DINO
Explore posts from serversTTCTheo's Typesafe Cult
•Created by DINO on 12/14/2024 in #questions
Is t3dotgg/yerba the best way to use electron in a monorepo?
Hello,
I have came across a lot of templates that implement an electron app in a turborepo, and
t3dotgg/yerba
was one them.
What I noticed is the lack of issues and maintenance on the templates so I thought I should ask if it's still good to use it or are there any better ways to have an electron app in a turborepo/monorepo project.
Thanks.3 replies
RReactiflux
•Created by DINO on 11/13/2024 in #react-forum
How do I handle authentication state in a SPA react app?
Hello,
I have implemented authentication in my backend that uses http only cookies, so now I am not sure how do I handle authentication state in a SPA react app.
When the user logs in I would like to store the auth state in a store like React Context or Zustand, but what if the user closes the app and opens it again? Should I hit an API route like
getUser
every time he opens it and if it returns the user that means he is sill logged in?
Should I use Provider component (React Context) that wraps the whole app that checks the API?
I would like to use a HashRouter
instead of a BrowserRouter
in my app.
Thanks.5 replies
TTCTheo's Typesafe Cult
•Created by DINO on 11/1/2024 in #questions
Hello how do I do authentication without Next-Auth or any other auth library?
Hello,
I would like implement a session based authentication in my t3 app but without Next-Auth or any auth library, this might be a dumb question but I really don't know where to start.
I know how to do it using Express, or Fastify though but i don't how to do with this stack and tempalte, is it using Express behind the scenes so I need to use
express-session
or what.
Thanks.10 replies
RReactiflux
•Created by DINO on 9/29/2024 in #react-forum
I would like implement multiple tabs navigation inside my React app, where do I start?

12 replies
How do I implement API key authorization along with JWT authorization in tRPC?
Hello,
I want to make some routes protected and to be accessed by either JWT auth or by API keys auth. So I check both auth methods in the same context.
To make things clear i will just share the code of my
context
:
I am using Fastify.js. What do you think of this approach?
Thanks.2 replies
TTCTheo's Typesafe Cult
•Created by DINO on 7/17/2024 in #questions
Uploadthing error "An error occured while parsing input/output" when I try to upload with Insomnia
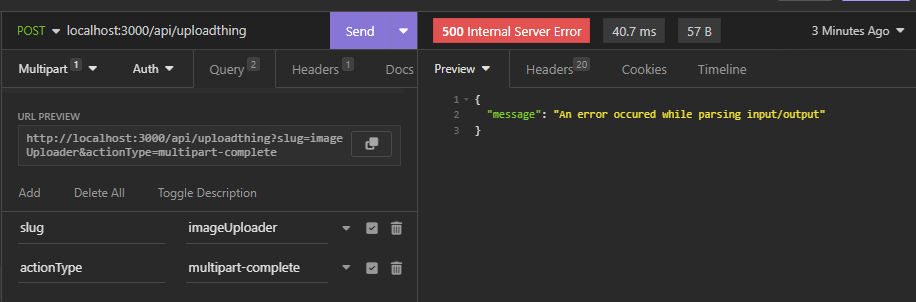
20 replies
Is to possible to update the trpc client url?
Hello,
I have create my trpc client this way:
Is there a way i can change the url in the httpBatchLink when the app is running? I tried to create and override the old instance but it didn't work.
Thanks.
2 replies
How do I set header value from localStorage or Zustand in tRPC and React?
Hello,
This is my tRPC client in my
App.tsx
:
The problem is if the jwt token changes, it doesn't get changed in the trpcClient unless I do something like this but I don't like it:
What is the best way to set jwt to trpc client?2 replies
What do you guys think about the approach of importing/exporting tRPC types to be used in separate B
Hello,
I was looking for a way to share tRPC API between multiple electron apps, I thought first about using a
Nx monorepo
but it turned out it's almost impossible to make it work with electron app that use electron-vite
.
After more research I found this repo: https://github.com/mkosir/trpc-api-boilerplate
What I understood from this approach is you export the types of the tRPC server with all db types from the backend repo and you import them in any other -frontend- repo using GitHub API (Octokit).
Is this approach common in general programming environments and what do you think about it?
Thanks.2 replies
RReactiflux
•Created by DINO on 4/14/2024 in #react-forum
How do I query data with Tanstack Query if one of the query params can be undefined.
Hello,
I have an
id
variable that can be undefined because I get him from useParams
, and I use the id
value to query data with Tanstack Query, but Tanstack Query requires to have a value that cannot be undefined which is very logical. So I had to do Number(id) || 1
and enabled: !!id
this way:
Is this a common practice? And is there any other way to do this?
Thanks.4 replies
RReactiflux
•Created by DINO on 4/5/2024 in #react-forum
Make Zustand store scalable
Hello,
This is my
Zustand
store state type:
In the future I will add more invoices (up to 10), and I kind of don't like doing something like:
Are there any common practices to handle such a thing?
Thanks.10 replies
useMutation() runs 3 times
Hello,
I have this weird problem that all my mutations across the app runs 3 times I don't know why.
This is my API in the backend:
And this is how I use them in the client:
I am sure that the call from the client doesn't run 3 times. I checked it with console.log.
I would be grateful if anyone helped. THanks.
14 replies