Nacho Elias
Explore posts from serversZod formatting Error
I'm using Zod for validation and whenever I get a validation error I'd like to be able to get the error message properly formatted. In the docs there's a section for this where it's sepcified that we can use the errorFormatter to achieve this. However, it's not doing anything at all. Btw, if I add a console.log within the errorFormatter nothing's being shown in the console, which is kind of weird if you ask me. However superjson is working completely fine, so I dont think its a configuration problem....
Here's my code
This is my validation
And this is what I get in my client in error.message instead of "Invalid ID"
4 replies
DTDrizzle Team
•Created by Nacho Elias on 6/18/2024 in #help
Many to many flatten response
I'm struggling to understand why does drizzle work this way and why there isnt an easy way to flatten the response structure. I have 3 tables, Profile, Interest and Language. I also have 2 other join tables, ProfileInterest and ProfileLanguage. As you may have noticed, I have a many-to-many relationship between Profile and Interest and between Profile and Language. All 5 tables are in place (3 "models" and 2 join tables). I also have all the relations created and everything works as expected. To query for a specific profile including its' interests and languages I run the following.
The problem? The response looks like this
And of course, I'd like the following instead
Is there a way to achieve this using the queryAPI? At the moment the only thing I can do is either use it this way, which is not the end of the world but very inconvenient, or I can just flatten the structure in my server, which is also quite inconvenient...
2 replies
Zod error not being formatted
I'm using Zod for validation and whenever I get a validation error I'd like to be able to get the error message properly formatted. In the docs there's a section for this where it's sepcified that we can use the errorFormatter to achieve this. However, it's not doing anything at all. Btw, if I add a console.log within the errorFormatter nothing's being shown in the console, which is kind of weird if you ask me. However superjson is working completely fine, so I dont think its a configuration problem....
Here's my code
This is my validation
And this is what I get in my client in error.message instead of "Invalid ID"
2 replies
DTDrizzle Team
•Created by Nacho Elias on 6/13/2024 in #help
Error in DB update because of Date
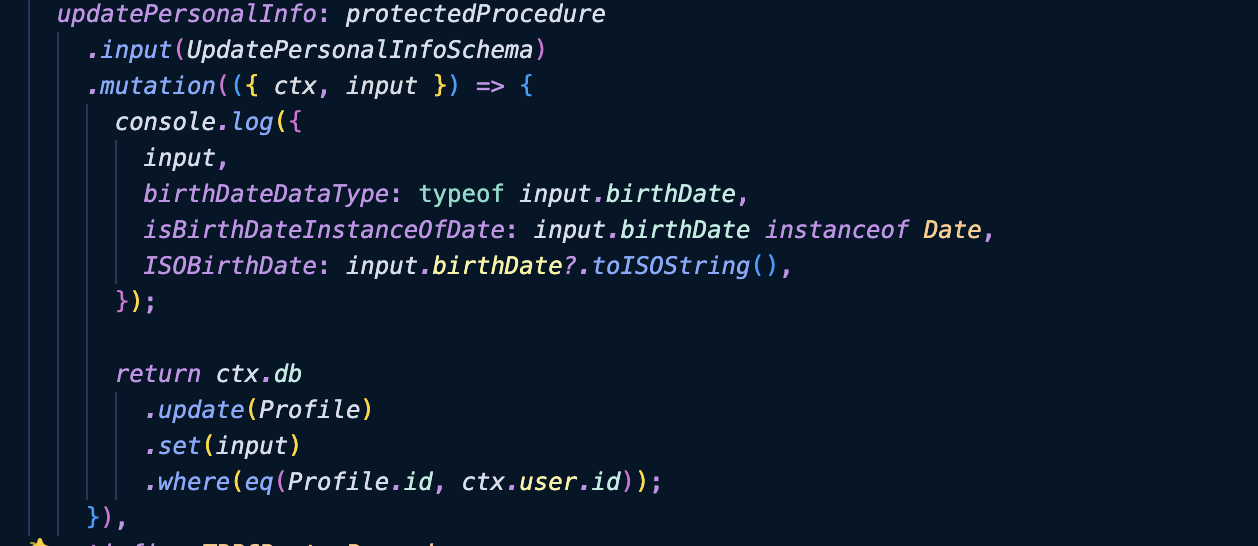
2 replies
Which http library trpc uses in the frontend?
I was wondering if
trpc
uses the Fetch API
or uses something like Axios
to do API calls from the frontend. I recently added supertokens
to an app and I need to add a middleware for session refreshment depending on the tool I'd be using3 replies
useQueryClient is not working as expected
So before v10 I was simply using useQueryClient and had my
queryClient
strongly typed. Now I moved to v10 and for some reason, the documentation says that the queryClient was removed from the context so I should use the useQueryClient hook directly from @tanstack
but if I do so then the queryClient returned by that hook is not the queryClient trpc
is using, it's just empty. I made it work by using the trpc.useContext
hook coming from my client utils but I definitely think that the docs are a bit f*cked up.71 replies