Circus
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Circus on 4/26/2024 in #questions
VSCode Import Autocomplete Purgatory (Relative, absolute, and barrel paths)
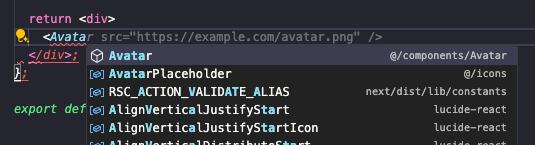
2 replies
TTCTheo's Typesafe Cult
•Created by Circus on 4/22/2024 in #questions
Appropriate Use of Modals - Attach to mapped components or pass modal logic down?
Is there considerable downside to attaching a modal to individual mapped components? Is it better/preferred to have a centralized Modal on the page and then pass down modal logic to the mapped components?
Example 1: Modal on each mapped component
Example 2: Centralized Modal
8 replies
Revalidate / invalidate in server components
Is there a solution for TRPC query invalidation (or revalidating), passing the actions from the client component where the action happens to server components? So far, I've only seen examples where you have to turn the respective server components into client components. Are there any better solutions?
3 replies
TTCTheo's Typesafe Cult
•Created by Circus on 3/26/2024 in #questions
T3, Intellisense, & Alias Path/Module
Using the latest T3 stack, I've been having issues getting Intellisense autocomplete to recognize any custom alias modules. It's not really an error, so no error message to give. Does anyone have any ideas? Screenshot for folder structure (src/app/_components/index for barrel importing)
2 replies
TTCTheo's Typesafe Cult
•Created by Circus on 3/21/2024 in #questions
Is it worth updating from the old T3 stack to new?
Hi, I was hoping to get some consensus on a decision about whether I should upgrade my application to the latest T3 stack and would love your input
Current stack
Next pages router, Clerk, Planetscale, Prisma, TRPC. Not a huge or complex app (my experience is mid-senior on frontend, early-mid on backend)
Current Issues
Planetscale's free tier has made
switching to a different database a nightmare. I've heard Supabase is nice but it doesn't play well with Prisma. There's a widening gap between new technologies that are only being set up for app router and I'm worried about the lifetime of pages router.
Trepidations
Clerk and app router notoriously don't work well together, and NextAuth is notoriously horrifying as well. I have very little users and data overall, so all the work needed to do the update.
Is it worth upgrading to the latest T3 stack? There seem to be tradeoffs going both ways, and right now it's very tempting to just spend $40/month at Planetscale to handle my whopping 100 rows of data even though my app makes no money just to not deal with the pain and frustration, but would love to get your input for a currently jaded developer
10 replies
TTCTheo's Typesafe Cult
•Created by Circus on 3/19/2024 in #questions
Migrating away from Planetscale (expiring hobby plan)
Due to Planetscale deprecating their hobby plan, I'm taking a very basic database of my hobby project and seeking to move it over. I've seen Supabase recommended, but the transfer of Planetscale's MySQL base to Supabase's Postgres is a nightmare for something that should be very simple.
For those of us that just want an easy migration, does anyone know of the most pain-free experience to migrate data?
12 replies
TTCTheo's Typesafe Cult
•Created by Circus on 3/1/2024 in #questions
Using getStaticProps with TRPC (Fetching data via mutate)
Hi, I'm trying to do a TRPC fetch that requires a mutation (not possible to do a query, GET request not allowed) just once on a page. It would make sense to have it in
getStaticProps
, but I can't get it to work properly. Either the ssg helpers aren't recognized as with other fetch routes or trying to retrieve the data in a hook in the component leads to infinite mutates. I've left small notes in the codes to show as much. What would you say is the optimal way to handle this?
2 replies
TTCTheo's Typesafe Cult
•Created by Circus on 2/27/2024 in #questions
TRPC with Google Places API (New)
Has anyone had any luck using TRPC with Google's Places API (New) that only has cURL examples? Here is my attempted router for nearby search using the example from Google's docs (https://developers.google.com/maps/documentation/places/web-service/nearby-search) that gets a generic 400 status. The cURL works, so there's something wrong with the router...or possibly the API 🙃
4 replies
TTCTheo's Typesafe Cult
•Created by Circus on 2/9/2024 in #questions
Using TRPC with Google's Place Details
Has anyone been able to properly set up a TRPC router with Google's Place Details API?
(https://developers.google.com/maps/documentation/places/web-service/place-details)
Doing the curl command from the link works fine, so it's something with the TRPC request (Status 400 HTTP error). I've also tried passing the parameters as headers in the
options
field to no avail
7 replies
TTCTheo's Typesafe Cult
•Created by Circus on 12/9/2023 in #questions
Editable text that doesn't change CSS between <text>, <p>, <div>, etc. and <input> tags (tailwind)
Hi, does anyone have a good example of how to have a textbox that, when in an editable state, doesn't change CSS properties so that it looks like you're typing right into the existing text? I've been having lots of issues with formatting the <input> classNames (via tailwind) to match the properties correctly
2 replies
TTCTheo's Typesafe Cult
•Created by Circus on 11/27/2023 in #questions
Clerk Webhooks with Prisma (Sync database, user model)
Hi, does anyone have a working example of how to sync user model via Prisma with Clerk? Clerk recommends using webhooks but the process has proven to be very tedious with little in the way of documentation.
