blockgoblin31
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 10/31/2024 in #java-help
How would I have a thread wait on a lambda?
This is my first time messing with threads. The project takes user input with a lambda, so I need to wait until that lambda gets valid data to resume the thread. From my research I havent found a good way to do that other than
while (var == null) {}
which doesn't seem ideal.55 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 7/27/2024 in #java-help
Why/how is displayMap null
I have a class here and it seems like nothing in the constructor is running except the super() call. None of the prints run and when repaint is called it throws an error for displayMap being null. What is wrong?
5 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 7/11/2024 in #java-help
How to add blank lines to the end of a Document?
I'm working on a text editor and it needs to copy some text from one JTextArea to another at the same line, but when one adds a line I can't copy it over with the code I'm using because the document doesnt have enough Elements. My code is
48 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 7/10/2024 in #java-help
Text color not changing
I have a custom class that extends JTextArea, I'm eventually going to use it to copy information between two different views but figured I might as well implement a quick dark mode. The problem is my new AttributeSet isn't applied, and I can't tell why. I've used StyledDocument.insertString() before for setting text and background color(working with a JTextPane then), but here it doesnt seem to work the same way. Do I need to extend a different class?
15 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 7/9/2024 in #java-help
Not sure what even is the problem
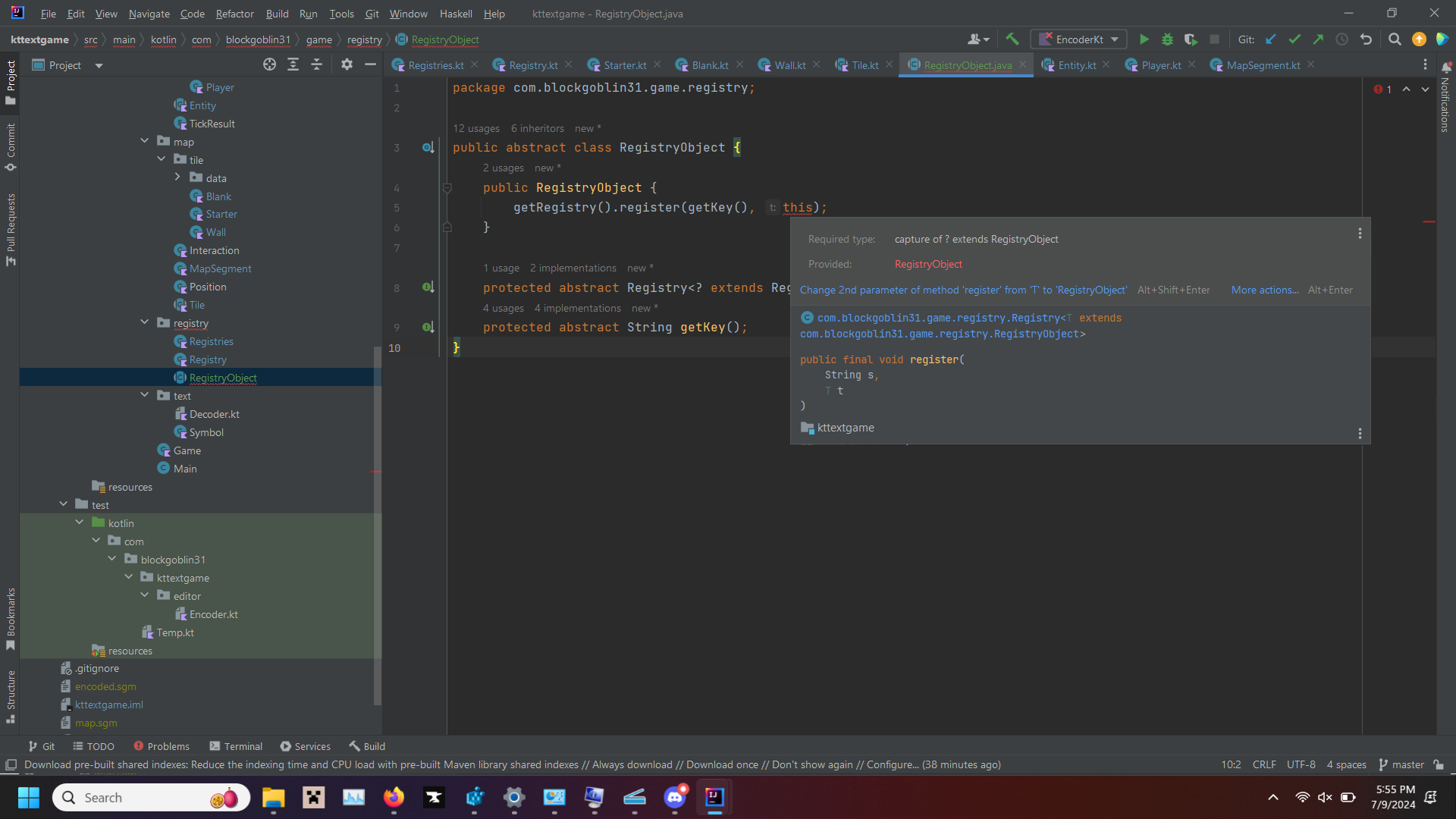
5 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 6/27/2024 in #java-help
Use a Class for a generic type?
