conniehunt
Explore posts from serversPPrisma
•Created by conniehunt on 7/6/2024 in #help-and-questions
NestJS + prisma
Giving nestjs and prisma a go together for the first time but can't get it to run a migration or db push
when I run
npx prisma migrate dev
or npx prisma db push
the process just freezes3 replies
TTCTheo's Typesafe Cult
•Created by conniehunt on 9/8/2023 in #questions
T3env: Module not found: Can't resolve '@/env.mjs'
Hi, I am trying to use T3env for the first time but i keep getting this error in build
Module not found: Can't resolve '@/env.mjs'
. My env.mjs is in my src folder.
here is my env.mjs file:
Very confused, since ive followed the documentation exactly aside from including import "./src/env.mjs";
in my next.config.js since I can't use imports outside of a module2 replies
TTCTheo's Typesafe Cult
•Created by conniehunt on 5/27/2023 in #questions
NextJS(13) API call cache MISS
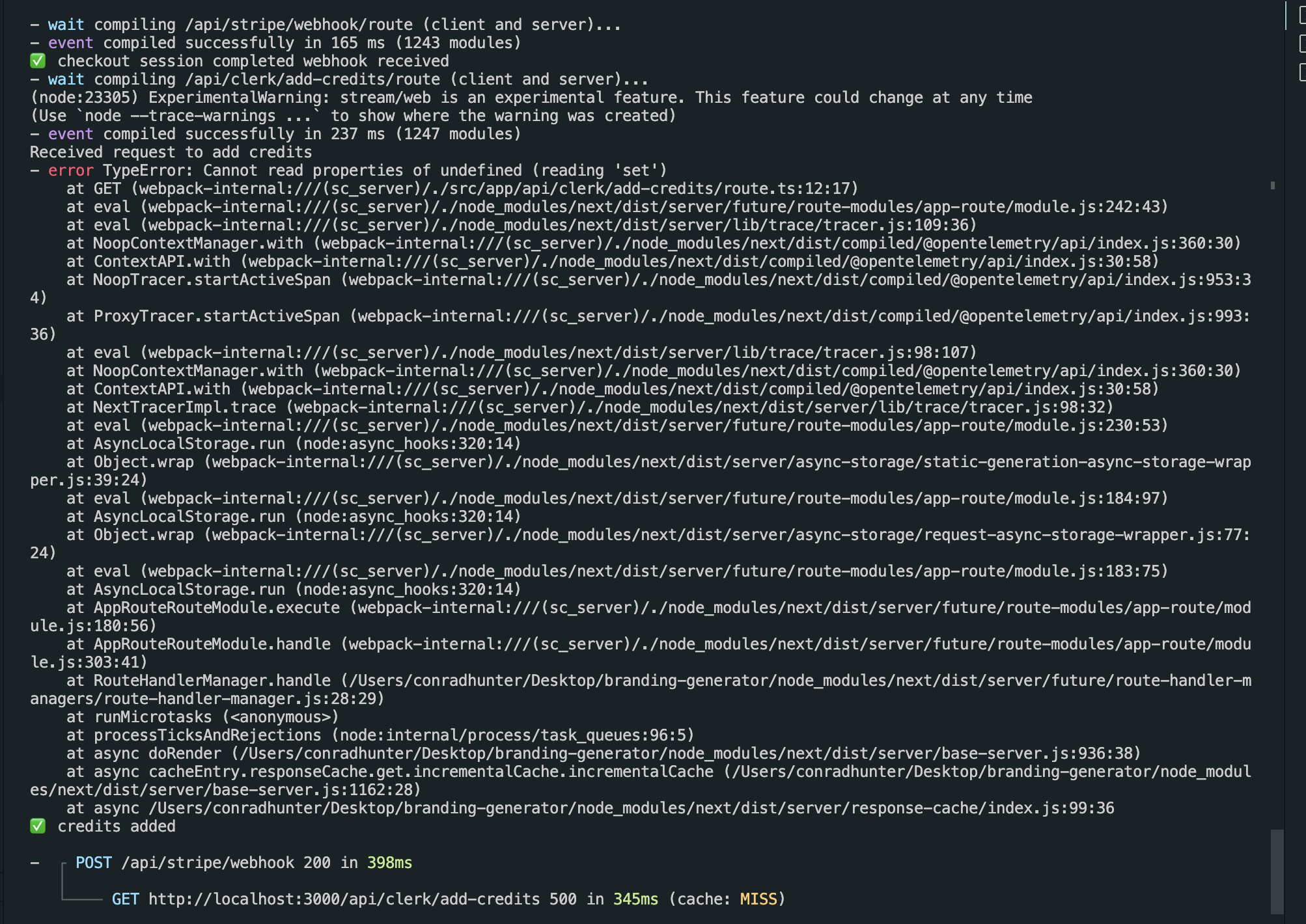
10 replies
TTCTheo's Typesafe Cult
•Created by conniehunt on 5/21/2023 in #questions
API route call from another API route
hoping someone can help me here. Below is my NextJs(v13 app dir) Api route which Stripe forwards webhooks to, when the event.type of "checkout.session.completed" is sent i want to call another API route (add-credits).
When the call to add-credits route is made i get status code 200 but it says (MISS) in console
2 replies