I know you can instead be sneaky and use the afterAuth middleware instead, so I'll take those examples as well.
30 replies
TTCTheo's Typesafe Cult
•Created by Circus on 10/9/2023 in #questions
T3 - How to create a User record on Clerk signin (i.e. proper way of keeping track of user info)
Hello, is there a way to creat/upsert a User into a User schema when someone logs into your app via Clerk? I'm thinking of creating a User model with similar functionality of typical social media uses e.g. upvotes, likes, posts, etc.
I know that with Clerk, people have said you can omit having a User model since you can make queries from your other models (e.g. showing all likes with the desired userId, showing all posts with the userId, etc.), but if you want to show several rows of different databases (posts, likes, etc), wouldn't it make more sense to have a User database so you can just run one query? I've been able to create a function that makes the user, but haven't seen how to run this on signing in, so there's the tedious process of having the user click a button or something else
Open-ended question on what is best practice overall and how to best proceed with conventional T3 stack for this functionality- Prisma, Clerk, TRPC, Next
3 replies
TTCTheo's Typesafe Cult
•Created by Circus on 10/3/2023 in #questions
Shadcn components don't work out of the box?
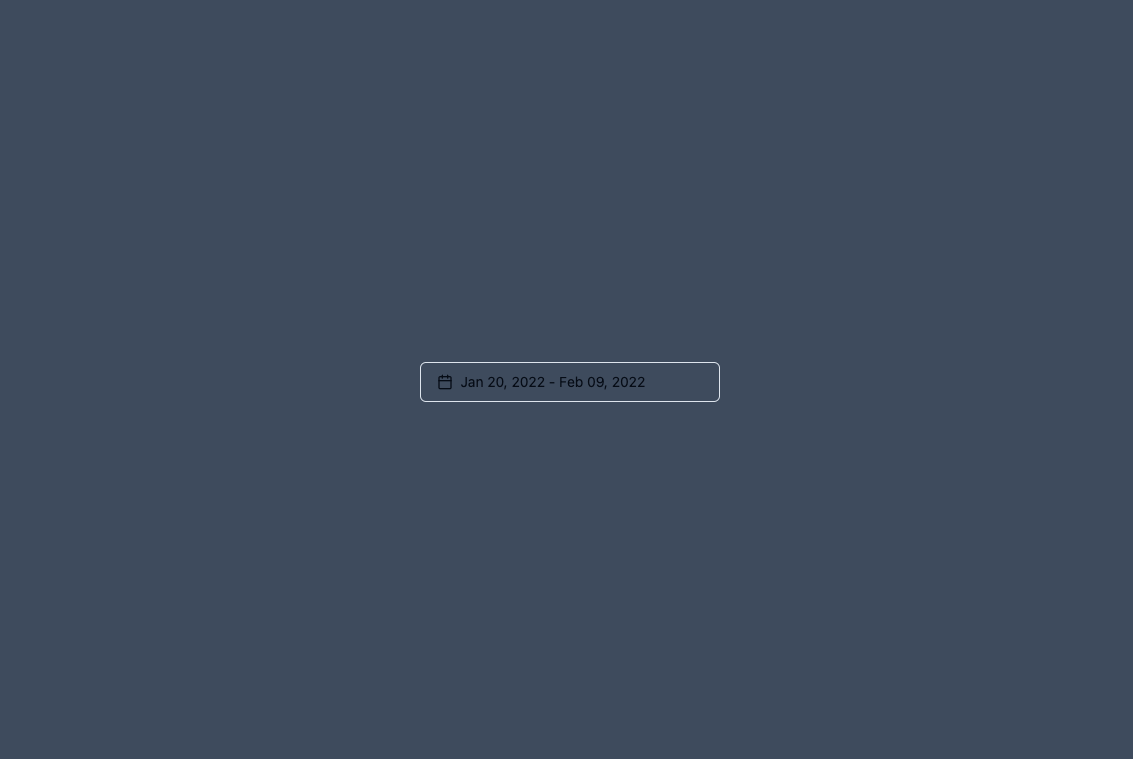
8 replies
TTCTheo's Typesafe Cult
•Created by Circus on 9/18/2023 in #questions
TRPC & Optimistic Update
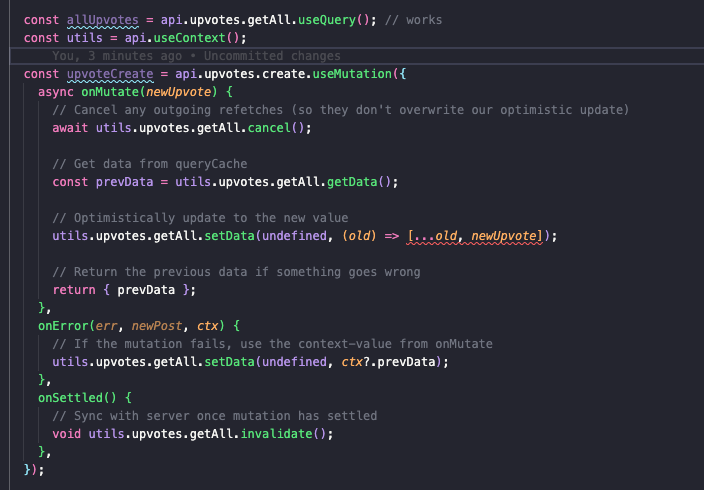
2 replies