is there a way to for example do
In case this is xy problem: I have a class called DataObject for storing some extra data on things, and it has a generic type for what type of data it's storing. There's another class called DataMap that takes its own generic type and extends DataObject with a HashMap<DataObject<T>, DataObject<?>>. I'm then serializing it to json with gson as my method of storing it in a file, but I need a custom deserializer for it, and I'm getting errors for it not being able to convert HashMap<DataObject<?>, DataObject<?>> to HashMap<DataObject<T>, DataObject<T>>. Storing the class name in the json is my current idea for a workaround.
24 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 2/25/2024 in #java-help
How do I append text to a JTextPane?
I've only used JTextAreas in the past, but my current project needs colored text, so I need to use JTextPane or JEditorPane. From googling JTextPane seems simpler but I still cant understand how I actually add text
24 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 2/23/2024 in #java-help
How would I listen for keypresses in a window?
I'm working on a game and want to grab keyboard input while a specific window is focused. It wont have many components, should just be a JTextArea(that can't be edited) though Ill probably add more as I get better with JFrames. How would I listen for the keyboard input? A key listener?
5 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 2/7/2024 in #java-help
Exclude files from jar intellij
I'm working on something that adds on to a project that I can't run easily for testing, so I have some test files that emulate it through command prompt(roughly), and I was wondering how I could exclude those files from the final jar. They're already in a test directory. The main problem is they wont compile when I'm making a build, because I need to change the type from my test class to the actual production class which breaks things in the test class.
30 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 2/6/2024 in #java-help
How would I compare HashMap keys with .equals() instead of ==?
HashMap.getOrDefault compares keys with == instead of .equals, is there an equivalent method or something else I could use to compare the keys with .equals() instead?(I assumed it was .equals and would have to recode about half my project if there isnt a workaround)
12 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 2/4/2024 in #java-help
gson really doesn't like my class, does anyone know why?
its only calling .toString() on it instead of convering it to json it can parse back into an object. Class is attached.
9 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 2/1/2024 in #java-help
How do I input longs
I have my own class that extends Random that I made so I can save the seed and use it again later if something weird happens, but it doesn't let me paste the number in from console. Specifically the error I'm getting is
java: integer number too large
. The number is 3449410621531671124
.8 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 11/28/2023 in #java-help
How does KeyListener work?
I have two(relevant) classes which ive made trying to figure out how this works.
39 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 11/21/2023 in #java-help
Check for a specific generic type
I have a variable of the type Supplier<? extends Item> and want to check if it’s Supplier<? extends BlockItem>. Is there a way to do that? instanceOf doesn’t seem to work according to IntelliJ. Minecraft forge 1.16.5 so java 8.
15 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 5/4/2023 in #java-help
Specify generics?
Can I do something like
class ArrayList<String> extends ArrayList<E> {
? I have a class with a generic and I want to override a method if the type is string, so I can use .matches() instead of ==. Being able to check the type of a generic would also work.16 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 5/1/2023 in #java-help
Is there a way to return a different type in a subclass method?
I have this
which seems to not be valid because the method .add(E) in ArrayList returns a boolean. Is there any way I can implement this?
28 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 5/1/2023 in #java-help
Intellij is complaining about this code, not sure why
its saying "variable used in lambda expression should be final or effectively final" (about j), and gives the option to convert j to atomic. Im wondering what that means, and what atomic is. (K and V are generics, this class extends HashMap)
16 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 4/18/2023 in #java-help
I am trying to get the index of the lowest value in a array/ArrayList. How do I do this?
I have an array of length 4(which I can make an ArrayList if that would be easier), and I need to find the index of the lowest value in the array, to remove that same index from an ArrayList.
26 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 4/17/2023 in #java-help
Why does one error and not the other?
I am using Intellij, and it is completely fine with this code but says this does not work, with the error "method does not override method from it's superclass." Both of these methods are overriding equivalent methods from the class this one extends.
26 replies
JCHJava Community | Help. Code. Learn.
•Created by blockgoblin31 on 4/6/2023 in #java-help
What is returning false?
With this code, when I run the test method and case two runs, no matter what keys[i] and hide[i] are, it returns false. What is going wrong?(Not sure if it happens with the other cases, my current code sets list to only 0s and 2s)
8 replies