Niels
PPrisma
•Created by Niels on 10/31/2024 in #help-and-questions
Way to run migrations & seeding using the PrismaClient
Hi, is it possible to run the migrations and seedings for Prisma at runtime using the PrismaClient instance?
Would prevent me from having to bundle all source files into my docker containers as well, since its all compiled down.
7 replies
PPrisma
•Created by Niels on 8/22/2024 in #help-and-questions
Prisma keeps generating same migrations
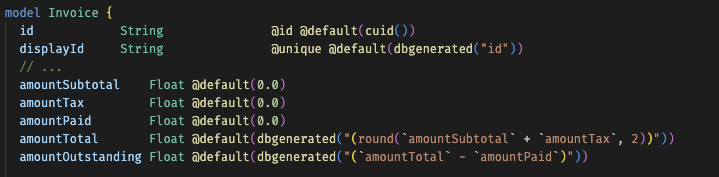
4 replies