Florian
PPrisma
•Created by Florian on 3/21/2025 in #help-and-questions
Workflow for getting your DB schema into production when using separate databases
Let's say I use separate databases in development and production.
How do you push your schema changes into your production DB? Do you just run
push
or migrate
after pulling the latest code from GitHub?12 replies
PPrisma
•Created by Florian on 3/20/2025 in #help-and-questions
Prisma setup on VPS
Hey, is it possible to use Prisma when my Next.js app and Postgres DB run on a VPS? How does Prisma connect to the DB in this case?
5 replies
PPrisma
•Created by Florian on 3/7/2025 in #help-and-questions
Composing relation types
How do ya'll set up your relation types?
I currently have one big type that includes all relations:
But some in places I only use a subset of these values.
I assume I should split that up. But what then? Do you create combinations of types like
type ProjectWithTasksAndAttachments = ProjectWithTasks & ProjectWithAttachments
?
What's your approach?9 replies
PPrisma
•Created by Florian on 2/24/2025 in #help-and-questions
Custom user type vs database type
I usually use the Prisma generated types throughout my app. But in my current project, I'm getting into problems.
Example:
Since it's a multi-tenant app, a user can belong to multiple organizations:
In my code, I only fetch the membership data for the currently active organization, which causes me to access the organization info like this:
This doesn't look great. Should I make my own user type and map the DB data to it? Then the user could have a role field.
8 replies
PPrisma
•Created by Florian on 1/29/2025 in #help-and-questions
Tips for dividing multiple schema files
It's amazing that Prisma now supports multiple schema files.
But I have trouble splitting up my schemas and deciding what belongs together.
For example, would you put all of these Auth.js related schemas into one file? Or would you make a separate file for each of them?
5 replies
PPrisma
•Created by Florian on 12/22/2024 in #help-and-questions
Use foreign key as primary key
I've implemented multi-table inheritance following your guide: https://www.prisma.io/docs/orm/prisma-schema/data-model/table-inheritance#multi-table-inheritance-mti
My question: Is it possible to merge the foreign key and primary key on my child table? It's easier to query if the parent table (
User
) and child table (Admin
) have the same id. But duplicating the ID is not great because it introduces room for mistakes.
I tried it earlier and got an error.
5 replies
PPrisma
•Created by Florian on 6/29/2024 in #help-and-questions
How do you organize your Prisma.validator schemas?
I have a bunch of predefined
select
and include
schemas. Where do I put them in my project? I need some inspiration.
3 replies
PPrisma
•Created by Florian on 6/12/2024 in #help-and-questions
Tips on designing this recursive database model
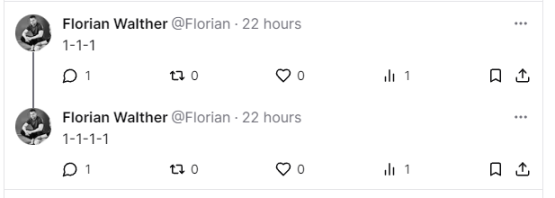
18 replies
PPrisma
•Created by Florian on 6/4/2024 in #help-and-questions
Type-safety for dates when returning DB entries as JSON
I used to use Prisma in server components where I don't need to do serialization.
Now I need to return DB entries from an API route handler in JSON form.
This means, the Date type of the Prisma model is not correct on the client anymore. JSONification turns the Date into a string.
What's the correct way to handle this on the client so I have type-safety?
11 replies
PPrisma
•Created by Florian on 5/24/2024 in #help-and-questions
No index found for fulltext search over relation (Planetscale)
I'm trying to find "favorited companions" combined with the ability to search through them.
As you can see in my code, I search inside a relation query. But I get an error that no index could be found.
Is this not possible with Planetscale?
Searching throug companions directly works. The search index on
name
+ description
exists. But I don't know what index I could add to the favorites model to make this work.
4 replies
PPrisma
•Created by Florian on 5/19/2024 in #help-and-questions
Prisma.validator with arguments
Is this the correct way to use Prisma validator (to reuse parts of a query) if the query depends on dynamic data?
2 replies
PPrisma
•Created by Florian on 5/14/2024 in #help-and-questions
Counting hashtags in all posts
I want to build a Twitter-link "trending" feature for which I need to find and count the hashtags inside user posts. What Prisma/Postgres feature would I use for this? Full-text search?
2 replies
PPrisma
•Created by Florian on 5/12/2024 in #help-and-questions
Additional processing after fetching data
I want to fetch posts from my database but additional scrape the opengraph info from the first link in the post. What would be a good abstraction or approach to do this for every request on this model?
2 replies
PPrisma
•Created by Florian on 5/11/2024 in #help-and-questions
Extra query into object (GetPayload)
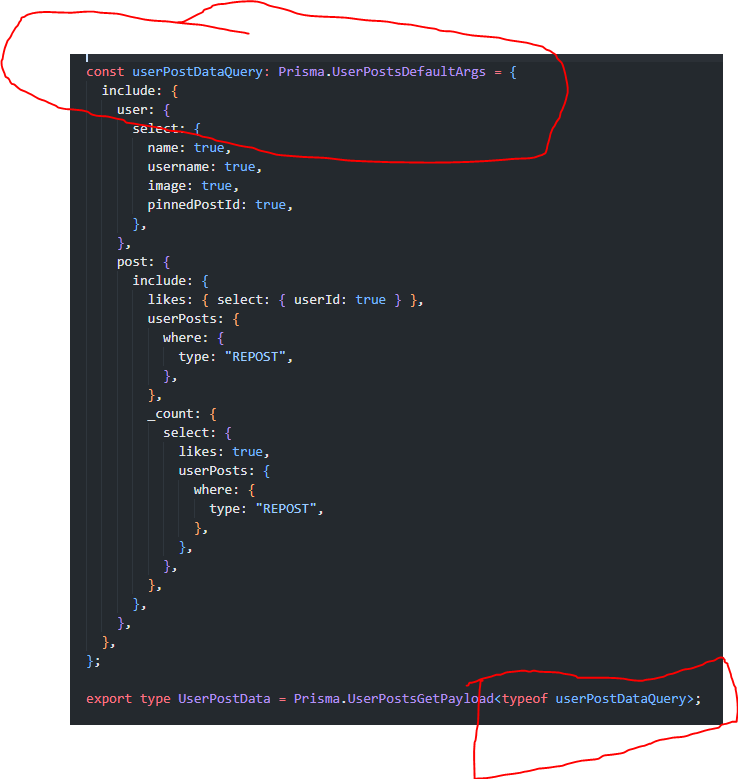
5 replies