7ms. So it doesnt seem to be the actual db.
What is this? 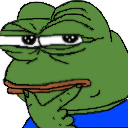
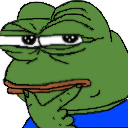
21 replies
❔ EF Core optional where extension method
I have a lot of optional parameters for queries. Filter by this, filter by that etc.
I can do it like this
would like to avoid the null check or part every time and write something like
context.table.Where(x => projectId == null || x.ProjectId == projectId)
(This actually doesnt even add a WHERE TRUE to the generated SQL Query 
.OptionalWhere(x => x.ProjectId, projectId)
but I cant find the correct syntax for the extension method.
Im trying stuff similiar to this
Func cant be translated. Expression cant be translated if I compile it. I guess because it turns into a func..
8 replies
❔ Rapid prototyping
Do you feel there is such a thing as rapid prototyping or mvp development where you do things different than at work?
I've been trying a couple of my own things lately and been cutting corners to just get the absolute minimum up and running.
Stuff like returning ef core entities directly instead of dto's / response models. But I run into issues sooo fast, making me go back to dedicated response models within like the first 20 hours.
Yea i can stop the serialization loops with a line of code. Yea I can ignore the silly swagger definitions it leads to, I know the models after all, i just made them up yesterday.
I feel like it actually slowed me down overall. Yea I feel silly for 2 minutes literally copy pasting the entity class to new class, removing 3 properties and returning that one, but I know it wont stay that way. It never does.
I also have a feeling that if there was actually something that would make development faster, no matter the tradeoffs. We would all be doing it at work...
2 replies
❔ Trying to generify List Func Task
Im trying to generify the following code.
I think my intention is clear, I have several ways to query contracts that are prioritized a certain way. If i dont find anything in the first priority list, check the 2nd, if i dont find anything, check the 3rd and so on.
I got this so far
I can call it like this
Are there any issues with this? Does this work simpler?
Working with tasks and lists of tasks and async await is always kinda dangerous. But it seems to work.
The
GetContractsPrioX
methods fire only if needed. If I have an exception in one of them, everything seems to behave normally, nothing gets swallowed.
I would like to remove the () => part, but I dont think its possible. If I remove it and just work with the GetContractsPrioX
methods directly, the tasks naturally start executing instantly.4 replies
❔ Manual route/template matching exactly like blazor
I want to take what blazors INavigationManager gives me, which is the ACTUAL uri like this: "/jobadmin/23" and find my @page route TEMPLATE based on it, which looks like this "/jobadmin/{JobNumber:int}".
I found this post https://blog.markvincze.com/matching-route-templates-manually-in-asp-net-core/
and played around with the code, adjusted it a little and now I got the following:
This seems to generally work.
I only found one "issue", when I set the requestPath = "/jobadmin/ff", it still matches.
I dont know if it should. Its not an int, but it still matches... internally aspnetcore probably just handles that part later. Its fine for me that this is a "match", however it makes me think to make sure.
My question is basically, does this work? Does this do what I think it does?
2 replies
❔ Smart way to code an insurance pricing calculation with explanation?
I'm refactoring a ~60% correct pricing method. To give you an idea its currently like 1000 lines of c# code, 1000 lines of sql queries and maybe about 7 involved tables.
The code and math is actually not heavily complicated. Its just a LOT of
"if there is a contract for this item in that table, its prio1. Otherwise look for an old contract in the other table with column = true, thats prio2, column = flase is prio3..."
"If there is a discount, apply the discount from that table, but only up to this total"
The heaviest mathemathical operation is actually just percentages.
Its important for users to see how the app ended up at the final number.
All I can think of is basically doing it manually. For every line of math, i just add an explanation after like this:
if(contract.AllowDiscount && discountTotal < allowedDiscount)
price = price * (1-contract.Discount)
Console.WriteLine("Discount is below {allowedDiscount}. Reducing price by {contract.Discount}%")
16 replies